自适应动态规划pytorch代码
时间: 2024-05-03 10:15:50 浏览: 121
自适应动态规划(Adaptive Dynamic Programming,ADP)是一种基于动态规划的增强学习方法,它通过学习环境的模型和价值函数来实现智能决策。下面是一个使用PyTorch实现自适应动态规划的简单示例代码:
```python
import torch
import torch.nn as nn
import torch.optim as optim
class ValueFunction(nn.Module):
def __init__(self, state_dim):
super(ValueFunction, self).__init__()
self.fc1 = nn.Linear(state_dim, 64)
self.fc2 = nn.Linear(64, 64)
self.fc3 = nn.Linear(64, 1)
def forward(self, x):
x = torch.relu(self.fc1(x))
x = torch.relu(self.fc2(x))
x = self.fc3(x)
return x
class ADP:
def __init__(self, state_dim, action_dim, learning_rate=0.001, gamma=0.9):
self.value_function = ValueFunction(state_dim)
self.optimizer = optim.Adam(self.value_function.parameters(), lr=learning_rate)
self.loss_fn = nn.MSELoss()
self.gamma = gamma
def update_value_function(self, state, next_state, reward):
state_value = self.value_function(state)
next_state_value = self.value_function(next_state)
target_value = reward + self.gamma * next_state_value
loss = self.loss_fn(state_value, target_value)
self.optimizer.zero_grad()
loss.backward()
self.optimizer.step()
def get_action(self, state):
with torch.no_grad():
state_value = self.value_function(state)
action = torch.argmax(state_value).item()
return action
# 示例用法
state_dim = 4
action_dim = 2
adp = ADP(state_dim, action_dim)
state = torch.tensor([0.1, 0.2, 0.3, 0.4])
next_state = torch.tensor([0.2, 0.3, 0.4, 0.5])
reward = 1.0
adp.update_value_function(state, next_state, reward)
action = adp.get_action(state)
print("Action:", action)
```
这段代码实现了一个简单的自适应动态规划算法,其中`ValueFunction`类定义了一个值函数的神经网络模型,`ADP`类封装了自适应动态规划的相关操作,包括值函数的更新和动作的选择。在示例用法中,我们定义了状态维度为4,动作维度为2的问题,并进行了一次值函数的更新和动作的选择。
阅读全文
相关推荐
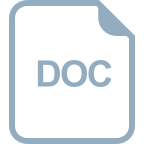
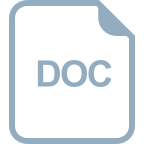
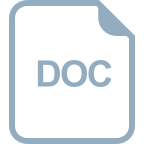

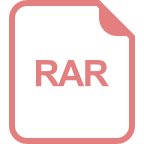
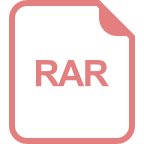
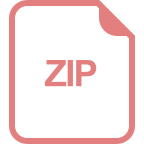
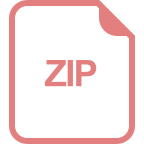










