(x_train, y_train), (x_test, y_test) = mnist.load_data() x_train = x_train / 255. x_test = x_test / 255. inputs = Input(shape=(28, 28,)) x = Flatten()(inputs) y = Spectral(200, activation='relu', name='Spec1', use_bias=False)(x) y = Spectral(300, activation='relu', is_diag_start_trainable=True, use_bias=False, name='Spec2')(y) y = Spectral(300, activation='relu', name='Dense1')(y) x = Spectral(200, activation='relu', name='Spec3', use_bias=False)(x) x = Spectral(300, activation='relu', is_diag_start_trainable=True, use_bias=False, name='Spec4')(x) x = Spectral(300, activation='relu', name='Spec5')(x) z = Average()([x, y]) outputs = Dense(10, activation="softmax")(z) model = Model(inputs=inputs, outputs=outputs, name="branched") model.compile(optimizer=Adam(1E-3), loss=scc(from_logits=False), metrics=["accuracy"])
时间: 2024-04-16 15:25:11 浏览: 75
这段代码是一个基于Keras框架的神经网络模型,用于在MNIST数据集上进行训练和测试。
首先,使用mnist.load_data()函数加载MNIST数据集,并将训练集和测试集分别赋值给(x_train, y_train)和(x_test, y_test)。
然后,对输入数据进行归一化处理,将像素值从0-255缩放到0-1范围。通过除以255实现了这一步骤。
接下来,定义了一个输入层,形状为(28, 28)的张量。
然后,通过Flatten层将输入展平为一维向量。
接着,创建了两个隐藏层,使用了Spectral层,具有200个和300个神经元,并使用ReLU激活函数。第二个隐藏层还具有一个可训练的对角线起始谱权重,并且不使用偏置。
同时,在输入数据上也使用了两个Spectral层,具有200个和300个神经元,并使用ReLU激活函数。第二个层也具有一个可训练的对角线起始谱权重,并且不使用偏置。
然后,通过Average层将x和y进行平均。
最后,通过Dense层将平均值映射到具有10个输出节点的softmax层,用于多类别分类任务。
接着定义了模型的结构,并使用Adam优化器、交叉熵损失函数和准确率作为指标进行模型编译。
最终得到了一个名为"branched"的神经网络模型,可以用于训练和评估MNIST数据集。
相关问题
mnist = tf.keras.datasets.mnist (x_train, y_train), (x_test, y_test) = mnist.load_data() x_train, x_test = x_train / 255.0, x_test / 255.0无法实现,有什么原因呢
这段代码中的问题在于第二个赋值语句 `x_train, x_test = x_train / 255.0, x_test / 255.0`,其中的除法操作只对 `x_test` 生效,而对 `x_train` 没有生效。这是因为这里的逗号实际上是创建了一个元组,而右边的除法操作只对 `x_test` 有效。如果想对 `x_train` 和 `x_test` 都进行除法操作,可以改为如下代码:
```python
import tensorflow as tf
# 加载数据
mnist = tf.keras.datasets.mnist
(x_train, y_train), (x_test, y_test) = mnist.load_data()
x_train = x_train / 255.0
x_test = x_test / 255.0
# 其他代码
```
这样就可以对 `x_train` 和 `x_test` 都进行除法操作了。
(X_train,Y_train),(X_test,Y_test) = mnist.load_data()
This code uses the `load_data()` function from the `mnist` module to load the MNIST dataset. The dataset is split into training and testing sets, with the training set stored in `X_train` and `Y_train` variables, and the testing set stored in `X_test` and `Y_test` variables. `X_train` and `X_test` contain the images of handwritten digits, while `Y_train` and `Y_test` contain the corresponding labels for those images.
阅读全文
相关推荐
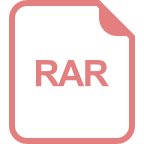
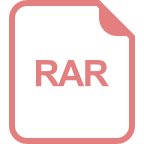
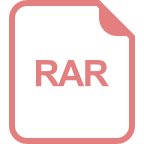













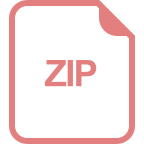