std::cin输入vector数组
时间: 2023-08-21 14:06:00 浏览: 219
您可以使用以下代码从标准输入流(std::cin)读取一个 vector 数组:
```cpp
#include <iostream>
#include <vector>
int main() {
std::vector<int> nums;
int num;
while (std::cin >> num) {
nums.push_back(num);
}
// 打印 vector 数组的元素
for (int n : nums) {
std::cout << n << " ";
}
std::cout << std::endl;
return 0;
}
```
这段代码会持续从标准输入流中读取整数,直到遇到非整数输入或者文件末尾。然后将读取到的整数添加到 vector 数组中,并最终打印出数组中的元素。
希望这能帮到您!如果您有任何其他问题,请随时提问。
相关问题
#include <bits/stdc++.h> using i64 = long long; void solve() { int n, k; std::cin >> n >> k; std::vector<int> a(n); for (int i = 0; i < n; i++) { std::cin >> a[i]; } std::sort(a.begin(), a.end()); i64 ans = -1E18; std::vector<i64> s(n + 1); for (int i = 0; i < n; i++) { s[i + 1] = s[i] + a[i]; } for (int i = 0; i <= k; i++) { ans = std::max(ans, s[n - (k - i)] - s[2 * i]); } std::cout << ans << "\n"; } int main() { std::ios::sync_with_stdio(false); std::cin.tie(nullptr); int t; std::cin >> t; while (t--) { solve(); } return 0; }
这段代码实现了一个题目的解法,题目描述和输入输出格式没有提供,但是可以猜测是一个数组分段求和的问题。具体解法如下:
1. 读入数据,包括数组长度 n 和分段数 k,以及数组 a。
2. 对数组 a 进行排序。
3. 计算前缀和数组 s,其中 s[i] 表示 a[0] 到 a[i-1] 的和。
4. 枚举区间 [i, n-(k-j)],其中 i 表示前 j 段的末尾位置(即前 j 段的元素个数为 2*j),n-(k-j) 表示后面 k-j 段的开头位置(即后面 k-j 段的元素个数为 n-(k-j)-i),计算该区间的和。
5. 在所有区间和中找到最大值,输出该值。
代码的时间复杂度为 O(t*n*logn),其中 t 是测试数据组数,n 是数组长度,logn 是排序的时间复杂度。
分析以下代码:#include <iostream>#include <string>#include <vector>std::string convertToZigzag(int numRows, const std::string& str) { if (numRows == 1 || str.length() <= numRows) { return str; } std::vector<std::string> rows(numRows); int currentRow = 0; bool goingDown = false; for (size_t i = 0; i < str.length(); i++) { rows[currentRow] += str[i]; if (currentRow == 0 || currentRow == numRows - 1) { goingDown = !goingDown; } currentRow += goingDown ? 1 : -1; } std::string result; for (size_t i = 0; i < rows.size(); i++) { result += rows[i]; } return result;}int main() { int numRows; std::string str; std::cin >> numRows; std::cin.ignore(); // 忽略换行符 std::getline(std::cin, str); std::string zigzag = convertToZigzag(numRows, str); std::cout << zigzag << std::endl; return 0;}
这段代码实现了将一个字符串按照“之”字形排列的功能。具体实现是先创建一个 numRows 个字符串的数组 rows,然后按照“之”字形将输入的字符串 str 中的字符依次插入到 rows 数组中,最后将 rows 数组中的字符串按照顺序合并成一个字符串作为输出。
具体实现过程中,使用了一个布尔变量 goingDown 来控制“之”字形的方向,初始为 false,表示从上向下,当当前行为第一行或最后一行时,将其取反,表示需要改变方向。
此外,为了读入包含空格的字符串,使用了 std::getline 函数,而在其之前使用 std::cin.ignore() 忽略了输入流中的换行符。
阅读全文
相关推荐
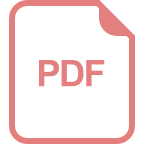
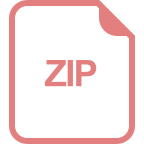
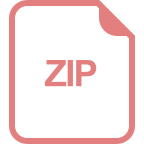




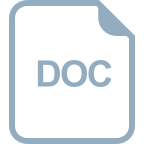
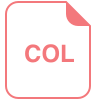
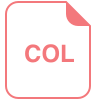






