用java编译定义两个线程,student 和 teacher,其中 student 准备睡一个小时后开始上课,teacher 在输出 3 句“上课”后,吵醒休眠的线程 student。
时间: 2024-11-06 22:19:01 浏览: 44
在Java中,你可以通过创建`Thread`对象、实现`Runnable`接口或者使用`Callable`接口并结合`FutureTask`来定义和控制线程的行为。这里我们展示一个使用`Runnable`接口的例子:
首先,定义一个实现了`Runnable`接口的`Teacher`类,它代表教师角色:
```java
public class Teacher implements Runnable {
private Thread teacherThread;
private int count;
public Teacher() {
this.teacherThread = new Thread(this);
}
@Override
public void run() {
while (count < 3) {
System.out.println("上课");
try {
Thread.sleep(1000); // 模拟讲一节课的时间
} catch (InterruptedException e) {
e.printStackTrace();
}
count++;
}
// 打印最后一条信息并唤醒学生
System.out.println("叫醒学生,课程结束");
Student student = new Student();
student.notify(); // 使用notify()唤醒Student线程
}
// 启动教师线程
public void startClass() {
teacherThread.start();
}
}
```
接着,定义一个`Student`类,它代表学生角色,并使用`sleep()`和`notify()`来模拟睡眠和被唤醒的状态:
```java
class Student {
private boolean asleep = true; // 学生初始状态为睡觉
synchronized void sleep() {
while (asleep) {
try {
wait(); // 睡觉,等待老师唤醒
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
synchronized void wakeUp() {
asleep = false;
notify(); // 当被唤醒时,通知其他等待的学生
}
}
```
在主程序中,创建并启动教师线程:
```java
public static void main(String[] args) {
Teacher teacher = new Teacher();
teacher.startClass();
}
```
在这个例子中,教师线程先打印三次"上课",然后唤醒正在睡觉的学生。当`Teacher`类的`run()`方法中调用`wait()`时,学生的线程会进入睡眠状态,直到收到`notify()`。学生线程会在被唤醒后继续执行。
阅读全文
相关推荐





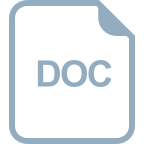












