c语言 ege库实现五子棋人机和人人对战
时间: 2023-10-10 16:15:55 浏览: 79
使用EGE图形库来实现五子棋人机和人人对战可以让游戏更加直观和交互性。下面是一个使用C语言和EGE库编写的示例代码,实现了五子棋的人机和人人对战功能。
```c
#include <stdio.h>
#include <graphics.h>
#define ROWS 15
#define COLS 15
#define SIZE 40
#define MARGIN 20
int chessboard[ROWS][COLS];
int currentPlayer = 1; // 当前玩家,1表示黑棋,2表示白棋
int gameMode = 0; // 游戏模式,0表示人机对战,1表示人人对战
int gameOver = 0; // 游戏是否结束
void drawChessboard() {
setfillcolor(RGB(255, 206, 158)); // 设置棋盘背景颜色
solidrectangle(MARGIN, MARGIN, MARGIN + COLS * SIZE, MARGIN + ROWS * SIZE);
setlinecolor(BLACK); // 设置棋盘线条颜色
// 绘制棋盘线条
for (int i = 0; i <= ROWS; i++) {
line(MARGIN, MARGIN + i * SIZE, MARGIN + COLS * SIZE, MARGIN + i * SIZE);
}
for (int j = 0; j <= COLS; j++) {
line(MARGIN + j * SIZE, MARGIN, MARGIN + j * SIZE, MARGIN + ROWS * SIZE);
}
// 绘制棋子
for (int i = 0; i < ROWS; i++) {
for (int j = 0; j < COLS; j++) {
if (chessboard[i][j] == 1) {
setfillcolor(BLACK);
solidcircle(MARGIN + j * SIZE, MARGIN + i * SIZE, SIZE / 2 - 2);
} else if (chessboard[i][j] == 2) {
setfillcolor(WHITE);
solidcircle(MARGIN + j * SIZE, MARGIN + i * SIZE, SIZE / 2 - 2);
}
}
}
}
void initChessboard() {
for (int i = 0; i < ROWS; i++) {
for (int j = 0; j < COLS; j++) {
chessboard[i][j] = 0;
}
}
}
void placePiece(int row, int col) {
chessboard[row][col] = currentPlayer;
}
int checkWin(int row, int col) {
int directions[4][2] = { {1, 0}, {0, 1}, {1, 1}, {-1, 1} };
for (int i = 0; i < 4; i++) {
int count = 1;
int dx = directions[i][0];
int dy = directions[i][1];
// 向正反两个方向搜索
for (int j = 1; j < 5; j++) {
int newRow = row + j * dx;
int newCol = col + j * dy;
if (newRow >= 0 && newRow < ROWS && newCol >= 0 && newCol < COLS && chessboard[newRow][newCol] == currentPlayer) {
count++;
} else {
break;
}
}
for (int j = 1; j < 5; j++) {
int newRow = row - j * dx;
int newCol = col - j * dy;
if (newRow >= 0 && newRow < ROWS && newCol >= 0 && newCol < COLS && chessboard[newRow][newCol] == currentPlayer) {
count++;
} else {
break;
}
}
if (count >= 5) {
return currentPlayer;
}
}
return 0;
}
void aiMove() {
// 随机选择一个空位落子
while (1) {
int row = rand() % ROWS;
int col = rand() % COLS;
if (chessboard[row][col] == 0) {
placePiece(row, col);
break;
}
}
}
void gameLoop() {
initgraph(COLS * SIZE + 2 * MARGIN, ROWS * SIZE + 2 * MARGIN);
while (!gameOver) {
drawChessboard();
if (gameMode == 0 && currentPlayer == 2) {
aiMove();
} else {
MOUSEMSG m;
m = GetMouseMsg();
if (m.uMsg == WM_LBUTTONDOWN) {
int x = m.x - MARGIN;
int y = m.y - MARGIN;
if (x >= 0 && x < COLS * SIZE && y >= 0 && y < ROWS * SIZE) {
int row = y / SIZE;
int col = x / SIZE;
if (chessboard[row][col] == 0) {
placePiece(row, col);
int winner = checkWin(row, col);
if (winner > 0) {
gameOver = 1;
char msg[20];
sprintf(msg, "Player %d wins!", winner);
MessageBox(GetHWnd(), msg, "Game Over", MB_OK);
} else {
currentPlayer = (currentPlayer == 1) ? 2 : 1;
}
}
}
}
}
}
closegraph();
}
int main() {
gameLoop();
return 0;
}
```
这段代码使用了EGE图形库来绘制棋盘和棋子,并且支持人机对战和人人对战两种游戏模式。在人机对战模式下,计算机会随机选择一个空位落子;在人人对战模式下,玩家轮流下棋。当有玩家获胜时,会弹出提示框显示胜利信息。
你需要在Windows环境下安装EGE库,并使用支持EGE库的C语言编译器来编译和运行这段代码。如果你想更深入了解EGE库的使用,可以查阅相关文档和教程。
相关推荐
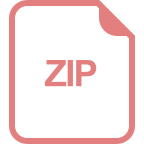














