OpenCV图像处理速成指南:从小白到高手
发布时间: 2024-08-14 02:12:32 阅读量: 5 订阅数: 13 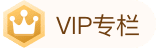
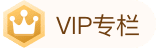

# 1. OpenCV图像处理简介
OpenCV(Open Source Computer Vision Library)是一个开源的计算机视觉库,它提供了广泛的图像处理和计算机视觉算法。它被广泛用于各种应用中,包括图像处理、视频分析、机器学习和增强现实。
OpenCV图像处理涉及使用计算机算法来处理和分析图像数据。它可以用于各种任务,例如图像增强、图像分割、目标检测和图像生成。通过使用OpenCV,开发者可以轻松地构建强大的图像处理应用程序,而无需从头开始开发复杂的算法。
OpenCV图像处理的优势包括:
- **开源和免费:**OpenCV是一个开源库,可以免费使用和修改。
- **广泛的算法:**OpenCV提供了广泛的图像处理和计算机视觉算法,可以满足各种需求。
- **易于使用:**OpenCV提供了一个直观的API,使开发者可以轻松地使用其算法。
- **跨平台支持:**OpenCV支持多种平台,包括Windows、Linux和MacOS。
# 2. 图像处理基础理论
### 2.1 图像数据结构和表示
#### 2.1.1 像素、通道和图像尺寸
图像由像素组成,每个像素代表图像中一个点的颜色信息。像素通常由三个通道表示:红色(R)、绿色(G)和蓝色(B)。这三个通道的值范围通常为 0 到 255,其中 0 表示黑色,255 表示白色。
图像尺寸由宽度和高度表示,单位为像素。例如,一张 640x480 的图像表示其宽度为 640 像素,高度为 480 像素。
#### 2.1.2 图像类型和文件格式
图像类型根据其通道数和像素深度进行分类。最常见的图像类型包括:
* **灰度图像:**单通道图像,像素值表示亮度。
* **RGB 图像:**三通道图像,像素值分别表示红色、绿色和蓝色。
* **RGBA 图像:**四通道图像,RGB 通道外还有一个 Alpha 通道,表示透明度。
图像文件格式用于存储和传输图像数据。常见的图像文件格式包括:
* **JPEG:**有损压缩格式,适用于自然图像。
* **PNG:**无损压缩格式,适用于带有透明度的图像。
* **TIFF:**未压缩格式,适用于高精度图像。
### 2.2 图像处理基本操作
#### 2.2.1 图像读取、显示和保存
在 OpenCV 中,可以使用以下函数读取图像:
```python
import cv2
# 读取图像
image = cv2.imread("image.jpg")
# 显示图像
cv2.imshow("Image", image)
cv2.waitKey(0)
# 保存图像
cv2.imwrite("new_image.jpg", image)
```
#### 2.2.2 图像几何变换
图像几何变换用于调整图像的形状和位置。常见的几何变换包括:
* **缩放:**调整图像的大小。
* **平移:**移动图像。
* **旋转:**旋转图像。
```python
# 缩放图像
scaled_image = cv2.resize(image, (new_width, new_height))
# 平移图像
translated_image = cv2.warpAffine(image, cv2.getTranslationMatrix2d((dx, dy)), (image.shape[1], image.shape[0]))
# 旋转图像
rotated_image = cv2.warpAffine(image, cv2.getRotationMatrix2d((cx, cy), angle, scale), (image.shape[1], image.shape[0]))
```
#### 2.2.3 图像颜色空间转换
图像颜色空间转换用于将图像从一种颜色空间转换为另一种颜色空间。常见的颜色空间转换包括:
* **RGB 到灰度:**将 RGB 图像转换为灰度图像。
* **RGB 到 HSV:**将 RGB 图像转换为 HSV(色相、饱和度、亮度)颜色空间。
* **HSV 到 RGB:**将 HSV 图像转换为 RGB 图像。
```python
# RGB 到灰度
gray_image = cv2.cvtColor(image, cv2.COLOR_RGB2GRAY)
# RGB 到 HSV
hsv_image = cv2.cvtColor(image, cv2.COLOR_RGB2HSV)
# HSV 到 RGB
rgb_image = cv2.cvtColor(hsv_image, cv2.COLOR_HSV2RGB)
```
# 3.1 图像增强
图像增强是图像处理中一项重要的技术,其目的是改善图像的视觉效果,使其更适合于特定任务或应用。图像增强技术有很多种,每种技术都有其独特的优点和缺点。
#### 3.1.1 直方图均衡化
直方图均衡化是一种图像增强技术,其目的是调整图像的直方图,使其更加均匀。直方图是图像中像素值分布的统计表示。均匀的直方图表示图像中所有像素值都均匀分布,而倾斜的直方图表示图像中某些像素值比其他像素值更常见。
直方图均衡化通过将图像中每个像素值映射到新的像素值来工作,使得新的像素值分布更加均匀。这可以改善图像的对比度和亮度,使其更易于查看和分析。
**代码块:**
```python
import cv2
import numpy as np
# 读取图像
image = cv2.imread('image.jpg')
# 转换图像为灰度图像
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# 计算图像的直方图
hist = cv2.calcHist([gray], [0], None, [256], [0, 256])
# 归一化直方图
hist = hist.ravel() / hist.max()
# 创建累积直方图
cdf = hist.cumsum()
# 创建查找表
lookup = np.interp(gray.ravel(), np.arange(256), cdf)
# 应用查找表
equ = lookup.reshape(gray.shape)
# 显示均衡化后的图像
cv2.imshow('Histogram Equalization', equ)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
**逻辑分析:**
* `cv2.calcHist()` 函数计算图像的直方图。
* `hist.ravel()` 将直方图展平为一维数组。
* `hist.max()` 返回直方图中的最大值。
* `hist.cumsum()` 计算直方图的累积和。
* `np.interp()` 函数使用线性插值将灰度值映射到新的像素值。
* `lookup.reshape(gray.shape)` 将查找表重新整形为图像的形状。
* `cv2.imshow()` 函数显示均衡化后的图像。
#### 3.1.2 伽马校正
伽马校正是一种图像增强技术,其目的是调整图像的亮度和对比度。伽马校正通过将图像中每个像素值乘以一个常数来工作,称为伽马值。伽马值大于 1 会使图像变亮,而伽马值小于 1 会使图像变暗。
**代码块:**
```python
import cv2
import numpy as np
# 读取图像
image = cv2.imread('image.jpg')
# 转换图像为灰度图像
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# 应用伽马校正
gamma = 1.5
gamma_corrected = np.power(gray / 255.0, gamma) * 255.0
# 显示伽马校正后的图像
cv2.imshow('Gamma Correction', gamma_corrected)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
**逻辑分析:**
* `cv2.cvtColor()` 函数将图像转换为灰度图像。
* `np.power()` 函数将图像中的每个像素值乘以伽马值。
* `cv2.imshow()` 函数显示伽马校正后的图像。
#### 3.1.3 锐化和模糊
锐化和模糊是图像增强中常用的两种技术,用于增强图像的细节或平滑图像。
* **锐化**通过增强图像中边缘的对比度来工作。这可以使图像中的细节更加明显。
* **模糊**通过平滑图像中像素值之间的过渡来工作。这可以减少图像中的噪声和伪影。
**代码块:**
```python
import cv2
# 读取图像
image = cv2.imread('image.jpg')
# 转换图像为灰度图像
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# 锐化图像
sharpened = cv2.filter2D(gray, -1, np.array([[0, -1, 0], [-1, 5, -1], [0, -1, 0]]))
# 模糊图像
blurred = cv2.GaussianBlur(gray, (5, 5), 0)
# 显示锐化和模糊后的图像
cv2.imshow('Sharpened Image', sharpened)
cv2.imshow('Blurred Image', blurred)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
**逻辑分析:**
* `cv2.filter2D()` 函数使用卷积核对图像进行锐化。
* `cv2.GaussianBlur()` 函数使用高斯核对图像进行模糊。
* `cv2.imshow()` 函数显示锐化和模糊后的图像。
# 4. 图像处理进阶应用
### 4.1 目标检测和识别
**4.1.1 目标检测算法**
目标检测是计算机视觉中一项基本任务,其目的是在图像或视频中找到并定位感兴趣的对象。常见的目标检测算法包括:
- **滑动窗口方法:**使用预定义的窗口在图像中滑动,并使用分类器对窗口中的内容进行分类。
- **区域提议网络 (RPN):**生成一组候选区域,然后使用分类器对这些区域进行分类和回归。
- **单次镜头检测 (SSD):**使用卷积神经网络 (CNN) 同时执行特征提取和目标检测。
- **You Only Look Once (YOLO):**使用单次 CNN 预测图像中的所有目标及其边界框。
**代码块:**
```python
import cv2
# 使用 SSD 模型进行目标检测
model = cv2.dnn.readNetFromCaffe("deploy.prototxt.txt", "mobilenet_iter_73000.caffemodel")
# 加载图像
image = cv2.imread("image.jpg")
# 预处理图像
blob = cv2.dnn.blobFromImage(image, 0.007843, (300, 300), 127.5)
# 设置输入
model.setInput(blob)
# 执行前向传递
detections = model.forward()
# 解析检测结果
for i in np.arange(0, detections.shape[2]):
confidence = detections[0, 0, i, 2]
if confidence > 0.2:
x1, y1, x2, y2 = (detections[0, 0, i, 3:7] * np.array([image.shape[1], image.shape[0], image.shape[1], image.shape[0]])).astype(int)
cv2.rectangle(image, (x1, y1), (x2, y2), (0, 255, 0), 2)
```
**逻辑分析:**
- `readNetFromCaffe()` 函数从 Caffe 模型文件中加载预训练的 SSD 模型。
- `blobFromImage()` 函数将图像转换为深度学习模型所需的输入格式。
- `setInput()` 函数将预处理的图像设置为模型的输入。
- `forward()` 函数执行前向传递,生成目标检测结果。
- 遍历检测结果并过滤掉置信度低于阈值的检测结果。
- 使用检测结果在图像上绘制边界框。
**4.1.2 特征提取和匹配**
特征提取是识别图像中感兴趣对象的关键步骤。常见的特征提取方法包括:
- **直方图定向梯度 (HOG):**计算图像梯度的方向和大小,并将其组织成直方图。
- **局部二值模式 (LBP):**比较像素及其周围像素的强度,生成二进制模式。
- **尺度不变特征变换 (SIFT):**检测图像中的关键点并提取其周围区域的特征描述符。
- **加速稳健特征 (SURF):**类似于 SIFT,但计算速度更快。
**代码块:**
```python
import cv2
# 使用 SIFT 特征提取器
sift = cv2.xfeatures2d.SIFT_create()
# 加载图像
image1 = cv2.imread("image1.jpg")
image2 = cv2.imread("image2.jpg")
# 检测关键点和描述符
keypoints1, descriptors1 = sift.detectAndCompute(image1, None)
keypoints2, descriptors2 = sift.detectAndCompute(image2, None)
# 匹配关键点
bf = cv2.BFMatcher()
matches = bf.knnMatch(descriptors1, descriptors2, k=2)
# 过滤匹配结果
good_matches = []
for m, n in matches:
if m.distance < 0.75 * n.distance:
good_matches.append(m)
# 绘制匹配结果
result = cv2.drawMatchesKnn(image1, keypoints1, image2, keypoints2, good_matches, None, flags=cv2.DrawMatchesFlags_NOT_DRAW_SINGLE_POINTS)
```
**逻辑分析:**
- `detectAndCompute()` 函数检测关键点并提取其描述符。
- `knnMatch()` 函数使用暴力匹配器匹配两个图像中的描述符。
- 过滤匹配结果,仅保留距离比值小于阈值的匹配。
- `drawMatchesKnn()` 函数将匹配结果可视化为图像。
**4.1.3 目标识别和分类**
目标识别和分类是将检测到的目标分类为特定类别的过程。常见的目标识别和分类算法包括:
- **支持向量机 (SVM):**使用超平面将数据点分类到不同的类别。
- **决策树:**使用一系列规则将数据点分配到不同的类别。
- **神经网络:**使用多层感知器或卷积神经网络 (CNN) 对数据点进行分类。
**代码块:**
```python
import cv2
# 使用 SVM 分类器
svm = cv2.ml.SVM_create()
# 加载训练数据
train_data = np.load("train_data.npy")
train_labels = np.load("train_labels.npy")
# 训练分类器
svm.train(train_data, cv2.ml.ROW_SAMPLE, train_labels)
# 加载测试图像
test_image = cv2.imread("test_image.jpg")
# 提取特征
features = ...
# 预测类别
prediction = svm.predict(features)
```
**逻辑分析:**
- `SVM_create()` 函数创建 SVM 分类器。
- 加载训练数据和标签。
- `train()` 函数使用训练数据训练分类器。
- 提取测试图像的特征。
- `predict()` 函数使用训练好的分类器预测测试图像的类别。
# 5. OpenCV图像处理实战项目
### 5.1 人脸检测与识别系统
#### 5.1.1 人脸检测算法
人脸检测是识别图像中人脸位置的过程。OpenCV提供了多种人脸检测算法,包括:
- **Haar级联分类器:**一种基于Haar特征的机器学习算法,速度快,但精度较低。
- **LBP级联分类器:**一种基于局部二值模式的机器学习算法,精度更高,但速度较慢。
- **深度学习算法:**如YOLO、SSD等,精度最高,但计算量也最大。
#### 5.1.2 人脸识别算法
人脸识别是识别图像中特定人脸的过程。OpenCV提供了多种人脸识别算法,包括:
- **特征点匹配:**通过提取人脸特征点并进行匹配来识别。
- **局部二值模式直方图(LBP-Hist):**通过计算人脸LBP直方图并进行匹配来识别。
- **深度学习算法:**如FaceNet、ArcFace等,精度最高,但计算量也最大。
#### 5.1.3 系统实现和应用
人脸检测与识别系统可以实现多种应用,如:
- **安全和监控:**人脸识别用于身份验证、人员追踪和犯罪调查。
- **社交媒体:**人脸检测用于自动标记照片和视频中的人脸。
- **医疗保健:**人脸识别用于患者识别和疾病诊断。
```python
import cv2
# 加载人脸检测模型
face_cascade = cv2.CascadeClassifier('haarcascade_frontalface_default.xml')
# 加载人脸识别模型
face_recognizer = cv2.face.LBPHFaceRecognizer_create()
face_recognizer.read('trained_faces.yml')
# 打开摄像头
cap = cv2.VideoCapture(0)
while True:
# 读取帧
ret, frame = cap.read()
# 转换帧为灰度图
gray = cv2.cvtColor(frame, cv2.COLOR_BGR2GRAY)
# 人脸检测
faces = face_cascade.detectMultiScale(gray, 1.1, 5)
# 遍历人脸
for (x, y, w, h) in faces:
# 人脸识别
id, confidence = face_recognizer.predict(gray[y:y+h, x:x+w])
# 绘制人脸框和识别结果
cv2.rectangle(frame, (x, y), (x+w, y+h), (0, 255, 0), 2)
cv2.putText(frame, str(id), (x, y-10), cv2.FONT_HERSHEY_SIMPLEX, 0.9, (0, 255, 0), 2)
# 显示帧
cv2.imshow('frame', frame)
# 按'q'退出
if cv2.waitKey(1) & 0xFF == ord('q'):
break
# 释放摄像头
cap.release()
# 销毁窗口
cv2.destroyAllWindows()
```
0
0
相关推荐
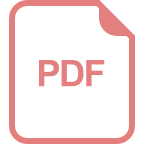
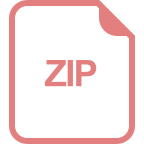
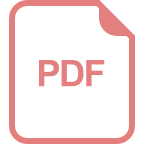





