建立单链表并求长度,用c语言
时间: 2024-10-11 09:03:39 浏览: 29
在 C 语言中,我们可以创建一个简单的单链表结构,并提供函数来插入节点和计算链表长度。这里是一个基础的例子:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构体
typedef struct Node {
int data;
struct Node* next;
} Node;
// 创建新节点并初始化数据
Node* createNode(int value) {
Node* newNode = (Node*)malloc(sizeof(Node));
if (newNode != NULL) {
newNode->data = value;
newNode->next = NULL; // 初始化下一个节点为空
}
return newNode;
}
// 插入节点到链表末尾
void appendToList(Node** head, int value) {
if (*head == NULL) { // 如果链表为空,创建头节点
*head = createNode(value);
} else {
Node* temp = *head;
while (temp->next != NULL) {
temp = temp->next;
}
temp->next = createNode(value); // 将新节点添加到末尾
}
}
// 计算链表长度
int listLength(Node* head) {
int length = 0;
if (head != NULL) {
Node* temp = head;
while (temp != NULL) {
length++;
temp = temp->next;
}
}
return length;
}
// 测试链表
int main() {
Node* head = NULL;
appendToList(&head, 1);
appendToList(&head, 2);
appendToList(&head, 3);
printf("链表长度: %d\n", listLength(head));
return 0;
}
```
在这个例子中,`createNode` 函数用于创建新节点,`appendToList` 函数负责将节点追加到链表尾部,而 `listLength` 函数则遍历整个链表计数。`main` 函数展示了如何创建和计算长度。
阅读全文
相关推荐
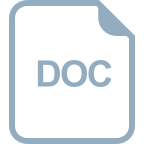
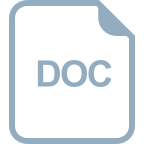
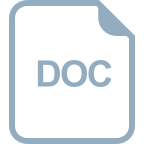






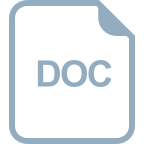








