pytorch 代码实现bilstm-self-attention
时间: 2023-08-28 16:06:16 浏览: 167
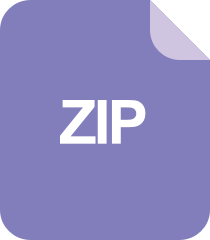
bi-lstm-crf:BI-LSTM-CRF模型的PyTorch实现
好的,这是一个比较常见的模型结构。以下是实现代码:
首先是导入需要的包:
```python
import torch
import torch.nn as nn
import torch.nn.functional as F
```
接下来是模型的主体结构:
```python
class BiLSTM_SelfAttention(nn.Module):
def __init__(self, num_tokens, embedding_size, hidden_size, num_layers):
super(BiLSTM_SelfAttention, self).__init__()
self.embedding = nn.Embedding(num_tokens, embedding_size)
self.lstm = nn.LSTM(embedding_size, hidden_size, num_layers=num_layers,
batch_first=True, bidirectional=True)
self.self_attn = nn.MultiheadAttention(embed_dim=hidden_size * 2, num_heads=8)
self.fc = nn.Linear(hidden_size * 2, 1)
def forward(self, x):
embedded = self.embedding(x)
bilstm_output, _ = self.lstm(embedded)
attn_output, _ = self.self_attn(bilstm_output.transpose(0, 1), bilstm_output.transpose(0, 1), bilstm_output.transpose(0, 1))
fc_output = self.fc(attn_output.squeeze(0))
return fc_output
```
代码中,模型使用了一个 Embedding 层将输入的 tokens 转成 embedding ,使用了一个 BiLSTM 层将句子做一个 Bidirectional 的处理,接下来是 self-attention 进行得分计算,最后通过一个线性层转换为预测得分输出。注意 self-attention 层的输入需要将 BiLSTM 输出进行 transpose 处理,使得每个时刻的 hidden state 形状为 batch_size * hidden_size * num_directions。做完 self-attention 后再将表示转置回来即可。
这样就完成了一个 pytorch 实现的 bilstm-self-attention 模型。希望我的回答对你有帮助!
阅读全文
相关推荐
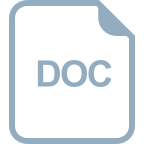
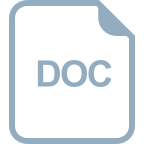







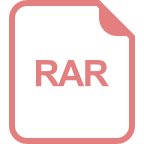
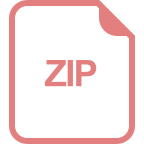
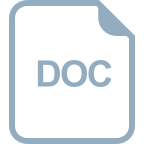
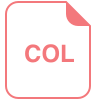
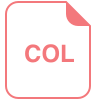



