编程3:输入两个整数表示年和月,输入年份为2000-2500之间,输出一个整数,即该月的天数。如果输入不是2000-2500,则打印不符合规则用C语言
时间: 2024-11-13 11:25:07 浏览: 2
在C语言中,你可以使用`stdio.h`库来进行用户输入,并结合`calendar.h`库中的函数来计算给定月份的天数。请注意,`calendar.h`库在一些编译环境中可能不可用,这时可以手动编写针对每个月的天数判断逻辑。以下是一个简单的示例程序,不依赖于`calendar.h`:
```c
#include <stdio.h>
int getDaysInMonth(int year, int month) {
// 检查年份是否在指定范围内
if (year < 2000 || year > 2500) {
printf("不符合规则:请输入2000-2500之间的年份。\n");
return -1; // 或者其他错误处理方式
}
// 非闰年的二月只有28天,其余月份直接返回对应月份的天数
switch (month) {
case 1:
case 3:
case 5:
case 7:
case 8:
case 10:
case 12:
return 31;
case 4:
case 6:
case 9:
case 11:
return 30;
case 2: // 特殊处理闰年的二月
if ((year % 4 == 0 && year % 100 != 0) || (year % 400 == 0)) { // 符合闰年条件
return 29;
} else {
return 28;
}
default:
printf("错误:输入的月份无效。\n");
return -1; // 或者其他错误处理方式
}
}
int main() {
int year, month;
printf("请输入年份: ");
scanf("%d", &year);
printf("请输入月份: ");
scanf("%d", &month);
int days = getDaysInMonth(year, month);
if (days != -1) {
printf("该月的天数为: %d\n", days);
}
return 0;
}
```
阅读全文
相关推荐
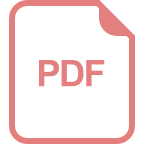
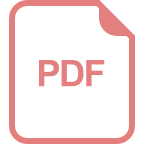
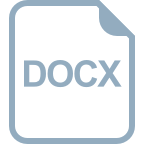











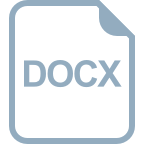
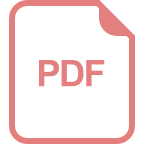
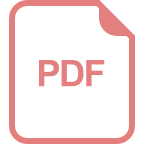
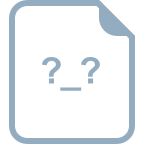
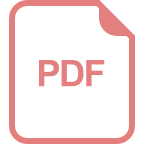