stof' : is not a member of 'std'
时间: 2023-12-25 22:02:51 浏览: 64
如果你在使用`std::stof()`时遇到了错误"stof' : is not a member of 'std'",可能是因为你没有正确包含头文件`<string>`和`<iostream>`,或者你的编译器不支持C++11标准。
确保你的代码中包含了以下头文件:
```cpp
#include <iostream>
#include <string>
```
另外,你可以尝试使用`std::atof()`函数进行字符串到浮点数的转换。下面是一个示例:
```cpp
#include <iostream>
#include <cstdlib> // 包含 atof 函数的头文件
int main() {
const char* str = "3.14";
float f = std::atof(str);
std::cout << "Float value: " << f << std::endl;
return 0;
}
```
在上面的示例中,我们使用`std::atof()`函数将字符串"3.14"转换为浮点数,并将结果存储在变量`f`中。然后,我们使用`std::cout`输出该浮点数的值。注意,`std::atof()`函数接受一个C风格的字符串(`const char*`类型),而不是C++的`std::string`类型。
相关问题
std::string转数字
可以使用`std::stoi`、`std::stol`、`std::stoll`、`std::stof`、`std::stod`、`std::stold`等函数将`std::string`类型的字符串转换为数字类型。其中,`std::stoi`、`std::stol`、`std::stoll`分别用于将字符串转换为int、long、long long类型的数字,`std::stof`、`std::stod`、`std::stold`分别用于将字符串转换为float、double、long double类型的数字。这些函数都在头文件`<string>`中定义。
另外,也可以使用`std::stringstream`将`std::string`类型的字符串转换为数字类型。具体实现可以参考下面的代码:
```c++
#include <iostream>
#include <string>
#include <sstream>
template<typename out_type, typename in_value>
out_type convert(const in_value & t){
std::stringstream stream;
stream << t;
out_type result;
stream >> result;
return result;
}
int main() {
std::string str = "123";
int num1 = std::stoi(str);
long num2 = std::stol(str);
float num3 = std::stof(str);
double num4 = std::stod(str);
std::cout << num1 << " " << num2 << " " << num3 << " " << num4 << std::endl;
double num5 = convert<double>(str);
std::cout << num5 << std::endl;
return 0;
}
```
std::string转换为浮点型
要将std::string转换为浮点型,可以使用std::stof或std::stod函数,具体取决于你需要将字符串转换为float类型还是double类型。
如果你需要将字符串转换为float类型,可以使用std::stof函数。它接受一个字符串作为参数,并返回对应的浮点数值。
以下是使用std::stof函数将字符串转换为浮点型的示例代码:
```cpp
#include <iostream>
#include <string>
int main() {
std::string str = "3.14";
float num = std::stof(str);
std::cout << "转换后的浮点数为: " << num << std::endl;
return 0;
}
```
如果你需要将字符串转换为double类型,可以使用std::stod函数。它与std::stof的用法类似,只是返回的是double类型的浮点数值。
以下是使用std::stod函数将字符串转换为双精度浮点型的示例代码:
```cpp
#include <iostream>
#include <string>
int main() {
std::string str = "3.14";
double num = std::stod(str);
std::cout << "转换后的双精度浮点数为: " << num << std::endl;
return 0;
}
```
在上述示例中,将字符串"3.14"转换为浮点型,并将结果存储在变量num中。然后将num的值输出到控制台。
请注意,如果字符串无法转换为有效的浮点数,std::stof或std::stod函数将抛出一个std::invalid_argument异常。因此,在使用这些函数时,需要确保字符串确实可以成功转换为浮点数。
相关推荐
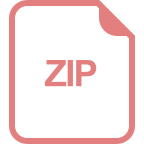
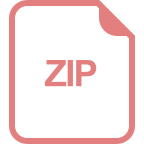
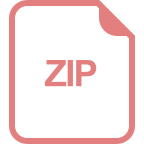












