c++输入一棵树的n-1条边,从节点1提起整棵树以后,求每个节点的深度。
时间: 2024-09-16 17:05:23 浏览: 54
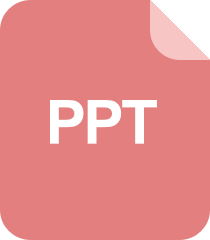
森林的遍历-C++树与森林
在 C++ 中,为了计算给定树中每个节点的深度,你需要遍历这棵未完全构建的树并使用递归或者其他数据结构(如层次遍历或广度优先搜索)。以下是基本步骤:
1. **数据结构**:首先,需要创建一个表示节点的数据结构,包含节点值、父节点引用以及子节点列表。对于有向图,可以使用邻接表存储边。
```cpp
struct TreeNode {
int val;
TreeNode* parent; // 父节点指针
vector<TreeNode*> children; // 子节点列表
};
```
2. **输入**:使用`std::vector`或其他容器来接收输入的n-1条边,每条边可以表示两个节点间的连接。例如,可以采用数组或二维数组的形式:
```cpp
std::vector<std::pair<int, int>> edges;
```
3. **构造树**:根据输入的边来建立树结构。假设我们从节点1开始:
```cpp
TreeNode* root = new TreeNode(1);
for (auto edge : edges) {
int node1 = edge.first, node2 = edge.second;
if (!root->children[node1 - 1]) { // 避免重复添加相同的子节点
root->children[node1 - 1] = new TreeNode(node2);
root->children[node2 - 1]->parent = root; // 设置连接关系
}
}
```
4. **计算深度**:可以递归地计算每个节点的深度。这里使用递归函数`depth`:
```cpp
int depth(TreeNode* node, int currentDepth = 0) {
if (node == nullptr) return currentDepth;
return std::max({currentDepth, depth(node->parent, currentDepth + 1)});
}
```
5. **结果输出**:最后,对根节点调用`depth(root)`得到整棵树的最大深度,然后遍历每个节点并打印其深度:
```cpp
for (const auto& child : root->children) {
cout << "Node " << child->val << " has a depth of " << depth(child) << endl;
}
```
阅读全文
相关推荐
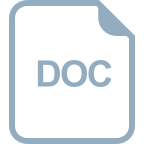
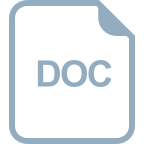










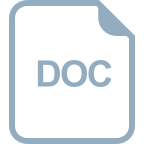
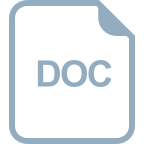
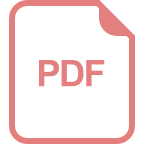
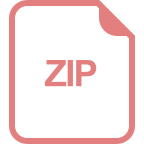
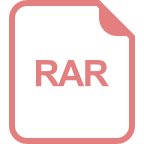
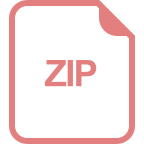