该题目要求编写一个函数delchar,它可以删除给定字符串中的指定字符,例如字符串"abrtgt",删除\n字符后变为"abrtgt",主函数完成后调用delchar函数,将给定字符串变为"abdef",然后将指定字符串和指定字符雨输入,调用delchar函数,完成字符串的删除指定字符操作。
时间: 2024-02-25 18:52:26 浏览: 24
好的,这是一个字符串删除指定字符的问题。下面是一个可能的解决方案:
```python
def delchar(string, char):
"""
删除给定字符串中的指定字符。
:param string: 给定的字符串
:param char: 指定的字符
:return: 删除指定字符后的字符串
"""
new_string = ""
for c in string:
if c != char:
new_string += c
return new_string
```
这个函数接受两个参数:一个字符串和一个字符。它遍历字符串中的每个字符,如果字符不是指定字符,则将其添加到新字符串中。最后返回新字符串,其中指定字符已被删除。
您可以通过以下方式调用该函数来删除给定字符串中的指定字符:
```python
string = "abrtgt"
char = "\n"
new_string = delchar(string, char)
print(new_string) # 输出 "abrtgt"
```
如果要将指定字符串中的指定字符删除,您可以按照以下方式调用该函数:
```python
string = "abcdef"
char = "c"
new_string = delchar(string, char)
print(new_string) # 输出 "abdef"
```
希望这个解决方案可以帮到您!
相关问题
编写函数Delete()在字符串中删除给定的某字符。例如:在"hello“中删掉'l',结果为"heo". 然后在主函数中,输入字符串和待删除的字符,再调用函数Delete删除字符串中与该字符相同的字符,最后输出删除字符后的字符串。 程序运行结果示例: Input a string: hello, my friend!↙ Input a character: l↙ Results:heo, my friend! 输入格式: 输入字符串用 gets()函数 输入单个字符用 getchar()函数 输出格式: 输入字符串的提示信息: "Input a string:\n" 输入单个字符的提示信息: "Input a character:\n" 输出格式: "Results:%s\n"
C++代码如下:
```cpp
#include <iostream>
#include <cstring>
using namespace std;
const int MAXN = 100;
void Delete(char s[], char c) {
int len = strlen(s);
int j = 0;
for (int i = 0; i < len; i++) {
if (s[i] != c) {
s[j++] = s[i];
}
}
s[j] = '\0';
}
int main() {
char s[MAXN], c;
cout << "Input a string:" << endl;
gets(s);
cout << "Input a character:" << endl;
c = getchar();
Delete(s, c);
cout << "Results:" << s << endl;
return 0;
}
```
首先,定义一个Delete函数,用于在字符串中删除给定的某字符。具体实现是,遍历字符串,如果当前字符不等于给定的字符,则将其加入到新的字符串中,最后将新的字符串赋值回原字符串。
然后,在主函数中,读入字符串和待删除的字符,调用Delete函数删除字符串中与该字符相同的字符,最后输出删除后的字符串。注意,字符串的读入使用gets函数,单个字符的读入使用getchar函数。
c语言编写函数Delete()在字符串中删除给定的某字符。例如:在"hello“中删掉'l',结果为"heo". 然后在主函数中,输入字符串和待删除的字符,再调用函数Delete删除字符串中与该字符相同的字符,最后输出删除字符后的字符串。 程序运行结果示例: Input a string: hello, my friend!↙ Input a character: l↙ Results:heo, my friend! 输入格式: 输入字符串用 gets()函数 输入单个字符用 getchar()函数 输出格式: 输入字符串的提示信息: "Input a string:\n" 输入单个字符的提示信息: "Input a character:\n" 输出格式: "Results:%s\n"
C语言代码如下:
```c
#include <stdio.h>
#include <string.h>
#define MAXN 100
void Delete(char s[], char c) {
int len = strlen(s);
int j = 0;
for (int i = 0; i < len; i++) {
if (s[i] != c) {
s[j++] = s[i];
}
}
s[j] = '\0';
}
int main() {
char s[MAXN], c;
printf("Input a string:\n");
gets(s);
printf("Input a character:\n");
c = getchar();
Delete(s, c);
printf("Results:%s\n", s);
return 0;
}
```
这个程序与C++程序的思路基本一致。使用gets函数读入字符串,getchar函数读入待删除的字符,然后调用Delete函数删除字符串中与该字符相同的字符,最后输出删除后的字符串。需要注意的是,由于C语言中没有iostream头文件,所以输入输出要使用printf和scanf函数。
阅读全文
相关推荐





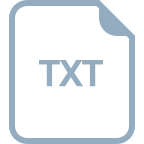







