用like判断新的字符串和给定字符串是否格式一直,给出python代码
时间: 2024-11-13 11:23:50 浏览: 5
在Python中,你可以使用`str.startswith()`和`str.endswith()`方法来检查新字符串是否以特定的前缀或后缀开始或结束,类似于SQL的LIKE操作符。这两个方法会返回布尔值,表示字符串是否满足条件。
例如,如果你想检查新的字符串`new_str`是否以给定的前缀`prefix`开始,可以这样做:
```python
prefix = "hello"
new_str = "hello world"
if new_str.startswith(prefix):
print("新字符串以指定的前缀开始")
else:
print("新字符串不以指定的前缀开始")
```
同样,如果你想知道它是否以后缀`suffix`结束,可以使用`endswith()`函数:
```python
suffix = "world"
if new_str.endswith(suffix):
print("新字符串以指定的后缀结束")
else:
print("新字符串不以指定的后缀结束")
```
如果需要更复杂的模式匹配,比如包含通配符,可以考虑正则表达式模块`re`,但这里仅限于基本的固定字符比较。
相关问题
判断新的字符串是否和给定字符串是同一种形式,给出python代码(用like判断)
在Python中,如果你想检查新字符串是否与给定字符串"在某种形式上相似",比如大小写不变、忽略某些特殊字符等,可以使用正则表达式(`re`模块)或者字符串的内置方法。这里有一个简单的例子,我们只考虑大小写的转换:
```python
import re
def is_same_form(new_str, given_str):
# 假设我们要比较的是忽略大小写的情况
new_str = new_str.lower() # 转换为小写
given_str = given_str.lower()
# 使用正则表达式匹配,如果两个字符串相同(除了空格),返回True
if re.match(r'^' + re.escape(given_str) + r'$', new_str, flags=re.IGNORECASE | re.DOTALL):
return True
else:
return False
# 示例
print(is_same_form("Hello World", "hello world")) # 输出: True
print(is_same_form("test!", "Test")) # 输出: False
统计一段长文本给定关键词出现的次数,python代码实现
在 Python 中统计一段长文本中给定关键词出现的次数,可以使用 Python 的字符串方法 `count()`。
例如,假设我们想要统计文本中 "apple" 这个词出现的次数,可以使用如下代码:
```python
text = "I have an apple. I like to eat apples. Apple is a fruit."
keyword = "apple"
count = text.count(keyword)
print(f"The keyword '{keyword}' appears {count} times in the text.")
```
输出结果如下:
```
The keyword 'apple' appears 3 times in the text.
```
注意,`count()` 方法是区分大小写的,如果需要忽略大小写,可以将文本和关键词都转换为小写或大写,然后再调用 `count()` 方法。例如:
```python
text = "I have an apple. I like to eat apples. Apple is a fruit."
keyword = "apple"
text = text.lower()
keyword = keyword.lower()
count = text.count(keyword)
print(f"The keyword '{keyword}' appears {count} times in the text.")
```
输出结果如下:
```
The keyword 'apple' appears 3 times in the text.
```
也可以使用正则表达式来忽略大小写,例如:
```python
import re
text = "I have an apple. I like to eat apples. Apple is a fruit."
keyword = "apple"
pattern = re.compile(keyword, re.IGNORECASE)
count = len(pattern.findall(text))
print(f"The keyword '{keyword}' appears {count} times in the text.")
```
输出结果同上。
此外,如果需要统计多个关键词的出现次数,可以使用循环来处理每个关键词,然后累加统计结果即可。
阅读全文
相关推荐
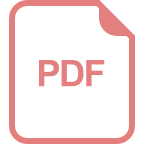
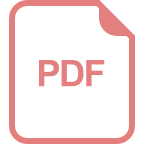
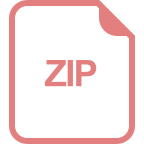
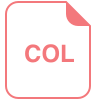
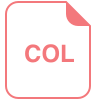
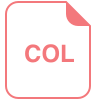
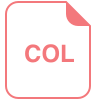
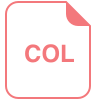
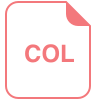

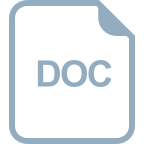
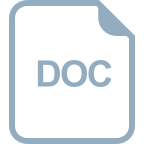
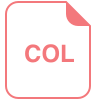
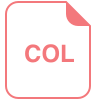
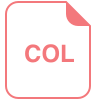
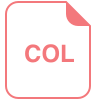
