pyside6 opencv
时间: 2023-10-23 19:08:39 浏览: 71
Python有很多图像处理库可以使用,以下是一些常用的库:
1. Pillow:Pillow是Python Imaging Library(PIL)的一个分支,是Python中最常用的图像处理库之一。提供了丰富的图像处理功能,包括图像打开、保存、调整大小、裁剪、旋转、滤镜等。
2. OpenCV:OpenCV是一个开源的计算机视觉库,可以用于图像和视频处理。它支持多种图像处理和计算机视觉算法,包括特征检测、物体识别、人脸识别等。
3. scikit-image:scikit-image是一个基于NumPy的图像处理库,提供了一系列简单易用的图像处理函数和工具。它支持图像的滤波、边缘检测、形态学操作、颜色空间转换等。
4. NumPy:NumPy是一个Python科学计算库,提供了高效的多维数组操作功能。可以使用NumPy来处理图像数据,进行像素级别的操作和计算。
这些都是非常强大和常用的图像处理库,你可以根据具体需求选择适合自己的库进行图像处理。
相关问题
pyside6 label旋转
在PySide6中,Label组件并不直接支持旋转功能,因为PySide6主要是用于构建图形用户界面(GUI),而非进行复杂的图像处理。如果你想要在一个PySide6应用中显示并旋转标签,通常需要借助额外的图像处理库,如`PIL`(Pillow)或`OpenCV`来先对图片进行旋转,然后再显示在Label上。
然而,如果你是在设计一个允许用户交互并调整Label显示内容(比如图片)旋转的应用,可以使用PySide6的`QGraphicsView`和`QGraphicsProxyWidget`配合`QGraphicsRotation`来实现。以下是一个简单的概念示例:
```python
from PySide6.QtWidgets import QApplication, QLabel, QGraphicsScene, QGraphicsView, QGraphicsProxyWidget, QGraphicsRotation
from PySide6.QtGui import QPixmap, QImage, QPainter
class RotateLabel(QGraphicsView):
def __init__(self, parent=None):
super().__init__(parent)
self.scene = QGraphicsScene()
self.view_widget = QLabel(self)
self.proxy = QGraphicsProxyWidget(self.view_widget)
self.proxy.setWidget(self.view_widget)
self.scene.addItem(self.proxy)
self.rotation_angle = 0
def set_image(self, pixmap):
rotated_pixmap = pixmap.scaledToWidth(self.view_widget.width())
rotated_pixmap = rotated_pixmap.transformed(QGraphicsRotation(self.rotation_angle, QPointF(0, 0)))
painter = QPainter(rotated_pixmap)
painter.drawPixmap(0, 0, pixmap)
self.scene.setSceneRect(rotated_pixmap.boundingRect())
def set_rotation(self, angle):
self.rotation_angle = angle
self.update()
# 使用示例
app = QApplication([])
label = RotateLabel()
label.set_image(QPixmap('path_to_your_image.jpg')) # 替换为实际图片路径
label.set_rotation(45) # 设置初始旋转角度
label.show()
app.exec_()
```
在这个例子中,`RotateLabel`类创建了一个可以旋转的QLabel。通过`set_image`方法,你可以设置一个图片,并通过`set_rotation`方法改变其旋转角度。
怎么将opencv视频流和PySide6结合
要将OpenCV视频流与PySide6结合,可以使用以下步骤:
1. 使用OpenCV捕获视频流
```python
import cv2
cap = cv2.VideoCapture(0) # 0表示默认摄像头,也可以使用视频文件路径
```
2. 创建PySide6窗口,并在其中显示视频流
```python
import sys
from PySide6.QtGui import QImage, QPixmap
from PySide6.QtWidgets import QApplication, QLabel
app = QApplication(sys.argv)
label = QLabel()
label.show()
while True:
ret, frame = cap.read() # 读取视频帧
if ret:
# 将OpenCV图像转换为PySide6图像
image = QImage(frame, frame.shape[1], frame.shape[0], QImage.Format_RGB888).rgbSwapped()
pixmap = QPixmap.fromImage(image)
# 在label中显示图像
label.setPixmap(pixmap)
# 处理PySide6事件,以确保窗口响应
app.processEvents()
```
3. 在程序结束时释放资源
```python
cap.release()
cv2.destroyAllWindows()
```
完整代码示例:
```python
import sys
import cv2
from PySide6.QtGui import QImage, QPixmap
from PySide6.QtWidgets import QApplication, QLabel
cap = cv2.VideoCapture(0)
app = QApplication(sys.argv)
label = QLabel()
label.show()
while True:
ret, frame = cap.read()
if ret:
image = QImage(frame, frame.shape[1], frame.shape[0], QImage.Format_RGB888).rgbSwapped()
pixmap = QPixmap.fromImage(image)
label.setPixmap(pixmap)
app.processEvents()
cap.release()
cv2.destroyAllWindows()
```
相关推荐
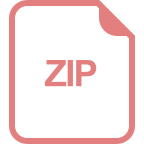
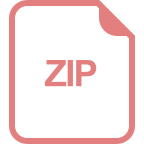
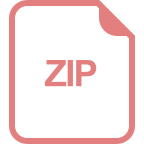












