数据结构课程设计银行业务模拟系统
时间: 2024-06-14 12:04:20 浏览: 164
银行业务模拟系统是一个常见的数据结构课程设计项目,它模拟了银行的基本业务流程,包括开户、存款、取款、转账等操作。下面是一个简单的银行业务模拟系统的示例:
```python
class Bank:
def __init__(self):
self.customers = {}
def add_customer(self, customer):
self.customers[customer.account_number] = customer
def remove_customer(self, account_number):
del self.customers[account_number]
def deposit(self, account_number, amount):
if account_number in self.customers:
self.customers[account_number].balance += amount
def withdraw(self, account_number, amount):
if account_number in self.customers:
if self.customers[account_number].balance >= amount:
self.customers[account_number].balance -= amount
else:
print("Insufficient balance")
def transfer(self, from_account, to_account, amount):
if from_account in self.customers and to_account in self.customers:
if self.customers[from_account].balance >= amount:
self.customers[from_account].balance -= amount
self.customers[to_account].balance += amount
else:
print("Insufficient balance")
class Customer:
def __init__(self, name, account_number, balance=0):
self.name = name
self.account_number = account_number
self.balance = balance
# 创建银行对象
bank = Bank()
# 添加客户
customer1 = Customer("Alice", "12345678", 1000)
customer2 = Customer("Bob", "87654321", 500)
bank.add_customer(customer1)
bank.add_customer(customer2)
# 存款
bank.deposit("12345678", 200)
# 取款
bank.withdraw("87654321", 100)
# 转账
bank.transfer("12345678", "87654321", 300)
# 输出客户信息
for account_number, customer in bank.customers.items():
print(f"Account Number: {account_number}, Name: {customer.name}, Balance: {customer.balance}")
```
这个示例中,我们创建了一个Bank类和一个Customer类。Bank类包含了添加客户、删除客户、存款、取款和转账等方法。Customer类表示银行的客户,包含了姓名、账号和余额等属性。
你可以根据需要扩展这个示例,添加更多的功能和业务逻辑。例如,可以添加查询余额、查询交易记录等方法。
阅读全文
相关推荐
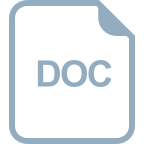
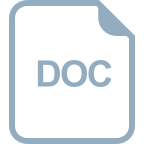
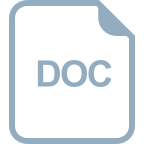
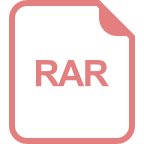
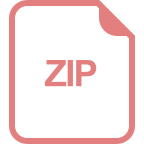
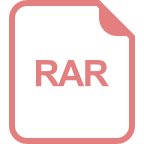
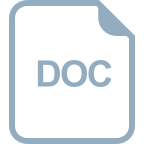
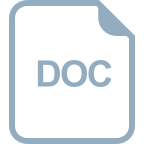
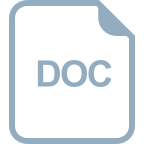
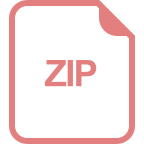
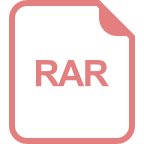
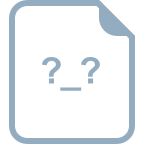
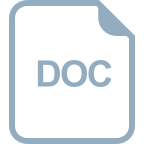
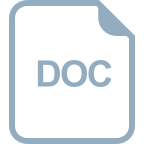
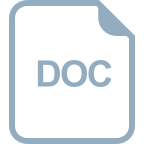
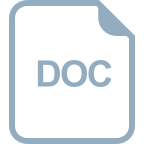
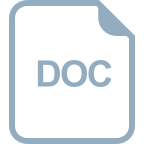
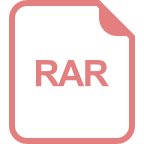
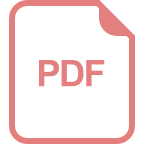