python绘图双纵坐标
时间: 2023-10-19 20:34:44 浏览: 41
要在Python中绘制双纵坐标的图表,可以使用Matplotlib库。下面是一种常见的方法:
1. 导入所需的库:
```python
import matplotlib.pyplot as plt
```
2. 创建图表和轴对象:
```python
fig, ax1 = plt.subplots()
```
3. 绘制第一个纵坐标轴的数据:
```python
ax1.plot(x1, y1, color='red', label='纵坐标1')
```
4. 设置第一个纵坐标轴的标签和图例:
```python
ax1.set_xlabel('横坐标')
ax1.set_ylabel('纵坐标1')
ax1.legend(loc='upper left')
```
5. 创建第二个纵坐标轴对象:
```python
ax2 = ax1.twinx()
```
6. 绘制第二个纵坐标轴的数据:
```python
ax2.plot(x2, y2, color='blue', label='纵坐标2')
```
7. 设置第二个纵坐标轴的标签和图例:
```python
ax2.set_ylabel('纵坐标2')
ax2.legend(loc='upper right')
```
8. 显示图表:
```python
plt.show()
```
这样就可以在同一个图表上绘制两个纵坐标轴的数据了。你可以根据自己的具体需求修改数据和设置。记得在代码中使用实际的数据和标签来替换`x1`、`y1`、`x2`、`y2`等变量。
相关问题
python绘图自定义纵坐标取值范围
在 Python 中,可以使用 Matplotlib 库来绘制图形并自定义纵坐标的取值范围。下面是一个简单的示例代码:
```python
import matplotlib.pyplot as plt
# 生成一些示例数据
x = [1, 2, 3, 4, 5]
y = [10, 20, 15, 25, 30]
# 创建图形对象和子图对象
fig, ax = plt.subplots()
# 绘制折线图
ax.plot(x, y)
# 设置纵坐标的取值范围
ax.set_ylim(0, 40) # 自定义取值范围为0到40
# 显示图形
plt.show()
```
在上面的示例中,我们使用 `ax.set_ylim()` 方法来设置纵坐标的取值范围,参数传入最小值和最大值。你可以根据自己的需求修改这两个参数来自定义纵坐标的取值范围。
python绘图设置纵坐标范围[0-1]
可以使用matplotlib库来绘制图形,并使用set_ylim()方法来设置纵坐标范围为[0,1]。
示例代码:
```python
import matplotlib.pyplot as plt
# 生成数据
x = [1, 2, 3, 4, 5]
y = [0.2, 0.4, 0.6, 0.8, 1]
# 绘制图形
plt.plot(x, y)
# 设置纵坐标范围为[0,1]
plt.ylim(0, 1)
# 显示图形
plt.show()
```
这段代码将生成一个简单的折线图,其中纵坐标范围为[0,1]。您可以根据需要修改数据和其他绘图属性。
相关推荐
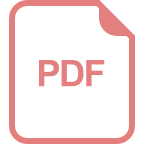
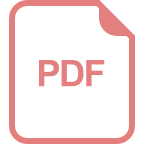
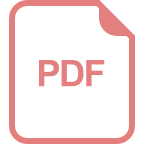












