扫雷图形化ege库鼠标操作代码
时间: 2023-11-13 19:06:06 浏览: 54
以下是使用ege库实现扫雷图形化界面的鼠标操作代码:
```c++
#include <graphics.h>
#include <conio.h>
#include <time.h>
#include <stdlib.h>
#include <stdio.h>
#include <Windows.h>
#define COLS 30
#define ROWS 16
#define MINES 99
#define BLOCK_SIZE 25
#define WINDOW_WIDTH COLS*BLOCK_SIZE
#define WINDOW_HEIGHT ROWS*BLOCK_SIZE
int x, y;
struct Block {
int x, y;
int value;
bool is_mine;
bool is_opened;
bool is_flagged;
};
Block blocks[ROWS][COLS];
int count_mines(int row, int col) {
int count = 0;
for (int i = -1; i <= 1; i++) {
for (int j = -1; j <= 1; j++) {
int r = row + i;
int c = col + j;
if (r >= 0 && r < ROWS && c >= 0 && c < COLS && blocks[r][c].is_mine) {
count++;
}
}
}
return count;
}
void draw_block(int row, int col) {
setfillcolor(LIGHTGRAY);
if (blocks[row][col].is_opened || blocks[row][col].is_flagged) {
setfillcolor(WHITE);
}
fillrectangle(col*BLOCK_SIZE, row*BLOCK_SIZE, (col + 1)*BLOCK_SIZE, (row + 1)*BLOCK_SIZE);
if (blocks[row][col].is_opened) {
if (blocks[row][col].is_mine) {
setfillcolor(RED);
solidcircle(col*BLOCK_SIZE + BLOCK_SIZE / 2, row*BLOCK_SIZE + BLOCK_SIZE / 2, BLOCK_SIZE / 2 - 2);
}
else {
settextcolor(BLUE);
char str[2] = "\0";
str[0] = blocks[row][col].value + '0';
outtextxy(col*BLOCK_SIZE + BLOCK_SIZE / 2 - 5, row*BLOCK_SIZE + BLOCK_SIZE / 2 - 5, str);
}
}
else if (blocks[row][col].is_flagged) {
settextcolor(RED);
char str[2] = "\0";
str[0] = 'F';
outtextxy(col*BLOCK_SIZE + BLOCK_SIZE / 2 - 5, row*BLOCK_SIZE + BLOCK_SIZE / 2 - 5, str);
}
}
void draw_board() {
cleardevice();
for (int row = 0; row < ROWS; row++) {
for (int col = 0; col < COLS; col++) {
draw_block(row, col);
}
}
}
void init_blocks() {
for (int row = 0; row < ROWS; row++) {
for (int col = 0; col < COLS; col++) {
blocks[row][col].x = col;
blocks[row][col].y = row;
blocks[row][col].value = 0;
blocks[row][col].is_mine = false;
blocks[row][col].is_opened = false;
blocks[row][col].is_flagged = false;
}
}
int count = 0;
while (count < MINES) {
int row = rand() % ROWS;
int col = rand() % COLS;
if (!blocks[row][col].is_mine) {
blocks[row][col].is_mine = true;
count++;
}
}
for (int row = 0; row < ROWS; row++) {
for (int col = 0; col < COLS; col++) {
if (!blocks[row][col].is_mine) {
blocks[row][col].value = count_mines(row, col);
}
}
}
}
void open_block(int row, int col) {
if (row < 0 || row >= ROWS || col < 0 || col >= COLS || blocks[row][col].is_opened || blocks[row][col].is_flagged) {
return;
}
blocks[row][col].is_opened = true;
if (blocks[row][col].is_mine) {
return;
}
if (blocks[row][col].value > 0) {
return;
}
open_block(row - 1, col - 1);
open_block(row - 1, col);
open_block(row - 1, col + 1);
open_block(row, col - 1);
open_block(row, col + 1);
open_block(row + 1, col - 1);
open_block(row + 1, col);
open_block(row + 1, col + 1);
}
void game_over() {
settextcolor(RED);
char str[20] = "Game Over!";
outtextxy(WINDOW_WIDTH / 2 - 50, WINDOW_HEIGHT / 2 - 5, str);
}
void game_win() {
settextcolor(GREEN);
char str[20] = "You Win!";
outtextxy(WINDOW_WIDTH / 2 - 40, WINDOW_HEIGHT / 2 - 5, str);
}
void game() {
bool gameover = false;
bool gamewin = false;
while (!gameover && !gamewin) {
draw_board();
if (kbhit()) {
char c = getch();
if (c == ' ') {
if (blocks[y][x].is_opened) {
open_block(y, x);
}
else if (blocks[y][x].is_flagged) {
blocks[y][x].is_flagged = false;
}
else {
blocks[y][x].is_flagged = true;
}
}
if (c == 'q') {
gameover = true;
}
}
if (GetAsyncKeyState(VK_LBUTTON)) {
int mx, my;
mousepos(&mx, &my);
int col = mx / BLOCK_SIZE;
int row = my / BLOCK_SIZE;
if (row >= 0 && row < ROWS && col >= 0 && col < COLS) {
if (!blocks[row][col].is_flagged) {
if (blocks[row][col].is_mine) {
gameover = true;
}
else {
open_block(row, col);
}
}
}
}
if (GetAsyncKeyState(VK_RBUTTON)) {
int mx, my;
mousepos(&mx, &my);
int col = mx / BLOCK_SIZE;
int row = my / BLOCK_SIZE;
if (row >= 0 && row < ROWS && col >= 0 && col < COLS) {
if (!blocks[row][col].is_opened) {
blocks[row][col].is_flagged = !blocks[row][col].is_flagged;
}
}
}
int count_opened = 0;
int count_flagged = 0;
for (int row = 0; row < ROWS; row++) {
for (int col = 0; col < COLS; col++) {
if (blocks[row][col].is_opened) {
count_opened++;
}
if (blocks[row][col].is_flagged) {
count_flagged++;
}
}
}
if (count_opened == ROWS*COLS - MINES) {
gamewin = true;
}
Sleep(50);
}
draw_board();
if (gameover) {
game_over();
}
else {
game_win();
}
}
int main() {
srand(time(NULL));
initgraph(WINDOW_WIDTH, WINDOW_HEIGHT);
init_blocks();
draw_board();
game();
getch();
closegraph();
return 0;
}
```
该程序实现了扫雷的基本功能,包括鼠标左键点击方块、鼠标右键标记方块、空白方块自动展开、游戏胜利和游戏失败等。
相关推荐
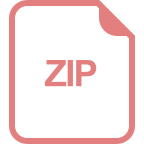














