帮我用Python搭建一个私链
时间: 2024-03-06 21:15:02 浏览: 133
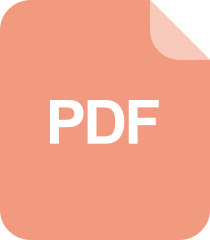
搭建以太坊私有链1
当您想要搭建一个私有区块链时,您可以使用Python编写自己的区块链代码。下面是一个简单的示例,演示如何使用Python创建一个基本的私有区块链:
```python
import hashlib
import datetime
class Block:
def __init__(self, index, timestamp, data, previous_hash):
self.index = index
self.timestamp = timestamp
self.data = data
self.previous_hash = previous_hash
self.hash = self.calculate_hash()
def calculate_hash(self):
sha = hashlib.sha256()
sha.update(str(self.index).encode('utf-8') +
str(self.timestamp).encode('utf-8') +
str(self.data).encode('utf-8') +
str(self.previous_hash).encode('utf-8'))
return sha.hexdigest()
class Blockchain:
def __init__(self):
self.chain = [self.create_genesis_block()]
def create_genesis_block(self):
return Block(0, datetime.datetime.now(), 'Genesis Block', '0')
def get_latest_block(self):
return self.chain[-1]
def add_block(self, new_block):
new_block.previous_hash = self.get_latest_block().hash
new_block.hash = new_block.calculate_hash()
self.chain.append(new_block)
# 创建区块链实例
my_blockchain = Blockchain()
# 添加新的区块
block1 = Block(1, datetime.datetime.now(), 'Block 1 Data', '')
my_blockchain.add_block(block1)
block2 = Block(2, datetime.datetime.now(), 'Block 2 Data', '')
my_blockchain.add_block(block2)
# 打印区块链
for block in my_blockchain.chain:
print("Block {}: {}".format(block.index, block.data))
print("Timestamp: {}".format(block.timestamp))
print("Previous Hash: {}".format(block.previous_hash))
print("Hash: {}\n".format(block.hash))
```
这是一个简单的示例,创建了一个包含创世块和两个区块的区块链。每个区块都包含索引、时间戳、数据、前一个区块的哈希值和当前区块的哈希值。在示例中,我们使用SHA-256哈希算法计算区块的哈希值。
您可以根据自己的需求扩展该代码,添加更多的功能,如交易、挖矿、共识算法等。请注意,这只是一个简单的示例,真实的区块链实现可能会更加复杂和庞大。
阅读全文
相关推荐
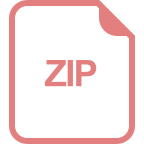
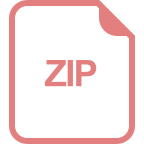
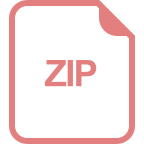
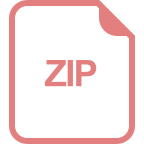
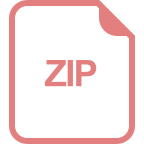
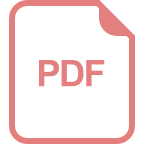
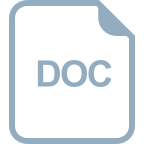
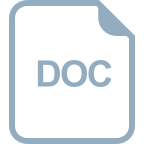
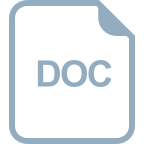
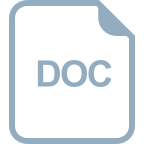
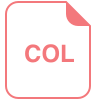
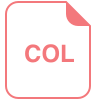
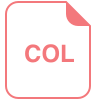
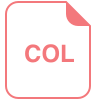
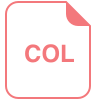
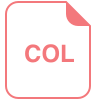