rot13加密算法c语言
时间: 2023-12-15 16:33:23 浏览: 182
根据提供的引用内容,没有找到关于rot13加密算法的C语言实现。不过,rot13加密算法是一种简单的字母替换加密方法,可以用C语言实现。下面是一个简单的C语言实现rot13加密算法的例子:
```c
#include <stdio.h>
#include <ctype.h>
int main() {
char c;
while ((c = getchar()) != EOF) {
if (isalpha(c)) {
if (islower(c)) {
c = ((c - 'a') + 13) % 26 + 'a';
} else {
c = ((c - 'A') + 13) % 26 + 'A';
}
}
putchar(c);
}
return 0;
}
```
上述代码中,使用了getchar()函数从标准输入读取字符,使用putchar()函数将加密后的字符输出到标准输出。isalpha()函数用于判断字符是否为字母,islower()函数用于判断字符是否为小写字母,toupper()函数用于将字符转换为大写字母。加密算法的实现是将字母的ASCII码值加上13,然后对26取模,再加上字母A或a的ASCII码值,得到加密后的字母。
相关问题
c语言rot13加密算法
很抱歉,提供的引用内容中并没有C语言实现的Rot13加密算法。不过,Rot13加密算法的实现比较简单,可以用C语言轻松实现。下面是一个C语言实现的Rot13加密算法的例子:
```c
#include <stdio.h>
#include <string.h>
void rot13(char *input, char *output) {
int i;
for (i = 0; i < strlen(input); i++) {
char c = input[i];
if (c >= 'a' && c <= 'm') {
c += 13;
} else if (c >= 'A' && c <= 'M') {
c += 13;
} else if (c >= 'n' && c <= 'z') {
c -= 13;
} else if (c >= 'N' && c <= 'Z') {
c -= 13;
}
output[i] = c;
}
output[i] = '\0';
}
int main() {
char input[100], output[100];
printf("请输入要加密的字符串:");
scanf("%s", input);
rot13(input, output);
printf("加密后的字符串为:%s\n", output);
return 0;
}
```
rot13加密算法c
以下是使用C语言实现Rot13加密算法的代码示例:
```c
#include <stdio.h>
#include <ctype.h>
char rot13(char c) {
if (isalpha(c)) {
if ((c >= 'a' && c <= 'm') || (c >= 'A' && c <= 'M')) {
c += 13;
} else {
c -= 13;
}
}
return c;
}
void rot13_encrypt(char *str) {
while (*str) {
*str = rot13(*str);
str++;
}
}
void rot13_decrypt(char *str) {
rot13_encrypt(str);
}
int main() {
char str[100];
printf("请输入要加密的字符串:");
fgets(str, 100, stdin);
rot13_encrypt(str);
printf("加密后的字符串为:%s", str);
rot13_decrypt(str);
printf("解密后的字符串为:%s", str);
return 0;
}
```
阅读全文
相关推荐
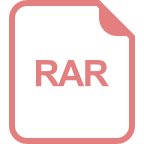
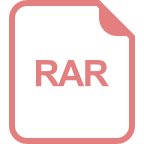
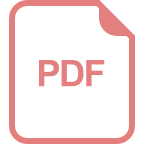
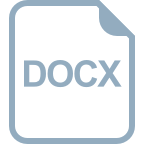
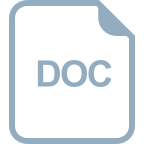
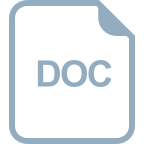
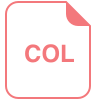


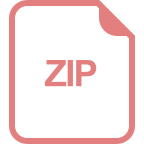
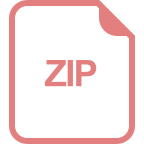
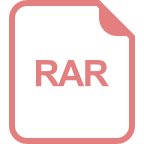
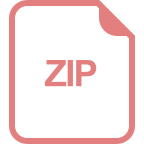