c++实现Image.fromarray
时间: 2024-06-12 20:10:21 浏览: 7
在C++中,可以使用OpenCV库来实现类似于Python中的Image.fromarray的功能。具体实现步骤如下:
1. 首先需要将数组转换为Mat对象,可以使用OpenCV的Mat构造函数来实现:
```
cv::Mat mat(rows, cols, CV_8UC3, data);
```
其中,rows和cols分别为数组的行数和列数,CV_8UC3表示每个像素由3个8位无符号整数表示,data为数组指针。
2. 然后可以使用imwrite函数将Mat对象保存为图像文件:
```
cv::imwrite("image.jpg", mat);
```
完整代码示例:
```
#include <opencv2/opencv.hpp>
int main() {
int rows = 100;
int cols = 100;
unsigned char data[rows * cols * 3];
// fill data with image data
cv::Mat mat(rows, cols, CV_8UC3, data);
cv::imwrite("image.jpg", mat);
return 0;
}
```
相关问题
class Camera(): def init(self, top): self.top = top self.h = int(data_demo.window_height // 1.5) self.w = int(data_demo.window_width // 1.74) self.canvas2 = Canvas(self.top, bg='LightSkyBlue', width=self.w, height=self.h, highlightthickness=2, highlightbackground='Black') self.canvas2.place(relx=0.0, rely=0.032) self.label = tk.Label(self.canvas2, text='摄像头显示区!', font=("黑体", 25), width=15, height=1) self.label.place(relx=0.32, rely=0.50, anchor='nw') self.queue = Queue() # 创建一个队列 def Firing_run(self, button_id): self.bool = True self.label.destroy() self.cap = cv2.VideoCapture(0) t = threading.Thread(target=self.windows_display) t.start() button_id.config(text='关闭摄像头', command=lambda: self.Cease_stop(button_id)) def Cease_stop(self, button_id): self.bool = False button_id.config(text='打开摄像头', command=lambda: self.Firing_run(button_id)) def windows_display(self): if self.bool: ref, frame = self.cap.read() if ref: image = cv2.cvtColor(frame, cv2.COLOR_BGR2RGB) image1 = Image.fromarray(image) image2 = image1.resize((self.w, self.h), Image.ANTIALIAS) photo = ImageTk.PhotoImage(image=image2) self.canvas2.create_image(0, 0, image=photo, anchor=tk.NW) self.canvas2.update() self.canvas2.after(15, self.windows_display())怎么优化
可以考虑以下几种优化方法:
1. 优化图像处理速度:可以采用一些优化的图像处理算法或者使用C++等高性能语言编写图像处理的部分,提高图像处理速度。
2. 减少线程间的通信:使用队列可以避免多个线程直接进行通信,但是每次从队列中读取数据也会有一定的开销,可以考虑使用共享内存等更高效的通信方式。
3. 优化界面显示:界面显示的部分可以使用异步更新的方式,避免界面卡顿或者闪烁的问题。
4. 合理利用硬件资源:可以考虑使用GPU等硬件加速技术来加快图像处理的速度,同时也可以考虑使用多个摄像头或者多个线程来进行并行处理。
如何实现 python调用C++ 函数 返回cv::mat
要实现 Python 调用 C++ 函数并返回 cv::Mat,可以使用以下步骤:
1. 在 C++ 中编写一个函数,该函数接受输入参数并返回 cv::Mat 类型的值。例如:
```c++
#include <opencv2/opencv.hpp>
cv::Mat myFunction(cv::Mat inputImage) {
// 这里可以进行一些图像处理操作
cv::Mat outputImage = inputImage.clone(); // 这里只是简单地将输入图像复制到输出图像中
return outputImage;
}
```
2. 将 C++ 函数编译为动态链接库(DLL)或共享对象(SO)文件,以便能够从 Python 中调用该函数。
3. 在 Python 中使用 ctypes 模块加载 C++ 动态链接库或共享对象,并使用 ctypes 将输入参数传递给 C++ 函数,并将返回值转换为 Python 中的 numpy 数组或 PIL 图像等格式。例如:
```python
import numpy as np
import ctypes
import cv2
# 加载 C++ 动态链接库或共享对象
my_lib = ctypes.cdll.LoadLibrary('./my_lib.so')
# 定义 C++ 函数的输入和输出类型
my_lib.myFunction.argtypes = [ctypes.c_void_p]
my_lib.myFunction.restype = ctypes.c_void_p
# 加载输入图像并将其转换为 cv::Mat 类型
input_image = cv2.imread('input.jpg')
input_mat = cv2.cvtColor(input_image, cv2.COLOR_BGR2RGB)
input_mat_ptr = ctypes.c_void_p(input_mat.ctypes.data)
# 调用 C++ 函数并将返回值转换为 numpy 数组
output_mat_ptr = my_lib.myFunction(input_mat_ptr)
output_mat = np.ctypeslib.as_array(output_mat_ptr, shape=input_mat.shape)
# 将 cv::Mat 转换为 PIL 图像
output_image = cv2.cvtColor(output_mat, cv2.COLOR_RGB2BGR)
pil_image = Image.fromarray(output_image)
pil_image.show()
```
以上就是实现 Python 调用 C++ 函数并返回 cv::Mat 的一般方法。注意,在使用 ctypes 模块进行转换时,需要确保传递给 C++ 函数的指针和返回的指针类型正确,并且需要进行适当的内存管理以避免内存泄漏。
相关推荐
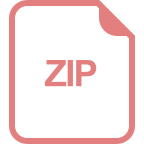
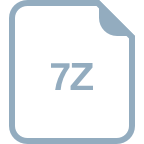
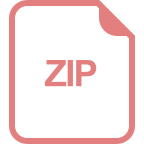












