输入10个学生的三门课的成绩,分别用C语言实现函数求:1、每个学生的平均分2、按学 生平均分降序排列输出相关信息3、统计不及格学生,输出信息4、编写一菜单主函数,菜单 内容包括以上4部分内容
时间: 2024-12-15 21:21:08 浏览: 18
在C语言中,我们可以创建一个结构体表示学生的信息,然后编写几个函数来完成这些任务。这里是一个简单的例子:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义学生结构体
typedef struct {
char name[50];
float score1, score2, score3;
} Student;
// 求平均分
float calculate_average(Student* student) {
return (student->score1 + student->score2 + student->score3) / 3.0;
}
// 按平均分排序
void sort_students_by_average(Student students[], int count) {
// 使用冒泡排序或其他排序算法,此处简化只做逻辑描述
for (int i = 0; i < count - 1; j++) {
if (calculate_average(&students[j]) > calculate_average(&students[j + 1])) {
Student temp = students[j];
students[j] = students[j + 1];
students[j + 1] = temp;
}
}
}
}
// 统计不及格的学生
int count_failing_students(Student students[], int count) {
int failing = 0;
for (int i = 0; i < count; i++) {
if (students[i].score1 < 60 || students[i].score2 < 60 || students[i].score3 < 60) {
failing++;
}
}
return failing;
}
// 主菜单函数
void menu() {
Student students[10]; // 假设最多10名学生
int numStudents, failedCount;
printf("请输入学生信息(姓名和三门课程成绩,每行一位学生)\n");
scanf("%s %f %f %f", students[0].name, &students[0].score1, &students[0].score2, &students[0].score3);
numStudents = 1;
while (scanf("%s %f %f %f", students[numStudents].name, &students[numStudents].score1, &students[numStudents].score2, &students[numStudents].score3) == 4) {
numStudents++;
}
// 计算平均分和不及格人数
double average[numStudents];
for (int i = 0; i < numStudents; i++) {
average[i] = calculate_average(&students[i]);
}
failedCount = count_failing_students(students, numStudents);
// 输出结果
printf("\n平均分及学生信息(从高到低):\n");
sort_students_by_average(students, numStudents);
for (int i = 0; i < numStudents; i++) {
printf("%s: 平均分 %.2f\n", students[i].name, average[i]);
}
printf("\n不及格学生数: %d\n", failedCount);
}
int main() {
menu();
return 0;
}
```
阅读全文
相关推荐
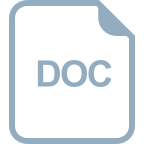
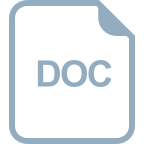
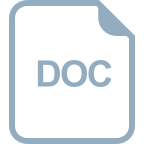
















