for in和async await\
时间: 2023-08-14 22:14:16 浏览: 62
`for-in` 和 `async-await` 是两个不同的概念,分别用于不同的编程场景。
`for-in` 是一种用于遍历可迭代对象(如数组、字符串、集合等)的循环语句。它可以用来依次访问集合中的每个元素,并执行相应的操作。在每次迭代中,`for-in` 循环会将集合中的元素赋值给一个临时变量,然后执行指定的代码块。例如:
```python
for item in iterable:
# 执行操作
```
`async-await` 是一种用于处理异步操作的语法糖,通常与 `async` 函数一起使用。`async-await` 允许在异步操作完成之前暂停函数的执行,并在异步操作完成后继续执行。这样可以避免回调地狱,使代码更易读、更易编写。在 JavaScript 中,`async-await` 是基于 Promise 实现的。例如:
```javascript
async function fetchData() {
try {
const response = await fetch(url);
const data = await response.json();
// 执行操作
} catch (error) {
// 处理错误
}
}
```
总结一下:
- `for-in` 用于遍历集合中的元素,适用于同步操作。
- `async-await` 用于处理异步操作,适用于需要等待异步结果的情况。
相关问题
async await和回调
async/await是ES7的新增语法,它是一种异步编程的解决方案,可以让异步代码看起来像同步代码,从而避免回调地狱的问题。async用于声明一个异步函数,而await用于等待一个异步函数执行完成并返回结果。
下面是一个使用async/await解决回调地狱的例子:
```python
async def get_user_info(user_id):
user = await get_user(user_id)
friends = await get_friends(user_id)
friend_info_list = []
for friend in friends:
friend_info = await get_user_info(friend.id)
friend_info_list.append(friend_info)
return {
'user': user,
'friends': friend_info_list
}
```
在上面的例子中,我们定义了一个异步函数get_user_info,它会依次调用get_user、get_friends和get_user_info函数,并将它们的结果组合成一个字典返回。在调用这些异步函数时,我们使用了await关键字来等待它们的执行结果。
async await
Async/await is a feature in modern JavaScript that allows developers to write asynchronous code in a synchronous style. The async keyword is used to define a function that returns a promise, while the await keyword is used to wait for the resolution of a promise.
Async/await is a more readable and easier-to-understand way to write asynchronous code than using callbacks or promises. It allows developers to write code that looks like regular synchronous code, making it easier to reason about and debug.
Here's an example of using async/await to fetch data from an API:
```
async function fetchData() {
const response = await fetch('https://jsonplaceholder.typicode.com/todos/1');
const data = await response.json();
console.log(data);
}
fetchData();
```
In this example, the fetchData function is declared as async and returns a promise. Inside the function, we use the await keyword to wait for the fetch request to complete and the response to be returned. Once we have the response, we use another await keyword to wait for the response to be parsed as JSON. Finally, we log the data to the console.
Async/await is a powerful tool for writing asynchronous code and is widely used in modern JavaScript applications.
相关推荐
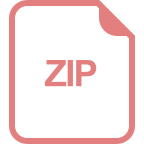
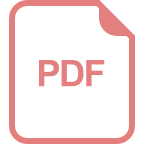
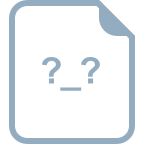
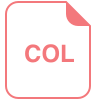
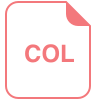
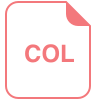
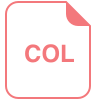
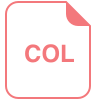







