对一幅图像进行平移(向右、向下平移20个像素)、缩放(放大2倍)和旋转操作(旋转30°),将结果与原图像显示于同一窗口。
时间: 2024-10-05 09:03:21 浏览: 28
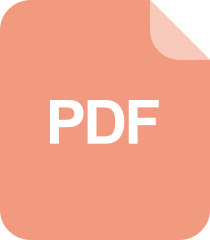
基于仿射变换模型的图像配准中的平移_旋转和缩放

在图像处理中,对一幅图像进行这样的操作通常需要使用专门的图像处理库,例如Python的PIL(Python Imaging Library)或者OpenCV。以下是步骤概述:
1. **导入所需库**:首先,你需要导入相关的库,如`PIL.Image` 或 `cv2`。
```python
from PIL import Image
```
或者
```python
import cv2
```
2. **读取原图**:使用`Image.open()` 或 `cv2.imread()`加载原始图片。
3. **创建副本**:为了不影响原始图像,我们先创建一个副本进行处理。
```python
image = image.copy() # 使用PIL
# or
image = image.clone() # 使用OpenCV
```
4. **平移**:在PIL中,可以使用`transpose()`函数配合切片操作来实现平移。而在OpenCV中,则是通过`shift()`。
```python
image = image.transpose(Image.ROTATE_90) # 假设你要向右下角平移20像素
# 或者
height, width = image.shape[:2]
image = image[height//2-20:height//2+20, width//2-20:width//2+20] # OpenCV
```
5. **缩放**:对于PIL,可以使用`thumbnail()`或`resize()`函数;OpenCV则有`resize()`。
```python
image = image.resize((image.width * 2, image.height * 2)) # 放大2倍
# 或
image = cv2.resize(image, None, fx=2, fy=2, interpolation=cv2.INTER_LINEAR) # OpenCV
```
6. **旋转**:使用`rotate()`(PIL)或`getRotationMatrix2D()` 和 ` warpAffine()`(OpenCV)进行旋转。
```python
rotated_image = image.rotate(30, expand=True) # PIL
# 或
M = cv2.getRotationMatrix2D((image.shape[1] // 2, image.shape[0] // 2), 30, 1)
rotated_image = cv2.warpAffine(image, M, (image.shape[1], image.shape[0])) # OpenCV
```
7. **显示原图和处理后的图**:最后,在同一个窗口显示原图和处理后的图像。
```python
# PIL
plt.subplot(1, 2, 1)
plt.imshow(image)
plt.subplot(1, 2, 2)
plt.imshow(rotated_image)
plt.show()
# OpenCV
cv2.imshow("Original", image)
cv2.imshow("Transformed", rotated_image)
cv2.waitKey(0) # 等待用户按键关闭
cv2.destroyAllWindows()
```
阅读全文
相关推荐
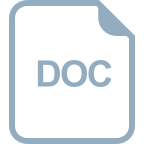
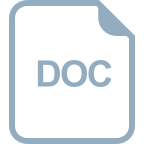

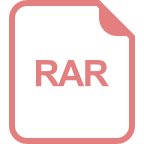
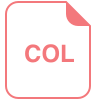
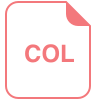
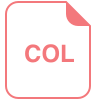
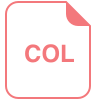
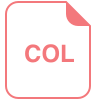
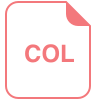
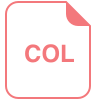
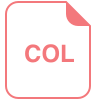
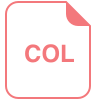
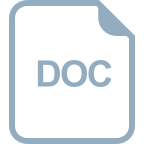
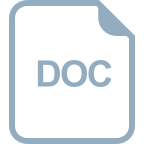
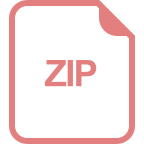
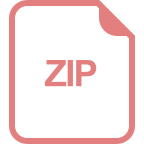