用c语言写一个程序,要求倒计时20秒,同时还可以输入文字并检测是否符合要求
时间: 2024-09-24 20:07:02 浏览: 33
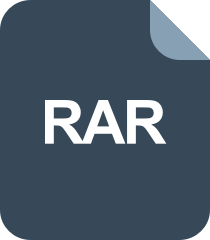
daojishi.rar_倒计时程序_单片机倒计时
你可以使用C语言编写一个简单的控制台程序,结合`time.h`库实现倒计时,并使用标准输入流`stdin`接收用户输入,检查字符串是否符合特定要求。下面是一个简单的示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
// 检查输入的字符串是否全部由数字组成
int is_number(const char *str) {
while (*str != '\0') {
if (!isdigit(*str)) {
return 0;
}
str++;
}
return 1;
}
void countdown(int seconds) {
for (int i = seconds; i > 0; i--) {
printf("Seconds remaining: %d\n", i);
sleep(1); // 使用sleep暂停1秒,实际环境需要替换为更精确的延时方法
}
printf("\nCountdown finished.\n");
}
int main() {
int input_seconds;
printf("Enter the number of seconds for countdown (0 to exit): ");
scanf("%d", &input_seconds);
if (input_seconds <= 0) {
printf("Exiting program.\n");
return 0;
}
countdown(input_seconds);
char user_input[100];
printf("Enter a string to check (numbers only accepted): ");
fgets(user_input, sizeof(user_input), stdin);
user_input[strcspn(user_input, "\n")] = '\0'; // Remove newline at the end
if (is_number(user_input)) {
printf("Input is valid (only numbers).\n");
} else {
printf("Input is not valid (contains non-number characters).\n");
}
return 0;
}
```
在这个程序中,我们首先定义了一个函数`is_number`来检查输入的字符串是否全为数字。然后在`main`函数中,获取用户的倒计时时间,如果小于等于0则退出。接着倒数并显示剩余秒数,最后读取用户输入并验证其是否只包含数字。
阅读全文
相关推荐
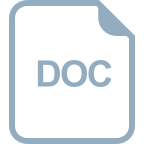
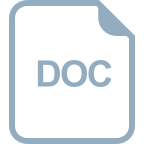
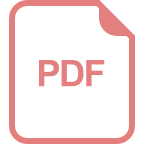
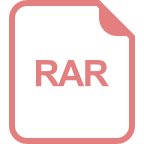
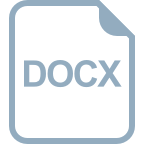
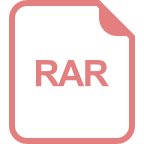
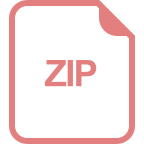
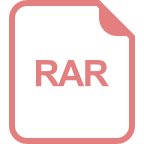
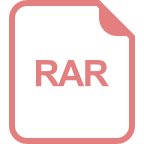
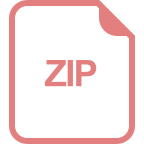
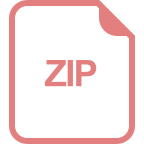
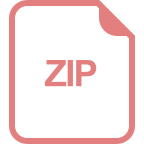
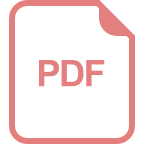
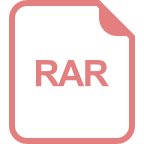
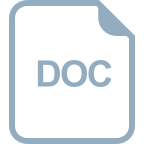