用c语言,加密规律是:针对小写和大写字母,用原来的字母后面第4个字母代替原来的字母,同时令字符 W , X , Y , Z 分别使用 D , C , B , A 代替(小写字母的替换规则相同)
时间: 2024-12-22 15:28:37 浏览: 18
在C语言中,你可以实现这个简单的凯撒密码(Caesar Cipher)变种算法,通常用于教学目的。这种加密方法通过将每个字符向前移动固定位数来进行加密。下面是基本步骤:
1. 定义两个数组,`lower_shift` 和 `upper_shift`,分别存储小写字母和大写字母的加密映射。对于大写字母Z和小写字母z,我们需要特殊处理。
```c
char lower_shift[] = "efghijklmnopqrstuvwxyzabcd";
char upper_shift[] = "uvwxyz ABCDEFGHIJKLMNOPQRSTUVWXYZ";
```
2. 编写加密函数,接受一个字符作为输入,检查其大小写并应用相应的加密规则。
```c
char encrypt(char c) {
if (islower(c)) { // 小写字母
return lower_shift[c - 'a' + 4];
} else if (isupper(c)) { // 大写字母
return upper_shift[c - 'A' + 4];
} else if (c == 'W') { // 特殊处理W -> D
return 'D';
} else if (c == 'X') { // 特殊处理X -> C
return 'C';
} else if (c == 'Y' || c == 'Z') { // 特殊处理YZ -> BA
return (c == 'Y' ? 'B' : 'A');
}
// 如果不是字母,保持原样
return c;
}
```
3. 对给定的字符串应用此加密函数,遍历每个字符。
```c
void caesar_encrypt(const char* plaintext, char* ciphertext, int shift) {
for (int i = 0; plaintext[i] != '\0'; ++i) {
ciphertext[i] = encrypt(plaintext[i]);
}
}
```
你可以创建一个完整的程序,接收用户输入的明文,然后生成密文。例如:
```c
int main() {
char plaintext[100], ciphertext[100];
printf("Enter the plaintext: ");
fgets(plaintext, sizeof(plaintext), stdin);
plaintext[strlen(plaintext) - 1] = '\0'; // 去除换行符
caesar_encrypt(plaintext, ciphertext, 4); // 使用第4个字母替换
printf("Encrypted message: %s\n", ciphertext);
return 0;
}
```
阅读全文
相关推荐
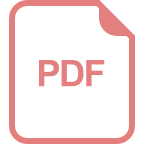
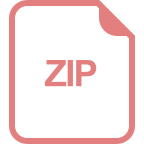
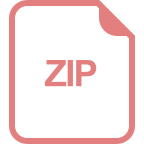















