给出一个pytorch中LSTM-Attention模型怎么搭建
时间: 2024-04-13 10:28:22 浏览: 143
要搭建一个基于PyTorch的LSTM-Attention模型,可以按照以下步骤进行:
首先,导入所需的库:
```python
import torch
import torch.nn as nn
```
然后,定义模型类:
```python
class LSTMAttentionModel(nn.Module):
def __init__(self, input_size, hidden_size, output_size):
super(LSTMAttentionModel, self).__init__()
self.hidden_size = hidden_size
# 定义LSTM层
self.lstm = nn.LSTM(input_size, hidden_size, batch_first=True)
# 定义Attention层
self.attention = nn.Linear(hidden_size, 1)
# 定义输出层
self.fc = nn.Linear(hidden_size, output_size)
def forward(self, input):
# LSTM层前向传播
output, _ = self.lstm(input)
# Attention层前向传播
attention_weights = torch.softmax(self.attention(output), dim=1)
attention_output = torch.sum(output * attention_weights, dim=1)
# 输出层前向传播
output = self.fc(attention_output)
return output
```
在上述代码中,我们定义了一个名为`LSTMAttentionModel`的模型类。在初始化函数中,我们定义了LSTM层、Attention层和输出层。在前向传播函数中,我们首先通过LSTM层获取输出,然后使用Attention层计算注意力权重,并将注意力权重与LSTM输出相乘得到注意力输出。最后,我们将注意力输出传入输出层,得到最终的模型输出。
注意:上述代码仅为搭建LSTM-Attention模型的基本框架,具体的输入维度、隐藏层维度和输出维度等需要根据实际任务进行调整。同时,还可以根据需要添加其他层或修改模型结构。
阅读全文
相关推荐
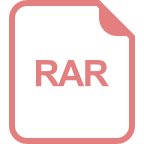
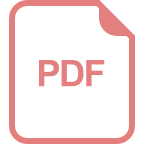


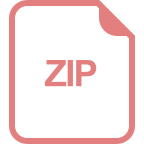
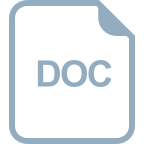
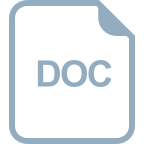
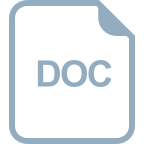
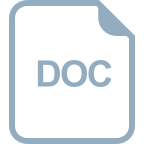
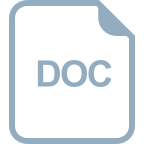
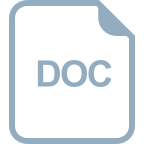





