编写Java代码实现用在有限域GF(2^8)下求多项式乘法和求多项式逆元
时间: 2024-12-25 18:30:56 浏览: 11
在Java中实现有限域GF(2^8)下的多项式乘法(Polynomial Multiplication)和求逆元(Division or Inverse),我们需要利用二进制扩展的方法以及数学上的模运算。这里是一个简单的示例,我们将使用类来表示多项式和有限域元素。
```java
import java.math.BigInteger;
class GF28 {
static final BigInteger MODULO = BigInteger.valueOf(1 << 8); // 2^8
private BigInteger coefficients[];
public GF28(BigInteger[] coeffs) {
this.coefficients = new BigInteger[coeffs.length];
for (int i = 0; i < coeffs.length; i++) {
if (coeffs[i] != null) {
coefficients[i] = coeffs[i].mod(MODULO);
}
}
}
// 多项式乘法
public GF28 multiply(GF28 other) {
BigInteger[] result = new BigInteger[this.degree() + other.degree()];
for (int i = 0; i < result.length; i++) {
result[i] = BigInteger.ZERO;
}
for (int i = 0; i <= this.degree(); i++) {
for (int j = 0; j <= other.degree(); j++) {
BigInteger coefficient = this.getCoefficient(i).multiply(other.getCoefficient(j));
result[i + j] = result[i + j].add(coefficient);
}
}
return new GF28(result);
}
// 获取系数
private BigInteger getCoefficient(int index) {
if (index < coefficients.length) {
return coefficients[index];
} else {
return BigInteger.ZERO;
}
}
// 返回多项式的度数
private int degree() {
return coefficients.length - 1;
}
// 多项式逆元,假设存在逆元
public boolean hasInverse() {
return gcd(this, BigInteger.ONE).compareTo(BigInteger.ONE) == 0;
}
// 计算两个多项式的最大公约数,用于测试是否可逆
private GF28 gcd(GF28 a, GF28 b) {
if (b.degree() == 0) {
return a;
}
GF28 temp = gcd(b, a.multiply(b.getCoefficient(0)).subtract(a));
return temp.degreesEven() ? temp : temp.shiftRight();
}
// 判断多项式的次数是否为偶数
private boolean degreesEven() {
return coefficients[degree()].bitLength() % 2 == 0;
}
// 左移操作模拟除以2的操作
private GF28 shiftRight() {
BigInteger[] shifted = new BigInteger[coefficients.length];
System.arraycopy(coefficients, 1, shifted, 0, coefficients.length - 1);
shifted[shifted.length - 1] = BigInteger.ZERO;
return new GF28(shifted);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
for (BigInteger c : coefficients) {
if (c.compareTo(BigInteger.ZERO) > 0) {
sb.append("X^").append(coefficients.length - 1 - coefficients.length).append(" + ");
}
sb.append(c.toString());
}
if (sb.length() > 0) {
sb.setLength(sb.length() - 2); // 去掉末尾的 "+ "
}
return sb.toString();
}
}
// 使用示例
public class Main {
public static void main(String[] args) {
GF28 p = new GF28(new BigInteger[]{BigInteger.ONE, BigInteger.ZERO}); // x+1
GF28 q = new GF28(new BigInteger[]{BigInteger.ZERO, BigInteger.ONE}); // x
GF28 product = p.multiply(q);
System.out.println("Multiplication: " + product);
if (p.hasInverse()) {
GF28 inverse = p.inverse();
System.out.println("Inversion: " + inverse);
} else {
System.out.println("The polynomial does not have an inverse.");
}
}
}
```
这个例子展示了如何创建一个有限域GF(2^8),并实现了多项式乘法和简单的一阶多项式求逆。请注意,对于更高阶的多项式逆元计算通常更复杂,可能需要采用更高级的算法如欧几里得算法或雅可比符号方法。此外,这里的示例假设输入多项式都是可逆的,实际应用中需要添加相应的错误处理。
阅读全文
相关推荐
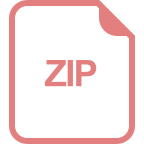
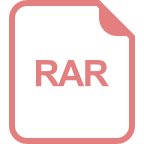

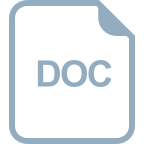







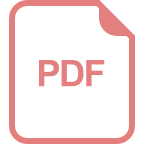
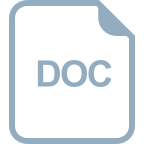
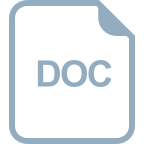
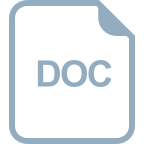
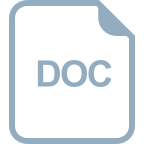

