string删除指定字符串
时间: 2024-09-14 17:05:12 浏览: 45
在编程中,删除字符串中的指定字符串通常是指从一个字符串(称为原字符串)中移除所有出现的另一个字符串(称为子字符串)的实例。这个操作取决于具体的编程语言,但大多数语言都提供了这样的功能。
在C#中,可以使用`String.Replace`方法来实现这一功能。这个方法将返回一个新的字符串,其中所有的子字符串实例都被替换为空字符串(即删除操作)。例如:
```csharp
string originalString = "Hello World";
string toRemove = "World";
string newString = originalString.Replace(toRemove, "");
```
在这个例子中,`newString`将会是"Hello ",因为"World"被从原字符串中删除了。
在Python中,可以使用`str.replace`方法或者正则表达式的`re.sub`方法来实现类似的操作。`str.replace`方法的用法如下:
```python
original_string = "Hello World"
to_remove = "World"
new_string = original_string.replace(to_remove, "")
```
这段代码将返回"Hello ",同样实现了删除子字符串的操作。
相关问题
std::string 实现删除字符串中指定字符
可以使用 `std::string::erase()` 函数删除字符串中指定字符。该函数的参数是指定要删除的字符的位置和数量。可以使用 `std::string::find()` 函数在字符串中查找指定字符的位置,然后使用 `std::string::erase()` 函数删除该字符。以下是一个示例代码:
```c++
#include <iostream>
#include <string>
int main() {
std::string str = "hello world";
char ch = 'l';
std::size_t pos = str.find(ch);
while (pos != std::string::npos) {
str.erase(pos, 1);
pos = str.find(ch);
}
std::cout << str << std::endl;
return 0;
}
```
在上面的示例代码中,首先定义了一个字符串 `str` 和一个要删除的字符 `ch`。然后使用 `std::string::find()` 函数查找 `ch` 在 `str` 中的位置,并使用 `std::string::erase()` 函数删除该字符。由于 `std::string::find()` 函数返回的是 `std::string::npos`,表示未找到指定字符,因此在一个循环中执行删除操作,直到找不到指定字符为止。最后输出删除指定字符后的字符串。
std::string移除指定字符串
你可以使用标准库中的字符串操作函数来移除指定的字符串。下面是一个示例代码,展示如何使用std::string的erase和find函数来移除指定字符串:
```cpp
#include <iostream>
#include <string>
void removeSubstring(std::string& mainStr, const std::string& subStr) {
// 查找子字符串
size_t pos = mainStr.find(subStr);
if (pos != std::string::npos) {
// 删除子字符串
mainStr.erase(pos, subStr.length());
}
}
int main() {
std::string str = "Hello World!";
std::string subStr = "o";
removeSubstring(str, subStr);
std::cout << "Modified string: " << str << std::endl;
return 0;
}
```
在上面的示例中,定义了一个`removeSubstring`函数,它接受一个`std::string`的引用和一个要移除的子字符串。该函数首先使用`find`函数查找子字符串在主字符串中的位置,并将结果保存在`pos`变量中。如果找到了子字符串,则使用`erase`函数从主字符串中删除该子字符串。
在主函数中,我们使用示例字符串"Hello World!"和要移除的子字符串"o"进行测试。运行后,输出结果为"Modified string: Hell Wrld!",子字符串"o"已被成功移除。
希望这个示例能够帮助到你!如果有更多问题,请随时提问。
阅读全文
相关推荐

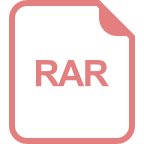














