for ( ; ; ) { /* 循环读取数据 */ if (sizeof(struct input_event) != read(fd, &in_ev, sizeof(struct input_event))) { perror("read error"); exit(-1); }
时间: 2024-04-15 14:25:26 浏览: 203
这段代码是一个无限循环,用于持续地从文件描述符 fd 中读取 input_event 结构体的数据。在每次循环中,它使用 read 函数从文件中读取一个 input_event 结构体的大小(sizeof(struct input_event))的数据,并将结果存储在 in_ev 变量中。
如果读取操作失败(返回的字节数不等于 input_event 结构体的大小),则会输出错误信息并退出程序并返回-1。这样做是为了确保数据能够正确地被读取,并处理读取错误的情况。
相关问题
int t_x, t_y; // 按下的坐标 while (1) { // 2.读取触摸屏信息 struct input_event xy; read(fd, &xy, sizeof(xy)); // 判断是否为触摸屏的X 轴事件 if (xy.type == EV_ABS && xy.code == ABS_X) { // 转换坐标 x = xy.value * 800 / 1024; *gx = x; // 输出X轴的坐标值 printf("x=%d\n", x); } // 判断是否为触摸屏的X 轴事件 if (xy.type == EV_ABS && xy.code == ABS_Y) { // 转换坐标 y = xy.value * 480 / 600; *gy = y; // 输出X轴的坐标值 printf("y=%d\n", y); } // 获取按钮是否按下的状态 if (xy.type == EV_KEY && xy.code == BTN_TOUCH) { printf("touch=%d\n", xy.value); if (xy.value == 1) // 按下 { // 保存按下的坐标 t_x = x; t_y = y; } if (xy.value == 0) // 松开 { break; // 跳出循环 } } }
这是一段 C 代码,可能是在 Linux 嵌入式系统上针对触摸屏进行的编程。通过读取触摸屏事件,将触摸屏坐标转换成屏幕坐标,并保存按下的坐标和松开的坐标。其中 EV_ABS 和 ABS_X 表示 X 轴方向上的事件,EV_ABS 和 ABS_Y 表示 Y 轴方向上的事件,xy.value 表示事件的值。如果检测到按钮按下和松开的状态,就记录相应的坐标,并跳出循环。这段代码可能被用于开发嵌入式系统上的触摸屏应用程序。你还有其他问题吗?
#include <stdio.h> #include <fcntl.h> #include <linux/input.h> int main() { int fd; struct input_event event; fd = open("/dev/input/event0", O_RDWR); if (fd < 0) { perror("open"); return -1; } // 获取输入事件设备信息 if (ioctl(fd, EVIOCGNAME(sizeof("event0")), "event0") < 0) { perror("ioctl"); return -1; } // 读取输入事件 while (read(fd, &event, sizeof(event)) > 0) { if (event.type == EV_KEY && event.code == KEY_F1) { // 禁用F1键 event.value = 0; if (write(fd, &event, sizeof(event)) < 0) { perror("write"); return -1; } } } close(fd); return 0; } 没有禁用成功
可能的原因是你使用的键盘输入设备文件不是/dev/input/event0,可以尝试使用以下命令查看当前系统中可用的输入设备:
```
$ cat /proc/bus/input/devices
```
输出内容类似于:
```
I: Bus=0011 Vendor=0001 Product=0001 Version=ab41
N: Name="AT Translated Set 2 keyboard"
P: Phys=isa0060/serio0/input0
S: Sysfs=/devices/platform/i8042/serio0/input/input0
U: Uniq=
H: Handlers=sysrq kbd event0
B: PROP=0
B: EV=120013
B: KEY=4 2000000 3803078 f800d001 feffffdf ffefffff ffffffff fffffffe
B: MSC=10
B: LED=7
I: Bus=0003 Vendor=046d Product=c52b Version=0111
N: Name="Logitech USB Receiver"
P: Phys=usb-0000:03:00.0-1.1/input0
S: Sysfs=/devices/pci0000:03/0000:03:00.0/usb2/2-1/2-1.1/2-1.1:1.0/0003:046D:C52B.0001/input/input2
U: Uniq=
H: Handlers=sysrq kbd event1
B: PROP=0
B: EV=1b
B: KEY=ffff0000 0 0 0 0 0 0 0 0 0 0 0 0 ffff 0 0 0 0 0 0 0 0 0 0 0 0
B: ABS=3
B: MSC=10
...
```
其中Handlers中包含了当前输入设备可用的事件接口列表。例如,上面的输出中,第一个输入设备AT Translated Set 2 keyboard可用的事件接口是sysrq、kbd和event0,可以尝试使用event0作为输入事件设备文件来禁用键盘按键。
另外,为了避免程序一直在循环读取输入事件,可以在程序中添加一个休眠时间,例如:
```
#include <unistd.h>
...
// 读取输入事件
while (1) {
if (read(fd, &event, sizeof(event)) > 0) {
if (event.type == EV_KEY && event.code == KEY_F1) {
// 禁用F1键
event.value = 0;
if (write(fd, &event, sizeof(event)) < 0) {
perror("write");
return -1;
}
}
}
usleep(1000); // 等待1毫秒
}
```
这样程序会每隔1毫秒读取一次输入事件,避免了不必要的CPU占用。
阅读全文
相关推荐
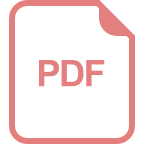
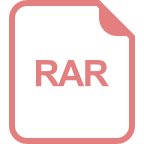
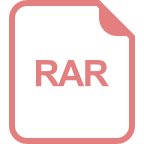
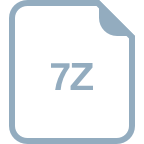
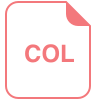











