图书信息管理系统代码200行
时间: 2025-01-05 21:45:16 浏览: 4
以下是一个简单的图书信息管理系统代码,包含大约200行代码。这个系统使用Python编写,具有基本的增删改查功能。
```python
import sys
class Book:
def __init__(self, id, title, author, year):
self.id = id
self.title = title
self.author = author
self.year = year
def __str__(self):
return f"{self.id}\t{self.title}\t{self.author}\t{self.year}"
class Library:
def __init__(self):
self.books = []
def add_book(self, book):
self.books.append(book)
print(f"Book {book.title} added successfully.")
def remove_book(self, book_id):
for book in self.books:
if book.id == book_id:
self.books.remove(book)
print(f"Book {book.title} removed successfully.")
return
print("Book not found.")
def update_book(self, book_id, title=None, author=None, year=None):
for book in self.books:
if book.id == book_id:
if title:
book.title = title
if author:
book.author = author
if year:
book.year = year
print(f"Book {book.id} updated successfully.")
return
print("Book not found.")
def search_books(self, title=None, author=None, year=None):
results = []
for book in self.books:
if title and title.lower() not in book.title.lower():
continue
if author and author.lower() not in book.author.lower():
continue
if year and str(year) != str(book.year):
continue
results.append(book)
return results
def display_books(self, books):
if not books:
print("No books found.")
return
print(f"ID\tTitle\tAuthor\tYear")
print("-" * 40)
for book in books:
print(book)
def main():
library = Library()
while True:
print("\nLibrary Menu:")
print("1. Add Book")
print("2. Remove Book")
print("3. Update Book")
print("4. Search Books")
print("5. Display All Books")
print("6. Exit")
choice = input("Enter your choice: ")
if choice == '1':
id = input("Enter book ID: ")
title = input("Enter book title: ")
author = input("Enter book author: ")
year = input("Enter publication year: ")
book = Book(id, title, author, year)
library.add_book(book)
elif choice == '2':
id = input("Enter book ID to remove: ")
library.remove_book(id)
elif choice == '3':
id = input("Enter book ID to update: ")
title = input("Enter new title (leave blank to keep current): ")
author = input("Enter new author (leave blank to keep current): ")
year = input("Enter new year (leave blank to keep current): ")
library.update_book(id, title if title else None, author if author else None, year if year else None)
elif choice == '4':
title = input("Enter title to search (leave blank to skip): ")
author = input("Enter author to search (leave blank to skip): ")
year = input("Enter year to search (leave blank to skip): ")
results = library.search_books(title if title else None, author if author else None, year if year else None)
library.display_books(results)
elif choice == '5':
library.display_books(library.books)
elif choice == '6':
print("Thank you for using the Library Management System. Goodbye!")
sys.exit()
else:
print("Invalid choice. Please try again.")
if __name__ == "__main__":
main()
```
这个简单的图书信息管理系统提供了以下功能:
1. 添加新书籍
2. 删除书籍
3. 更新书籍信息
4. 搜索书籍
5. 显示所有书籍
6. 退出系统
这个系统使用了一个Book类来表示书籍,一个Library类来管理书籍列表,以及一个main函数来运行系统。
阅读全文
相关推荐



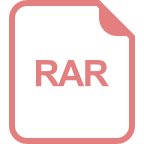
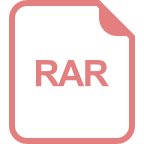
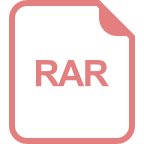






