Python遗传算法求解函数最小值
时间: 2024-12-23 09:17:08 浏览: 2
Python中的遗传算法是一种模拟自然选择过程的优化技术,通常用于解决复杂的优化问题,包括寻找函数的最小值。遗传算法的基本步骤如下:
1. 初始化种群:随机生成一组解作为初始的“个体”,这些个体可以看作是候选的最优解。
2. 适应度评估:计算每个个体对应目标函数的值,称为适应度。适应度越高,表示个体越接近解。
3. 选择操作:根据每个个体的适应度进行选择,一般使用概率选择策略,如轮盘赌选择法,优秀的个体有更大的概率被保留。
4. 变异操作:对选中的个体进行变异,这可能导致新的解决方案。变异操作可能会改变个体的一些属性,增加多样性。
5. 交叉操作:通过交换两个个体的部分基因(即解的某些部分),产生新的后代个体。
6. 重复步骤3到5直到达到一定的迭代次数或找到满足条件的解。
为了求解函数最小值,你可以使用Python的`deap`库,它提供了高级的工具来实现遗传算法。下面是一个简单的示例:
```python
from deap import base, creator, tools
# 假设我们有一个函数f(x) = x^2 - 10*x + 15
creator.create("FitnessMin", base.Fitness, weights=(-1.0,))
creator.create("Individual", list, fitness=creator.FitnessMin)
def evalOneMax(individual):
return (-individual[0],), # 函数的负值作为适应度,因为我们要找的是最小值
toolbox = base.Toolbox()
toolbox.register("attr_float", random.random)
toolbox.register("individual", tools.initRepeat, creator.Individual, toolbox.attr_float, n=1)
toolbox.register("population", tools.initRepeat, list, toolbox.individual)
toolbox.register("evaluate", evalOneMax)
toolbox.register("mate", tools.cxTwoPoint)
toolbox.register("mutate", tools.mutFlipBit, indpb=0.05)
toolbox.register("select", tools.selTournament, tournsize=3)
pop = toolbox.population(n=50)
for gen in range(50): # 迭代次数
offspring = toolbox.select(pop, len(pop))
offspring = [toolbox.clone(ind) for ind in offspring]
for child1, child2 in zip(offspring[::2], offspring[1::2]):
if random.random() < 0.5:
toolbox.mate(child1, child2)
del child1.fitness.values
del child2.fitness.values
for mutant in offspring:
if random.random() < 0.2:
toolbox.mutate(mutant)
del mutant.fitness.values
invalid_ind = [ind for ind in offspring if not ind.fitness.valid]
fitnesses = toolbox.map(toolbox.evaluate, invalid_ind)
for ind, fit in zip(invalid_ind, fitnesses):
ind.fitness.values = fit
pop[:] = offspring
best_solution = toolbox.bestSolution(pop)
```
这个例子展示了一个基本的单变量函数最小化遗传算法。运行完后,`best_solution`就是最接近函数最小值的解。
阅读全文
相关推荐
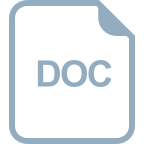
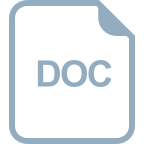
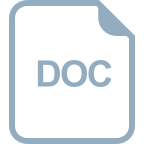






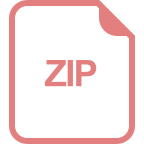
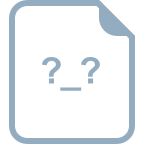
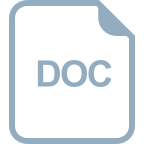
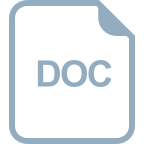



