用 fork( )创建一个进程,再调用 exec( )用新的程序替换该子进程的内容,利用 wait( )来控制进程执行顺序,并分析其结果。
时间: 2024-12-16 21:15:35 浏览: 10
在C语言中,你可以使用`fork()`创建一个新的进程,然后使用`exec()`函数来替换这个新进程的命令解释器,从而执行全新的程序。这通常用于动态加载和运行新的程序,而无需终止原进程。下面是一个简单的例子:
```c
#include <stdio.h>
#include <unistd.h>
#include <sys/wait.h>
#include <stdlib.h>
int main() {
pid_t pid;
const char* program[] = {"ls", "-l", NULL}; // 要执行的命令
// 创建子进程
if ((pid = fork()) == -1) {
perror("Failed to fork");
return 1;
} else if (pid == 0) {
// 这是子进程
execvp(program[0], program); // 执行新程序
perror("Failed to execvp");
return 1;
} else {
// 父进程
printf("Parent process ID is %d\n", getpid());
// 使用wait()等待子进程结束
int status;
wait(&status);
printf("Child process with PID %d finished with status %d\n", pid, WEXITSTATUS(status));
}
return 0;
}
```
在这个程序中,`fork()`创建了一个新的子进程,然后在子进程中,`execvp()`会替换当前的进程上下文,使得子进程开始执行 `ls -l` 命令,列出目录内容。父进程则继续执行,等待子进程结束后,通过`wait()`获取子进程的状态并打印出来。
运行这个程序,你会看到类似这样的输出:
```
Parent process ID is [your_pid]
Child process with PID [child_pid] finished with status [exit_status]
```
这里,`exit_status`将根据子进程的实际退出状态有所不同(通常是0表示成功,非零值表示错误)。
阅读全文
相关推荐
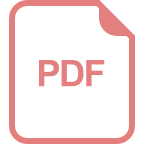
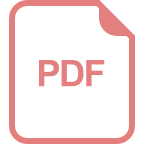
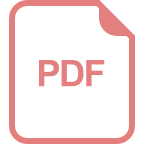















