pytorch建立卷积网络
时间: 2024-03-20 08:38:10 浏览: 22
PyTorch是一个开源的深度学习框架,可以用于构建卷积神经网络(Convolutional Neural Network, CNN)。下面是使用PyTorch建立卷积网络的一般步骤:
1. 导入必要的库和模块:
```python
import torch
import torch.nn as nn
import torch.optim as optim
```
2. 定义卷积神经网络的结构:
```python
class ConvNet(nn.Module):
def __init__(self):
super(ConvNet, self).__init__()
self.conv1 = nn.Conv2d(in_channels, out_channels, kernel_size)
self.relu = nn.ReLU()
self.pool = nn.MaxPool2d(kernel_size)
self.fc = nn.Linear(in_features, out_features)
def forward(self, x):
x = self.conv1(x)
x = self.relu(x)
x = self.pool(x)
x = x.view(x.size(0), -1)
x = self.fc(x)
return x
```
在这个例子中,我们定义了一个简单的卷积神经网络,包含一个卷积层、ReLU激活函数、池化层和全连接层。
3. 实例化网络模型:
```python
model = ConvNet()
```
4. 定义损失函数和优化器:
```python
criterion = nn.CrossEntropyLoss()
optimizer = optim.SGD(model.parameters(), lr=learning_rate)
```
这里使用交叉熵损失函数和随机梯度下降(SGD)优化器。
5. 训练网络:
```python
for epoch in range(num_epochs):
for images, labels in train_loader:
optimizer.zero_grad()
outputs = model(images)
loss = criterion(outputs, labels)
loss.backward()
optimizer.step()
```
这里使用一个双层循环,外层循环控制训练的轮数,内层循环遍历训练数据集,计算损失并进行反向传播和参数更新。
6. 测试网络:
```python
with torch.no_grad():
correct = 0
total = 0
for images, labels in test_loader:
outputs = model(images)
_, predicted = torch.max(outputs.data, 1)
total += labels.size(0)
correct += (predicted == labels).sum().item()
accuracy = 100 * correct / total
print('Test Accuracy: {}%'.format(accuracy))
```
这里使用测试数据集对训练好的网络进行评估,并计算准确率。
相关推荐
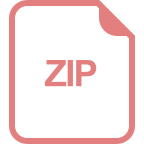
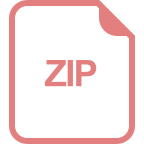














