用ege做一个计算器 的代码
时间: 2024-04-30 10:25:02 浏览: 226
以下是使用ege库实现简单计算器的代码:
```c++
#include <graphics.h>
#include <conio.h>
#include <stdio.h>
#include <stdlib.h>
#include <math.h>
#define WIDTH 270
#define HEIGHT 360
int main() {
initgraph(WIDTH, HEIGHT);
setbkcolor(WHITE);
char input[20] = "";
int x = 10, y = 10;
outtextxy(x, y, "Calculator");
settextstyle(10, 0, 3);
setcolor(BLACK);
rectangle(x, y + 50, x + 250, y + 290);
settextstyle(10, 0, 2);
int i = 0, j = 0;
for (i = 0; i < 3; i++) {
for (j = 0; j < 3; j++) {
char num[2];
sprintf(num, "%d", i * 3 + j + 1);
rectangle(x + j * 80, y + i * 80 + 60, x + j * 80 + 70, y + i * 80 + 140);
outtextxy(x + j * 80 + 30, y + i * 80 + 100, num);
}
}
outtextxy(x + 30, y + 300, "0");
rectangle(x + 80, y + 300, x + 160, y + 340);
outtextxy(x + 110, y + 310, ".");
rectangle(x, y + 300, x + 70, y + 340);
outtextxy(x + 30, y + 310, "C");
rectangle(x + 170, y + 60, x + 250, y + 100);
outtextxy(x + 190, y + 80, "+");
rectangle(x + 170, y + 120, x + 250, y + 160);
outtextxy(x + 190, y + 140, "-");
rectangle(x + 170, y + 180, x + 250, y + 220);
outtextxy(x + 190, y + 200, "*");
rectangle(x + 170, y + 240, x + 250, y + 280);
outtextxy(x + 190, y + 260, "/");
while (1) {
char ch = getch();
if (ch >= '0' && ch <= '9') {
input[strlen(input)] = ch;
outtextxy(x + 15, y + 310, input);
}
else if (ch == '.') {
if (strchr(input, '.') == NULL) {
input[strlen(input)] = ch;
outtextxy(x + 15, y + 310, input);
}
}
else if (ch == '+' || ch == '-' || ch == '*' || ch == '/') {
char num[20];
double a = atof(input);
memset(input, 0, sizeof(input));
sprintf(num, "%.2f", a);
outtextxy(x + 15, y + 310, num);
input[strlen(input)] = ch;
}
else if (ch == 8) {
if (strlen(input) > 0) {
input[strlen(input) - 1] = '\0';
cleardevice();
setbkcolor(WHITE);
outtextxy(x, y, "Calculator");
settextstyle(10, 0, 3);
setcolor(BLACK);
rectangle(x, y + 50, x + 250, y + 290);
settextstyle(10, 0, 2);
int i = 0, j = 0;
for (i = 0; i < 3; i++) {
for (j = 0; j < 3; j++) {
char num[2];
sprintf(num, "%d", i * 3 + j + 1);
rectangle(x + j * 80, y + i * 80 + 60, x + j * 80 + 70, y + i * 80 + 140);
outtextxy(x + j * 80 + 30, y + i * 80 + 100, num);
}
}
outtextxy(x + 30, y + 300, "0");
rectangle(x + 80, y + 300, x + 160, y + 340);
outtextxy(x + 110, y + 310, ".");
rectangle(x, y + 300, x + 70, y + 340);
outtextxy(x + 30, y + 310, "C");
rectangle(x + 170, y + 60, x + 250, y + 100);
outtextxy(x + 190, y + 80, "+");
rectangle(x + 170, y + 120, x + 250, y + 160);
outtextxy(x + 190, y + 140, "-");
rectangle(x + 170, y + 180, x + 250, y + 220);
outtextxy(x + 190, y + 200, "*");
rectangle(x + 170, y + 240, x + 250, y + 280);
outtextxy(x + 190, y + 260, "/");
outtextxy(x + 15, y + 310, input);
}
}
else if (ch == 13) {
double a = atof(input);
double b = 0;
char num[20];
memset(input, 0, sizeof(input));
if (strchr(num, '+') != NULL) {
b = atof(strchr(num, '+') + 1);
sprintf(num, "%.2f", a + b);
}
else if (strchr(num, '-') != NULL) {
b = atof(strchr(num, '-') + 1);
sprintf(num, "%.2f", a - b);
}
else if (strchr(num, '*') != NULL) {
b = atof(strchr(num, '*') + 1);
sprintf(num, "%.2f", a * b);
}
else if (strchr(num, '/') != NULL) {
b = atof(strchr(num, '/') + 1);
sprintf(num, "%.2f", a / b);
}
outtextxy(x + 15, y + 310, num);
}
else if (ch == 'c' || ch == 'C') {
memset(input, 0, sizeof(input));
outtextxy(x + 15, y + 310, input);
}
}
getch();
closegraph();
return 0;
}
```
这个计算器只支持加、减、乘、除四种运算,并且没有考虑到负数、小数、括号以及其他高级运算。如果需要实现更复杂的计算器,可以考虑使用其他第三方库或者自己实现计算逻辑。
阅读全文
相关推荐
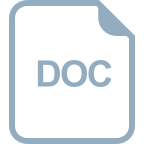
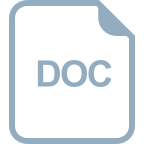
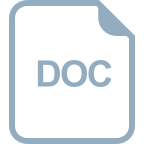

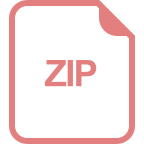
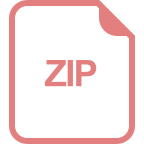
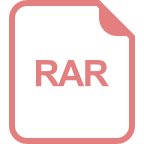










