严重性 代码 说明 项目 文件 行 禁止显示状态 错误(活动) E0020 未定义标识符 "EGE_WHITE" GUI C:\Users\86187\source\repos\GUI\源.cpp 11
时间: 2024-03-28 20:33:55 浏览: 28
根据提供的引用内容,你遇到了一个编译错误,错误信息是"未定义标识符 'EGE_WHITE'"。这个错误通常是由于缺少必要的头文件或库文件导致的。在你的代码中,你使用了一个名为'EGE_WHITE'的标识符,但编译器无法找到它的定义。
为了解决这个问题,你需要确保你的代码中包含了定义'EGE_WHITE'的头文件或库文件。根据你提供的信息,你可能需要添加一个名为'EGE'的图形绘制库。你可以在CodeBlocks中添加这个库,然后在你的代码中包含相应的头文件。
请检查以下几个方面来解决这个问题:
1. 确保你已经正确安装了'EGE'图形绘制库,并将其添加到了CodeBlocks中。
2. 在你的代码中,使用`#include`指令包含正确的头文件,以便使用'EGE_WHITE'标识符。
3. 确保你的代码中没有拼写错误或语法错误,这可能导致编译器无法找到'EGE_WHITE'的定义。
如果你已经按照上述步骤操作,并且仍然遇到相同的错误,请检查你的代码中是否有其他错误或缺少其他必要的库文件。
相关问题
EGE图形库滚动条控件示例代码
EGE图形库是一款简单易用的图形库,它支持绘制基本的图形、文字,还有一些常用的控件,如按钮、文本框、滚动条等。下面是一个简单的EGE图形库滚动条控件示例代码:
```
#include <graphics.h>
#include <conio.h>
int main()
{
initgraph(640, 480); // 初始化图形界面
int x = 300; // 滚动条位置
int barWidth = 20; // 滚动条宽度
int barHeight = 100; // 滚动条高度
int scrollHeight = 300; // 滚动区域高度
int barTop = (480 - barHeight) / 2; // 滚动条顶部位置
int scrollTop = (480 - scrollHeight) / 2; // 滚动区域顶部位置
while (true)
{
cleardevice(); // 清空屏幕
// 绘制滚动区域
setfillcolor(LIGHTGRAY);
bar(100, scrollTop, 540, scrollTop + scrollHeight);
// 绘制滚动条背景
setfillcolor(WHITE);
bar(x, barTop, x + barWidth, barTop + barHeight);
// 绘制滚动条
setfillcolor(DARKGRAY);
bar(x, barTop + (scrollHeight - barHeight) * (x - 100) / (540 - 100 - barWidth), x + barWidth, barTop + (scrollHeight - barHeight) * (x - 100) / (540 - 100 - barWidth) + barHeight);
if (kbhit()) // 监听键盘事件
{
char ch = getch();
if (ch == 'w' || ch == 'W' || ch == VK_UP) // 向上移动滚动条
{
if (x > 100)
{
x--;
}
}
else if (ch == 's' || ch == 'S' || ch == VK_DOWN) // 向下移动滚动条
{
if (x < 540 - barWidth)
{
x++;
}
}
}
delay(10); // 延迟10毫秒,控制帧率
flushmouse(); // 刷新鼠标状态
}
closegraph(); // 关闭图形界面
return 0;
}
```
这段代码实现了一个简单的滚动条控件,可以通过键盘上下箭头或W、S键来控制滚动条的位置。滚动条会根据位置的改变,自动调整滚动区域的显示内容。
如何 从“ege::COLORS”转换为“ege::color_t”
可以使用以下方法将ege::COLORS转换为ege::color_t:
1. 首先,需要了解ege::COLORS是一个枚举类型,它列出了一些常用颜色的名称和对应的RGB值。
2. 其次,需要知道ege::color_t是一个结构体,它包含了红、绿、蓝三个通道的值。
3. 为了将ege::COLORS转换为ege::color_t,需要使用下面的代码:
```
ege::color_t color = ege::color_t(ege::COLORS::颜色名称);
```
例如,将ege::COLORS::RED转换为ege::color_t:
```
ege::color_t color = ege::color_t(ege::COLORS::RED);
```
这将创建一个ege::color_t实例,其中红色通道的值为255,绿色和蓝色通道的值为0。
相关推荐
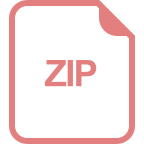
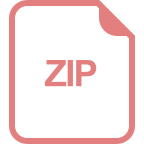












