paython中,匹配一个密码8-12位数字字母下划线构成
时间: 2024-12-19 10:22:20 浏览: 7
在Python中,你可以使用正则表达式(re)模块来验证一个字符串是否满足特定的格式,比如8到12位的数字、字母和下划线。下面是一个简单的例子:
```python
import re
def is_valid_password(password):
pattern = r"^(?=.*\d)(?=.*[a-zA-Z])(?=.*_)[0-9a-zA-Z_]{8,12}$"
if re.match(pattern, password):
return True
else:
return False
# 测试函数
print(is_valid_password("password123_")) # 输出:True 或者 False,取决于输入的密码是否符合条件
```
这个正则表达式的解释:
- `^` 表示字符串的开始
- `(?=.*\d)` 匹配至少有一个数字
- `(?=.*[a-zA-Z])` 匹配至少有一个字母(大小写都算)
- `(?=.*_)` 匹配至少有一个下划线 _
- `[0-9a-zA-Z_]` 匹配数字、字母和下划线
- `{8,12}` 指定最少8位,最多12位
- `$` 表示字符串的结束
相关问题
paython:凯撒密码
以下是 Python 代码,可以实现凯撒密码的加密和解密:
```python
def caesar_cipher(text, shift, mode):
result = ""
if mode == "decode":
shift = 0 - shift
for char in text:
if char.isalpha():
ascii_code = ord(char) + shift
if char.isupper():
if ascii_code > ord("Z"):
ascii_code -= 26
elif ascii_code < ord("A"):
ascii_code += 26
elif char.islower():
if ascii_code > ord("z"):
ascii_code -= 26
elif ascii_code < ord("a"):
ascii_code += 26
result += chr(ascii_code)
else:
result += char
return result
# 测试
text = "Hello, World!"
shift = 3
encrypted_text = caesar_cipher(text, shift, "encode")
print("加密后的文本:", encrypted_text)
decrypted_text = caesar_cipher(encrypted_text, shift, "decode")
print("解密后的文本:", decrypted_text)
```
输出结果为:
```
加密后的文本: Khoor, Zruog!
解密后的文本: Hello, World!
```
其中,`text` 是原始文本,`shift` 是偏移量,`mode` 可以是 "encode" 或 "decode",分别表示加密和解密。函数返回加密或解密后的文本。
paython如何生成一个随机算式
要生成一个随机的算式,可以使用Python的random库。以下是一个简单的示例代码:
```python
import random
def generate_equation():
# 生成随机数字和运算符
num1 = random.randint(1, 10)
num2 = random.randint(1, 10)
operator = random.choice(['+', '-', '*', '/'])
# 根据运算符生成算式
if operator == '+':
equation = str(num1) + ' + ' + str(num2)
elif operator == '-':
equation = str(num1) + ' - ' + str(num2)
elif operator == '*':
equation = str(num1) + ' * ' + str(num2)
elif operator == '/':
equation = str(num1) + ' / ' + str(num2)
return equation
# 生成一个随机算式并打印
equation = generate_equation()
print(equation)
```
这段代码首先导入random库,然后定义了一个函数`generate_equation()`来生成随机算式。函数内部使用`random.randint()`生成两个随机数,其中参数1和参数10表示生成的随机数的范围是1到10(包括1和10)。`random.choice()`则用来随机选择运算符。根据运算符的不同,函数会返回相应的算式字符串。
最后,通过调用函数并打印返回的算式字符串,即可生成并显示一个随机算式。
阅读全文
相关推荐
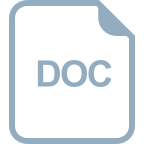
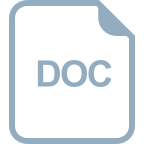
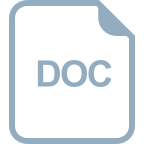
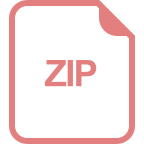
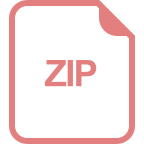
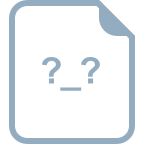










