python多线程写入同一个txt文件
时间: 2023-10-14 09:04:36 浏览: 226
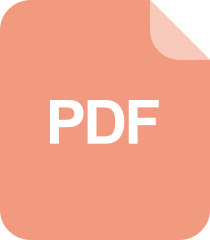
python多线程同步之文件读写控制
在 Python 中,你可以使用 `threading` 模块来实现多线程写入同一个 txt 文件。下面是一个示例代码:
```python
import threading
# 共享的文件对象
file_lock = threading.Lock()
file = open("example.txt", "a")
# 线程函数
def write_to_file(thread_name):
global file
global file_lock
for i in range(10):
with file_lock:
file.write(f"Thread {thread_name}: Line {i}\n")
# 模拟其他耗时操作
threading.currentThread().sleep(0.1)
# 创建并启动多个线程
threads = []
for i in range(5):
thread = threading.Thread(target=write_to_file, args=(i,))
thread.start()
threads.append(thread)
# 等待所有线程完成
for thread in threads:
thread.join()
# 关闭文件
file.close()
```
在上述代码中,我们创建了一个共享的文件对象 `file` 和一个文件锁 `file_lock` 来确保多个线程写入文件时的互斥访问。每个线程都会调用 `write_to_file` 函数来写入数据到文件中。在写入过程中,线程会获取文件锁,以保证每次只有一个线程在写入操作。在示例中,我们创建了 5 个线程并启动它们,最后等待所有线程完成后关闭文件。
请注意,在多线程写入同一个文件时需要注意并发写入可能导致的数据竞争和错误。因此,在实际应用中,你可能需要更复杂的同步机制来确保线程安全的文件写入操作。
阅读全文
相关推荐
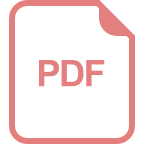
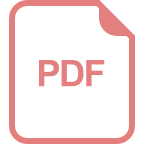















