基于Python,如果数据的采样时间间隔为15min,如何利用Sequential构建BP神经网络进行提前4hour预测,并用RMSE评估其结果代码
时间: 2024-04-10 19:34:36 浏览: 77
您可以使用Python中的numpy、tensorflow和sklearn库来实现基于Sequential模型的BP神经网络进行提前4小时预测,并使用RMSE评估结果。以下是一个示例代码:
```python
import numpy as np
import tensorflow as tf
from sklearn.metrics import mean_squared_error
# 假设您已经有了采样数据和相应的目标值
# 假设数据变量名为X,目标值变量名为y
# 将数据和目标值分为训练集和测试集,通常我们将大部分数据用于训练,少部分用于测试
train_size = int(len(X) * 0.8)
X_train, X_test = X[:train_size], X[train_size:]
y_train, y_test = y[:train_size], y[train_size:]
# 标准化数据,将数据缩放到0-1之间,提高模型的收敛速度和准确性
X_train = (X_train - np.min(X_train)) / (np.max(X_train) - np.min(X_train))
y_train = (y_train - np.min(y_train)) / (np.max(y_train) - np.min(y_train))
X_test = (X_test - np.min(X_test)) / (np.max(X_test) - np.min(X_test))
y_test = (y_test - np.min(y_test)) / (np.max(y_test) - np.min(y_test))
# 构建Sequential模型
model = tf.keras.Sequential([
tf.keras.layers.Dense(100, activation='relu', input_shape=(X_train.shape[1],)),
tf.keras.layers.Dense(100, activation='relu'),
tf.keras.layers.Dense(1)
])
# 编译模型
model.compile(optimizer='adam', loss='mse')
# 训练模型
model.fit(X_train, y_train, epochs=100, batch_size=32, verbose=0)
# 预测
y_pred = model.predict(X_test)
# 将预测结果反标准化,还原到原始范围
y_pred = y_pred * (np.max(y) - np.min(y)) + np.min(y)
y_test = y_test * (np.max(y) - np.min(y)) + np.min(y)
# 计算RMSE
rmse = np.sqrt(mean_squared_error(y_test, y_pred))
print("RMSE:", rmse)
```
请注意,代码中的参数(如隐藏层大小、激活函数、优化器、批次大小等)是示例值,您可以根据实际情况进行调整。此外,还需要根据实际数据的格式和特征工程进行适当的调整。
阅读全文
相关推荐



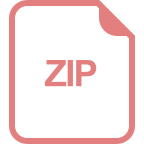
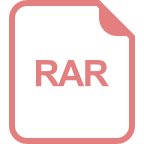
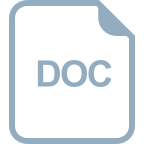









