将文件中的代码使用vue3实现
时间: 2024-12-09 18:17:09 浏览: 11
将这段 Vue 2 代码迁移到 Vue 3 需要考虑以下几个主要方面的变更:
1. **组件结构**:Vue 3 推荐使用组合式 API(Composition API)而不是选项式 API。
2. **生命周期钩子**:Vue 3 中的部分生命周期钩子有变化,例如 `mounted` 替换为 `onMounted`。
3. **模版编译**:虽然模版本身的变化不大,但需要确保所有依赖项和插件都支持 Vue 3。
以下是如何将给定的代码迁移至 Vue 3,并尽量保持功能不变:
### Step 1: 安装 Vue 3 及相关依赖
确保你已经安装了 Vue 3 和相关的包:
```sh
npm install vue@next axios lodash.isequal leaflet vue3-loading-overlay ant-design-vue
```
### Step 2: 修改代码
以下是修改后的代码:
```html
<template>
<a-spin :loading="confirmLoading">
<a-tabs :active-key="valKey" @change="handleTabChange" style="width: 97%; margin: 0 auto 20px">
<a-tab-pane key="1" tab="基础信息"></a-tab-pane>
<a-tab-pane key="2" tab="其他信息"></a-tab-pane>
</a-tabs>
<j-form-container class="add-site" :disabled="type === 'info'">
<a-form :model="form" ref="formRef">
<!-- 表单项 -->
<div v-if="valKey == '1'">
<!-- 基本信息 -->
<a-form-item label="设备编号" prop="equipmentNumber">
<a-input v-model="form.equipmentNumber" placeholder="请输入设备编号" maxlength="64"></a-input>
</a-form-item>
<a-form-item label="设备名称" prop="name">
<a-input v-model="form.name" placeholder="请输入设备名称" maxlength="32"></a-input>
</a-form-item>
<!-- 其他字段... -->
</div>
<div v-if="valKey == '2'">
<!-- 材质信息 -->
<a-form-item label="材质" prop="material">
<a-input v-model="form.material" placeholder="请输入材质" maxlength="32"></a-input>
</a-form-item>
<!-- 其他字段... -->
</div>
<!-- 提交按钮 -->
<a-button type="primary" @click="handleSubmit">提交</a-button>
</a-form>
</j-form-container>
</a-spin>
</template>
<script setup>
import { ref, reactive, onMounted, toRefs, watch } from 'vue';
import { useStore } from 'vuex';
import axios from 'axios';
import L from 'leaflet';
import { AMap, AMapAutocomplete, AMapPlaceSearch } from '@amap/amap-js-api-loader';
const store = useStore();
const confirmLoading = ref(false);
const formRef = ref(null);
const form = reactive({
equipmentNumber: '',
name: '',
// 其他字段...
});
const valKey = ref('1');
const fileList = ref([]);
const url = {
add: '/web/gisInspectionEquipment/add',
edit: '/web/gisInspectionEquipment/edit',
queryById: '/web/gisInspectionEquipment/queryById',
getPipelines: '/web/gisInspectionPipeline/getSuperiorPipelines',
};
// 生命周期钩子
onMounted(async () => {
await initLeafletMap();
// 初始化其他数据
});
// 方法
const handleTabChange = (key) => {
valKey.value = key;
};
const handleSubmit = () => {
formRef.value.validate(async (valid) => {
if (valid) {
confirmLoading.value = true;
try {
let response;
if (!form.id) {
response = await axios.post(url.add, form);
} else {
response = await axios.put(url.edit, form);
}
console.log('Response:', response);
} catch (error) {
console.error('Error:', error);
} finally {
confirmLoading.value = false;
}
}
});
};
// 地图初始化
const initLeafletMap = async () => {
const locationIcon = L.icon({
iconUrl: '/path/to/your/icon.svg',
iconSize: [60, 64],
iconAnchor: [25, 55]
});
const map = new L.Map('mapformid', {
zoom: 14,
center: store.getters.gisMapCenter,
boxZoom: true,
zoomControl: false,
doubleClickZoom: false,
attributionControl: false,
preferCanvas: true
});
L.control.scale().addTo(map);
const layer = L.tileLayer(
'//webrd0{s}.is.autonavi.com/appmaptile?lang=zh_cn&size=1&scale=1&style=8&x={x}&y={y}&z={z}',
{
maxZoom: 20,
maxNativeZoom: 18,
minZoom: 3,
attribution: '高德地图 AutoNavi.com',
subdomains: '1234'
}
).addTo(map);
L.control.zoom().addTo(map);
await AMapLoader.load({
key: 'Your_AMap_Key', // 申请的应用APPKey
version: '2.0', // 指定要加载的JSAPI的版本,默认为1.4.15
plugins: ['AMap.Autocomplete', 'AMap.PlaceSearch']
});
const auto = new AMap.Autocomplete({ input: 'addrFormInput' });
const placeSearch = new AMap.PlaceSearch({ map });
auto.on('select', (e) => {
if (e.poi.location) {
map.setView([e.poi.location.lat, e.poi.location.lng]);
L.marker([e.poi.location.lat, e.poi.location.lng], { icon: locationIcon }).addTo(map);
}
});
map.on('click', (e) => {
// 更新表单数据
});
return map;
};
</script>
<style scoped>
.title {
color: rgba(0, 0, 0, 0.85);
font-size: 14px;
font-weight: 500;
margin-bottom: 16px;
}
.count {
position: absolute;
right: 3px;
bottom: -26px;
font-size: 12px;
line-height: 1;
}
/* 其他样式 */
</style>
```
### 关键点解释
1. **模板部分**:大部分模版保留原样,只是调整了一些属性名称(如 `:model` 和 `v-model`)以符合 Vue 3 的规范。
2. **脚本部分**:使用 Composition API 进行状态管理和方法定义。
3. **生命周期钩子**:将 Vue 2 的 `created` 或 `mounted` 转换成 Vue 3 的 `onMounted`。
4. **地图初始化**:引入并初始化 Leaflet 和 AMap。
希望这些修改能帮助你将代码成功迁移到 Vue 3。如果有任何问题或进一步的需求,请随时告诉我。
阅读全文
相关推荐
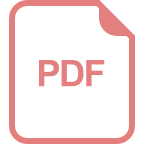
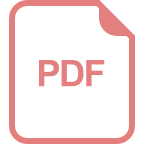
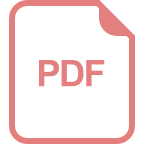
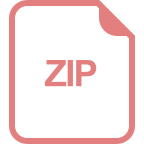
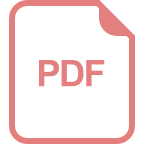
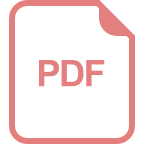
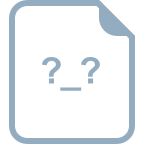
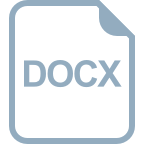
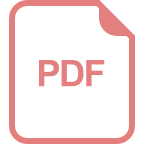
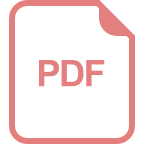
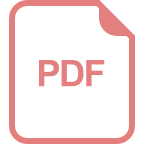
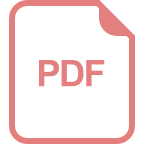
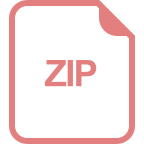
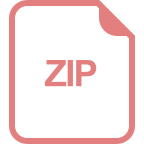
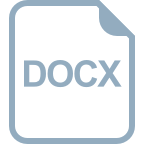
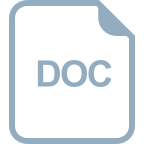
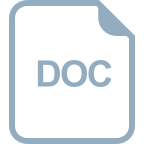
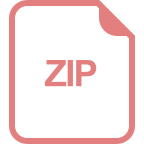