给定n个单词,请将每个单词的字母填充到一个7行若干列的矩阵中。字母按输 入顺序逐列从上往下、从左往右填充矩阵。如果最后一列不足七个字符,用*补 足。c++
时间: 2024-10-28 16:08:27 浏览: 17
在C++中,你可以使用二维数组或者动态分配的空间来实现这个功能。这里是一个简单的示例代码,它假设所有单词的长度都不超过6个字符(包括空格),并且总字符数不超过49:
```cpp
#include <iostream>
#include <vector>
std::string fillMatrix(const std::vector<std::string>& words) {
int totalChars = 0;
for (const auto& word : words) {
totalChars += word.size();
}
int rows = 7, cols = ceil(totalChars / rows);
std::vector<std::vector<char>> matrix(rows, std::vector<char>(cols));
int index = 0;
for (auto& row : matrix) {
for (int i = 0; i < cols && index < words.size(); ++i) {
row[i] = words[index][i];
if (index + 1 == words.size() || i + 1 == cols) { // 如果达到一行结束或一列结束
while (row[i] != '\0') { // 将剩余的字符替换为*
row[i++] = '*';
}
} else {
++index;
}
}
}
return std::string(matrix.begin(), matrix.end());
}
int main() {
std::vector<std::string> words = {"hello", "world", "this", "is", "a", "test"};
std::string result = fillMatrix(words);
std::cout << result << std::endl;
return 0;
}
```
这个函数首先计算所有单词的总字符数,然后确定矩阵的大小。接着遍历每个单词,按照指定规则填充矩阵。当遇到单词结束或列满时,会用星号`*`填充剩余位置。
注意:实际应用中,如果单词长度可能会超过6个字符,你需要预先处理或者让用户保证输入的合理长度。另外,此代码不包含错误检查,例如空指针异常等,根据实际情况添加相应的错误处理。
阅读全文
相关推荐
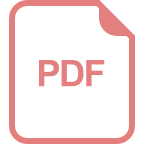
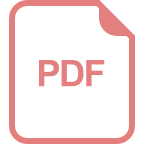
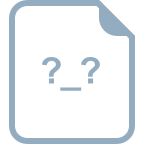















