编写一个java程序,在程序中定义一个Employee类,Employee类有四个构造方法,分别对不同的属性进行初始化,有一个setAge()方法能设置age属性值,有一个getAge()方法能返回age属性值
时间: 2024-10-27 20:03:21 浏览: 28
在Java中,我们可以创建一个名为`Employee`的类,它包含四个构造函数以便于初始化不同的属性,比如姓名、工号和初始年龄。同时,我们将添加一个`setAge()`方法用于设置员工的年龄,以及一个`getAge()`方法来获取这个年龄。以下是详细的类定义:
```java
public class Employee {
private String name;
private int id;
private int age;
// 构造方法1:仅初始化默认年龄
public Employee(String name, int id) {
this.name = name;
this.id = id;
this.age = 0; // 默认年龄
}
// 构造方法2:指定年龄
public Employee(String name, int id, int initialAge) {
this.name = name;
this.id = id;
this.age = initialAge;
}
// 构造方法3:仅初始化姓名和工号
public Employee(String name, int idWithoutAge) {
this.name = name;
this.id = idWithoutAge;
this.age = -1; // 表示年龄未提供
}
// 构造方法4:所有属性都指定
public Employee(String name, int id, int initialAgeWithoutDefault) {
this.name = name;
this.id = id;
this.age = initialAgeWithoutDefault;
}
// setAge方法
public void setAge(int newAge) {
this.age = newAge;
}
// getAge方法
public int getAge() {
return age;
}
// 示例
public static void main(String[] args) {
Employee e1 = new Employee("张三", 1001);
e1.setAge(30);
System.out.println("员工年龄: " + e1.getAge());
Employee e2 = new Employee("李四", 2002, 25);
System.out.println("员工李四年龄: " + e2.getAge());
}
}
```
在这个例子中,`Employee`类展示了如何通过构造函数和成员方法支持多种情况下的年龄设置。在`main`方法中,我们演示了如何根据需要创建并操作不同的`Employee`对象。
阅读全文
相关推荐
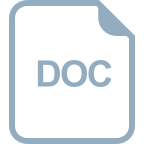
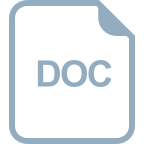
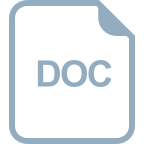

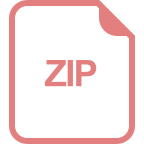
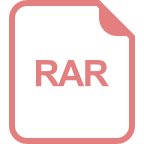
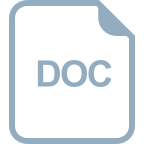
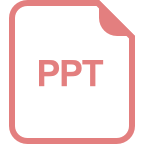
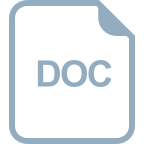
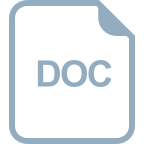
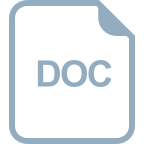
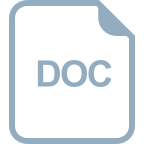
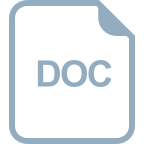
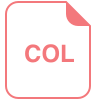





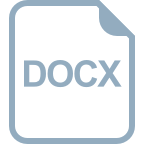