新建 C++控制台应用程序,实现给定一条多段线 Polyline 和 任意一个点 P(x, y),计算返回点 P 到 Polyline 的最短距离
时间: 2024-09-11 17:10:08 浏览: 67
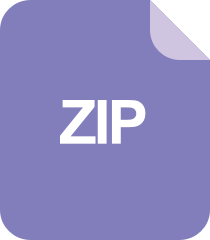
ss.zip_lsp程序_多段线_多段线闭合 lsp_面积lsp_面积标注
在C++中创建一个控制台应用程序来计算点到多段线的最短距离,通常涉及到一些几何学算法,例如狄卡尔距离公式或射线交点法。这里我们可以使用一种简化版本的方法,假设直线段连接两个端点,并采用欧几里得距离来估计最近的距离。
首先,你需要定义一个多段线的数据结构,可以包含一系列的线段 `[Segment start, end]`。然后,对于每个线段,你可以计算它通过点P的垂直投影长度,如果这个长度小于线段的实际长度,则点P就在该线段内部或边界上,直接返回该线段到点P的距离即可。如果点不在线段内,则继续检查下一个线段。
以下是一个简单的伪代码示例:
```cpp
#include <iostream>
#include <vector>
#include <cmath>
struct Point {
double x, y;
};
struct Segment {
Point start, end;
};
double distance(Segment s, Point p) {
// 计算垂直投影距离
double projection = abs(s.end.x - s.start.x) * (p.y - s.start.y) / (s.end.y - s.start.y);
// 如果投影在端点之间,则返回线段距离
if (projection >= std::min(s.start.x, s.end.x) && projection <= std::max(s.start.x, s.end.x)) {
return std::hypot(p.x - s.start.x, p.y - s.start.y);
} else {
// 点在两端点之外,考虑下一段线段
return std::min(std::min(distance(s, p), distance({s.end, Point{s.end.x, p.y}})), // 检查下一个线段
distance({Point{p.x, p.y}, s.end}, p)); // 检查当前线段反向的情况
}
}
int main() {
// 假设你有个多段线数据结构lines和一个点P
std::vector<Segment> lines;
Point P;
// 实现计算和读取数据的逻辑
double shortest_distance = distance(lines[0], P); // 从第一个线段开始
for (auto& line : lines) {
shortest_distance = std::min(shortest_distance, distance(line, P));
}
std::cout << "Point P to polyline's shortest distance is: " << shortest_distance << std::endl;
return 0;
}
```
注意这只是一个基础版的解决方案,实际应用中可能需要处理更多复杂情况,比如曲线段、多边形等。同时,上述代码并未优化遍历顺序,对于较长的多段线,可以考虑使用分治策略或其他搜索算法提高效率。
阅读全文
相关推荐
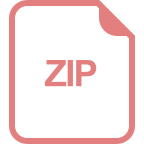















