Java请生成多项式A=5+9x+11x^6+14x^11-21x^18+ 18x^18 和B=8x+12x^3+2x^6-14x^11+12X^15,并输 出A+B的结果
时间: 2024-09-26 17:11:22 浏览: 28
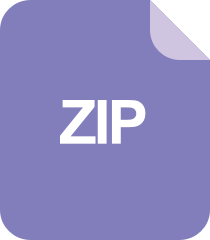
CRC16检验码生成+二维码生成
在Java中,我们可以创建一个`Polynomial`类来表示多项式,并包含加法操作的方法。首先,定义一个类结构:
```java
public class Polynomial {
private List<Integer> coefficients; // 用于存储各项系数
public Polynomial() {
this.coefficients = new ArrayList<>();
}
// 添加项的方法
public void addTerm(int exponent, int coefficient) {
coefficients.add(new Pair(exponent, coefficient));
}
// 加法方法
public Polynomial add(Polynomial other) {
Polynomial result = new Polynomial();
for (int i = 0; i < Math.max(coefficients.size(), other.coefficients.size()); i++) {
int thisCoeff = i < coefficients.size() ? coefficients.get(i).getSecond() : 0;
int otherCoeff = i < other.coefficients.size() ? other.coefficients.get(i).getSecond() : 0;
result.addTerm(i, thisCoeff + otherCoeff);
}
return result;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
for (int i = 0; i < coefficients.size(); i++) {
if (i > 0) {
sb.append(" + ");
}
int exponent = coefficients.get(i).getFirst();
if (exponent == 0) {
sb.append(coefficients.get(i).getSecond());
} else {
sb.append(coefficients.get(i).getSecond()).append("x^").append(exponent);
}
}
return sb.toString().trim();
}
// 其他辅助类 Pair 可能需要定义
static class Pair {
int first;
int second;
Pair(int first, int second) {
this.first = first;
this.second = second;
}
}
}
```
然后你可以按照多项式的定义生成并计算A和B,最后将它们相加:
```java
public static void main(String[] args) {
Polynomial A = new Polynomial();
A.addTerm(0, 5); // 5
A.addTerm(1, 9); // 9x
A.addTerm(6, 11); // 11x^6
A.addTerm(11, 14); // 14x^11
A.addTerm(18, -21); // -21x^18
Polynomial B = new Polynomial();
B.addTerm(1, 8); // 8x
B.addTerm(3, 12); // 12x^3
B.addTerm(6, 2); // 2x^6
B.addTerm(11, -14); // -14x^11
B.addTerm(15, 12); // 12x^15
Polynomial sum = A.add(B);
System.out.println("A + B = " + sum);
}
```
运行上面的代码,你会得到A和B相加后的结果。
阅读全文
相关推荐
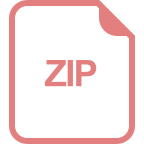
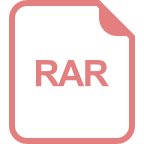





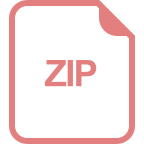
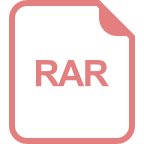
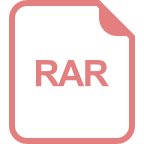
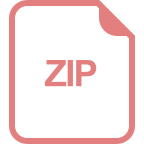
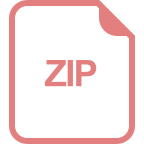
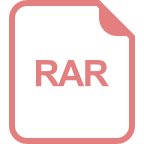
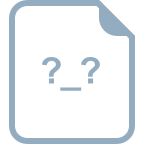
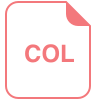
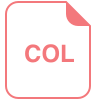
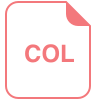

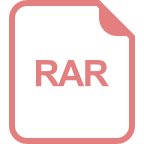