Python性能提升策略:字典与列表结合使用时的性能考量
发布时间: 2024-09-11 23:33:37 阅读量: 158 订阅数: 37 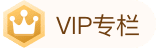
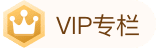
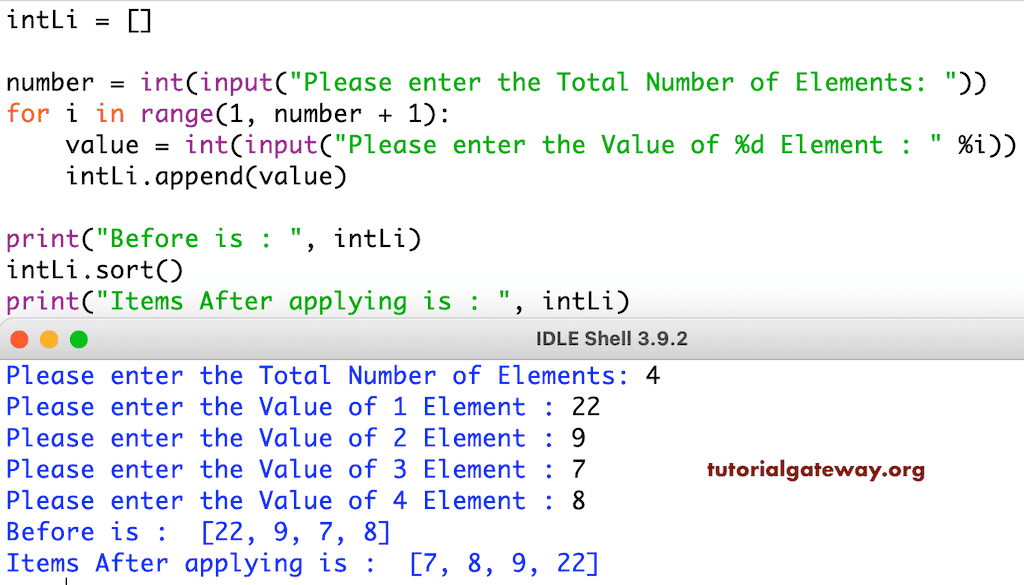
# 1. Python字典与列表基础
## 1.1 列表的基本概念和操作
列表(List)是Python中的一种基本数据类型,它是一个有序的、可变的、且可以包含不同类型元素的集合。创建列表可以使用方括号`[]`,或者用`list()`函数将其他类型的数据转换为列表。
```python
# 创建列表
fruits = ['apple', 'banana', 'cherry']
# 获取列表元素
print(fruits[0]) # 输出: apple
# 列表切片
print(fruits[1:3]) # 输出: ['banana', 'cherry']
```
列表的基本操作包括添加、删除、修改和索引查找等。
## 1.2 字典的基本概念和操作
字典(Dictionary)在Python中也是一种有序且可变的类型,它是一个无序的键值对集合。字典用大括号`{}`包围,键和值通过冒号`:`分隔。
```python
# 创建字典
person = {'name': 'John', 'age': 30}
# 访问字典值
print(person['name']) # 输出: John
# 添加或修改字典中的键值对
person['city'] = 'New York'
```
字典的操作包括键值对的添加、删除、更新和值的检索。
## 1.3 列表与字典的区别与应用场景
列表和字典在Python中都扮演着至关重要的角色,但它们在应用场景上有所不同。列表更适用于存储有序且索引明确的同类型数据集合。而字典则适用于需要通过键快速查找值的场景,尤其是当数据项为不同数据类型时。
选择使用列表还是字典,需要根据具体的应用需求来决定。例如,处理学生分数列表时,可以使用列表,但如果需要根据学生ID来快速检索分数,则使用字典会更加高效。
# 2. 字典与列表的性能特性
## 2.1 字典与列表操作的复杂度分析
### 2.1.1 常见操作的时间复杂度
在Python中,字典和列表是最常用的两种数据结构,它们的操作复杂度对于程序的性能有着直接的影响。以下是一些常见操作的时间复杂度:
- **列表**:
- **append**: O(1) - 最常使用的操作,用于在列表末尾添加一个元素。
- **pop**: O(1) - 在列表末尾移除一个元素。
- **insert**: O(n) - 在列表中任意位置插入一个元素。
- **remove**: O(n) - 移除列表中第一个匹配的元素。
- **index**: O(n) - 查找一个元素在列表中的位置。
- **字典**:
- **get**: O(1) - 快速获取键对应的值。
- **setdefault**: O(1) - 如果键不存在于字典中,则设置一个默认值。
- **popitem**: O(1) - 随机移除并返回字典中的一个键值对。
- **keys/values/items**: O(n) - 返回字典键、值或键值对的视图对象,n为字典中元素的个数。
- **update**: O(n) - 更新字典,通过另一个字典或键值对序列。
值得注意的是,Python的字典在3.6版本之后是有序的,但这并不影响get, setdefault等操作的O(1)复杂度。
### 2.1.2 不同操作下的性能对比
为了更直观地理解字典和列表在不同操作下的性能差异,我们可以通过一些简单的基准测试来对比。例如,我们可以比较在大数据集上添加、查找和删除操作的效率。
下面是一个基准测试的代码示例:
```python
import time
import random
# 初始化一个较大的列表和字典
big_list = list(range(100000))
big_dict = {str(k): k for k in big_list}
def test_list_append性能():
start_time = time.time()
for i in range(10000):
big_list.append(i)
return time.time() - start_time
def test_dict_set性能():
start_time = time.time()
for i in range(10000):
big_dict[str(i)] = i
return time.time() - start_time
print("List Append Performance: ", test_list_append性能())
print("Dict Set Performance: ", test_dict_set性能())
```
在上述代码中,我们创建了一个包含10万个元素的列表和字典,并分别测试了对它们进行1万次append和set操作的性能。根据结果,我们可以判断哪种操作在实际应用中更加高效。
## 2.2 字典与列表的内存消耗比较
### 2.2.1 内存分配机制
Python中的字典和列表有不同的内存分配机制。列表是一种动态数组,而字典在Python 3.6之前的实现是基于开放寻址法的散列表,从Python 3.7开始,字典保持了键值对的插入顺序。
- **列表**:列表的内存分配取决于列表的容量大小,列表容量会随着元素的增加而动态调整。当列表扩展时,可能会导致大量的内存重新分配,这是一个O(n)的操作,因为需要移动所有元素。
- **字典**:字典在创建时分配一个初始大小,随着元素的增加,字典也需要调整大小。字典的调整通常是将内部哈希表的大小加倍,复制原有元素到新的内存位置。尽管如此,单个元素的增删操作通常仍保持O(1)的时间复杂度。
### 2.2.2 大数据量下的内存表现
在处理大数据量时,内存的使用成为一个重要的性能考量点。字典和列表在存储大数据时的表现如下:
- **列表**:由于列表是连续存储的,因此在大数据量时,列表的内存使用会显著增加,尤其是当列表大小接近内存页大小时,可能会导致不连续的内存分配,从而增加内存使用。
- **字典**:字典的内存使用通常会大于等量数据的列表,这是因为字典在内部存储了额外的信息,例如哈希值和指向值的指针。然而,由于字典的存储更加分散,并且支持哈希冲突处理,因此在某些情况下,字典对于快速访问和修改可能是更高效的选择。
下面是一个内存使用比较的示例代码,用于展示列表和字典在存储相同数量元素时的内存差异:
```python
import sys
def memory_usage():
n = 100000
a_list = list(range(n))
a_dict = {str(i): i for i in range(n)}
print("List Memory Usage: ", sys.getsizeof(a_list), "bytes")
print("Dict Memory Usage: ", sys.getsizeof(a_dict), "bytes")
memory_usage()
```
通过此代码我们可以获取到在Python 3环境下,存储同样数量数据时列表和字典的内存使用情况。这能帮助我们更直观地比较在不同场景下的内存使用差异。
[继续第三章:性能优化实战技巧](#)
# 3. 性能优化实战技巧
在数据密集型的编程任务中,性能优化可以显著提升程序的运行效率和资源利用。在本章节,我们将从实战角度出发,介绍在不同场景下如何选择合适的数据结构,以及如何通过高效组合字典与列表来达到优化目的。同时,我们也将探讨一些常见的性能优化策略,并分析其在实际编程中的应用。
## 3.1 数据结构选择的依据
选择合适的数据结构是程序性能优化的第一步。不同的数据结构有着各自的特点和优势,因此在不同的使用场景下,应当仔细考量以做出最优选择。
### 3.1.1 不同场景下的数据结构选择
在需要快速访问元素时,字典结构通常比列表更为高效。字典通过键值对存储数据,利用哈希表实现平均O(1)时间复杂度的快速查找。而列表则适用于元素顺序访问和存储一系列有序数据。
**使用场景示例:**
- **字典:**当需
0
0
相关推荐
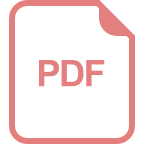
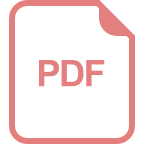
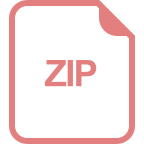
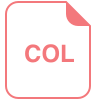
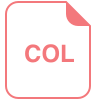
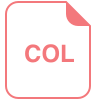
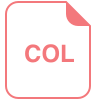
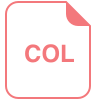