dfs和bfs python
时间: 2024-11-20 19:43:27 浏览: 5
DFS (深度优先搜索) 和 BFS (广度优先搜索) 都是图遍历算法,常用于寻找图中的路径、节点连通性等。在 Python 中,可以利用内置的数据结构如列表或队列以及递归或迭代的方式实现。
**DFS** (深度优先搜索):
1. 使用递归实现简单,从根节点开始,尽可能深地访问分支。
2. 通常使用 stack (栈) 数据结构辅助,因为访问的是“深度”方向。
3. 示例代码(Python):
```python
def dfs(graph, node, visited=None):
if visited is None:
visited = set()
visited.add(node)
print(node)
for neighbor in graph[node]:
if neighbor not in visited:
dfs(graph, neighbor, visited)
```
**BFS** (广度优先搜索):
1. 运行过程更像一层层探索,先访问所有邻居再深入一层。
2. 通常使用 queue (队列) 数据结构,保证总是访问离起点最近的节点。
3. 示例代码(Python):
```python
from collections import deque
def bfs(graph, start):
visited = set()
queue = deque([start])
while queue:
vertex = queue.popleft()
if vertex not in visited:
visited.add(vertex)
print(vertex)
queue.extend(graph[vertex] - visited)
```
阅读全文
相关推荐
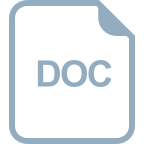
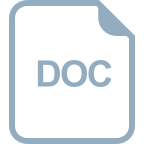
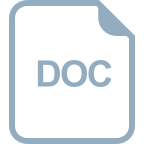

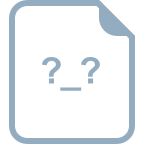






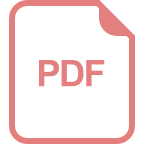
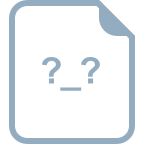
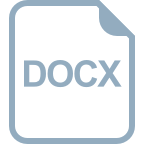
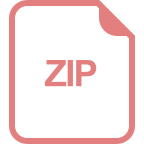
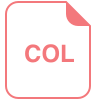


