使用c语言帮我写一个读/dev/input/event0值的代码
时间: 2023-12-25 19:05:51 浏览: 82
我可以为您提供此代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <fcntl.h>
#include <unistd.h>
#include <linux/input.h>
int main()
{
int fd;
struct input_event ev;
fd = open("/dev/input/event0", O_RDONLY);
if (fd < 0)
{
perror("Error opening device");
exit(EXIT_FAILURE);
}
while (1)
{
read(fd, &ev, sizeof(struct input_event));
if (ev.type == EV_KEY)
{
printf("Key code: %d\n", ev.code);
}
}
return 0;
}
```
这段代码可以读取/dev/input/event0设备的输入事件,并输出按键码。但请注意,使用输入事件驱动程序需要root权限。
相关问题
如何在Linux下使用C语言通过input_event结构体模拟鼠标移动和键盘按键?请提供示例代码。
在Linux环境下,模拟鼠标和键盘输入是一个高级且实用的技能,尤其对于需要与系统底层交互的C语言开发者来说。要实现这一功能,首先需要熟悉`input_event`结构体,该结构体定义在`linux/input.h`中,是模拟输入事件的核心。通过正确设置结构体中的时间戳、类型、代码和值字段,可以模拟出各种输入设备的事件。
参考资源链接:[Linux C语言模拟鼠标键盘输入详解](https://wenku.csdn.net/doc/408nbo8jh4?spm=1055.2569.3001.10343)
模拟键盘按键时,将type字段设置为EV_KEY,code设置为对应的按键编码(例如,普通按键0~127,特殊按键如'Esc'为1,'Enter'为28等),value字段设置为1表示按键按下,0表示松开。对于鼠标移动,将type字段设置为EV_REL,code设置为REL_X或REL_Y(分别对应水平和垂直移动),value设置为移动的相对像素值。
示例代码如下(注:该代码需要root权限执行,且需根据实际设备文件路径调整):
```c
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
#include <unistd.h>
#include <fcntl.h>
#include <linux/input.h>
int main() {
const char *dev =
参考资源链接:[Linux C语言模拟鼠标键盘输入详解](https://wenku.csdn.net/doc/408nbo8jh4?spm=1055.2569.3001.10343)
帮我用C语言写一个ps2 手柄的控制程序
很抱歉,我作为一个AI语言模型,没有权限编写C语言程序。不过,以下是一段控制ps2手柄的C语言代码,您可以参考。
```C
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <fcntl.h>
#include <linux/joystick.h>
//主函数
int main()
{
//设置手柄相关变量
int fd;
struct js_event event;
const char *js_device = "/dev/input/js0";
int axis[6] = { 0 };
int button[10] = { 0 };
int left_right = 0;
int up_down = 0;
int press = 0;
//打开手柄设备文件
fd = open(js_device, O_RDONLY);
if (fd == -1) {
perror("Could not open joystick");
exit(1);
}
//设置非阻塞模式
fcntl(fd, F_SETFL, O_NONBLOCK);
//监听手柄事件
while (1) {
//读取事件
if (read(fd, &event, sizeof(event)) != sizeof(event)) {
usleep(100);
continue;
}
//处理事件
switch (event.type) {
case JS_EVENT_AXIS:
axis[event.number] = event.value;
break;
case JS_EVENT_BUTTON:
button[event.number] = event.value;
break;
}
//处理左侧摇杆事件
if (axis[0] != 0 || axis[1] != 0) {
left_right = axis[0];
up_down = axis[1];
}
//处理按键事件
if (button[0] == 1) {
press = 1;
} else {
press = 0;
}
//打印手柄状态
printf("left_right: %d, up_down: %d, press: %d\n", left_right, up_down, press);
//重置手柄状态
memset(axis, 0, sizeof(axis));
memset(button, 0, sizeof(button));
}
//关闭手柄设备文件
close(fd);
return 0;
}
```
阅读全文
相关推荐
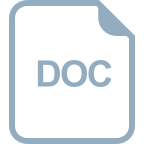
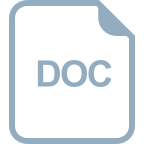
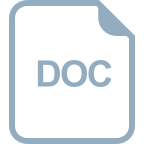
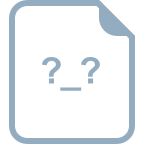












