(1) 用三种遍历算法遍历该二叉树,给出对应的输出结果。 (2) 写一个函数查找二叉树中的节点,根据其是否属于该树,输出true或者false。 (3) 统计该树的满节点、叶子节点、总节点数。 生成C代码
时间: 2024-12-14 12:17:52 浏览: 5
(1)对于这个二叉树,我们可以使用前序遍历(Preorder)、中序遍历(Inorder)和后序遍历(Postorder)三种方式进行遍历:
**前序遍历(根-左-右):**
- 输出:100 45 4 12 8 32 67 90 87
**中序遍历(左-根-右):**
- 输出:4 12 8 32 45 100 87 67 90
**后序遍历(左-右-根):**
- 输出:4 8 12 32 87 90 67 45 100
(2)这里是一个C函数,用于查找二叉树中的特定节点,如果找到则返回`true`,未找到则返回`false`:
```c
int find_node(Node* root, int target) {
if (root == NULL) {
return false; // 节点不存在于树中
} else if (root->value == target) {
return true; // 找到了目标节点
} else {
return root->value < target ? find_node(root->right, target) : find_node(root->left, target); // 递归查找
}
}
```
你可以用这个函数来查找到32这个节点,例如:`find_node(root, 32)`。
(3)统计树的满节点(完全填满左右子树的节点)、叶子节点(没有子节点的节点)和总节点数:
```c
int count_full_nodes(Node* root) {
if (root == NULL) {
return 0;
} else if (root->left == NULL && root->right == NULL) {
return 1;
} else {
return count_full_nodes(root->left) + count_full_nodes(root->right);
}
}
int count_leaf_nodes(Node* root) {
if (root == NULL) {
return 0;
} else if (root->left == NULL && root->right == NULL) {
return 1;
} else {
return 0; // 非叶节点,不需要计算
}
}
int count_total_nodes(Node* root) {
return count_full_nodes(root) + count_leaf_nodes(root) + 1; // 加1是因为根节点也计入总数
}
int main() {
Node* root = ...; // 根据实际二叉树构建
int full_nodes = count_full_nodes(root);
int leaf_nodes = count_leaf_nodes(root);
int total_nodes = count_total_nodes(root);
printf("Full nodes: %d, Leaf nodes: %d, Total nodes: %d\n", full_nodes, leaf_nodes, total_nodes);
return 0;
}
```
阅读全文
相关推荐
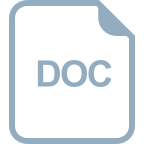
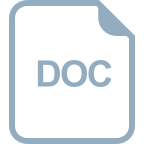
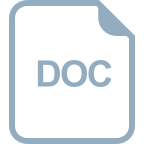


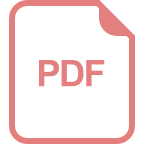
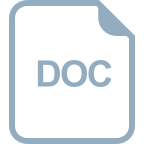
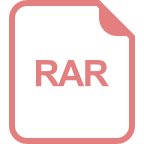
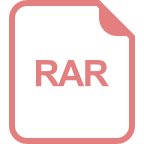
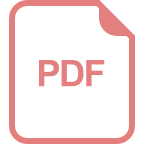
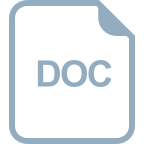
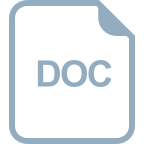
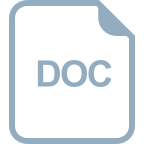





