对于给定的 n 个元素的数组a[0:n-1],要求从中找出第 k 小的元素。写出一个C语言程序
时间: 2024-10-18 18:06:49 浏览: 44
为了在C语言中找到给定数组`a[0:n-1]`中的第k小元素,你可以使用一种叫做“快速选择”(QuickSelect)的算法,它是一种基于分治策略的选择算法。这是一种平均时间复杂度为O(n)的解决方案,但在最坏的情况下为O(n^2)。下面是简化版的C语言程序:
```c
#include <stdio.h>
#include <stdlib.h>
// 这里假设数组已经排序
int partition(int a[], int left, int right, int pivotIndex) {
int pivot = a[pivotIndex];
int i = (left - 1); // Index of smaller element
for (int j = left; j <= right; j++) {
if (a[j] < pivot) {
i++;
swap(&a[i], &a[j]); // Swap elements
}
}
swap(&a[i + 1], &a[pivotIndex]);
return (i + 1);
}
// QuickSelect function
int quickSelect(int a[], int left, int right, int k) {
if (left == right) {
return a[left];
}
int pivotIndex = rand() % (right - left + 1) + left; // Random pivot index
pivotIndex = partition(a, left, right, pivotIndex);
if (k == pivotIndex) {
return a[k];
} else if (k < pivotIndex) {
return quickSelect(a, left, pivotIndex - 1, k);
} else {
return quickSelect(a, pivotIndex + 1, right, k);
}
}
// 主函数
int main() {
int n;
printf("Enter the number of elements in the array: ");
scanf("%d", &n);
int a[n]; // Declare and input your array elements here
printf("Enter the array elements: ");
for (int i = 0; i < n; i++) {
scanf("%d", &a[i]);
}
int k;
printf("Enter the value of k: ");
scanf("%d", &k);
if (k >= 0 && k < n) {
int kthSmallestElement = quickSelect(a, 0, n - 1, k - 1); // Subtract 1 from k because arrays are 0-indexed
printf("The k-th smallest element is: %d\n", kthSmallestElement);
} else {
printf("Invalid value of k.\n");
}
return 0;
}
```
阅读全文
相关推荐
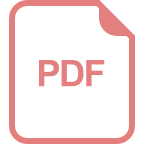
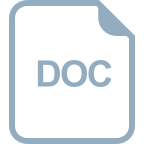
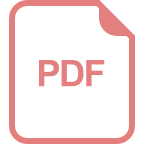





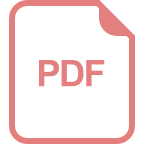
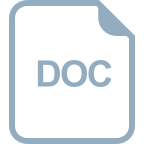
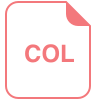
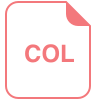
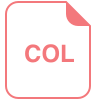
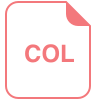
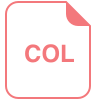




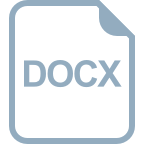