使用Spring Security加强Web应用的安全性
发布时间: 2024-01-26 09:01:45 阅读量: 49 订阅数: 31 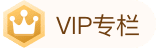
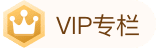
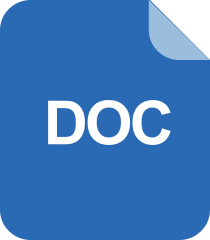
使用 Spring Security 保护 Web 应用的安全

# 1. Spring Security简介
## 1.1 Spring Security的概念和作用
Spring Security是一个功能强大且高度可定制的身份验证和访问控制框架。它为Java应用程序提供了全面的安全性解决方案,可以轻松集成到Spring框架中。
## 1.2 Spring Security在Web应用中的应用场景
Spring Security可用于保护Web应用的各个方面,包括用户身份验证、授权、防止攻击、会话管理等。它能够确保应用程序的安全性,并提供灵活的配置选项,以满足不同的安全需求。
## 1.3 Spring Security的核心功能和特点
Spring Security的核心功能包括认证(Authentication)和授权(Authorization),它提供了丰富的API和插件,以支持各种认证方式(如基本认证、表单登录、LDAP认证等)和授权策略(如角色控制、资源控制等)。同时,它还具有高度的可扩展性和定制性,能够满足复杂安全需求的实现。
# 2. Spring Security的基本配置
在本章中,我们将介绍如何在Spring项目中集成Spring Security,并进行基本的配置,实现认证和授权的功能。
#### 2.1 在Spring项目中集成Spring Security
首先,我们需要在项目的依赖中添加Spring Security的相关库。在Maven项目中,可以在`pom.xml`文件中添加以下依赖:
```xml
<dependencies>
<!-- Spring Security -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
</dependencies>
```
在Gradle项目中,可以在`build.gradle`文件中添加以下依赖:
```groovy
dependencies {
// Spring Security
implementation 'org.springframework.boot:spring-boot-starter-security'
}
```
完成依赖的添加后,我们可以通过在项目中创建一个配置类来进行Spring Security的基本配置。新建一个类并添加`@EnableWebSecurity`注解:
```java
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
// 配置认证和授权规则
http.authorizeRequests()
.antMatchers("/admin/**").hasRole("ADMIN")
.antMatchers("/user/**").hasAnyRole("ADMIN", "USER")
.anyRequest().authenticated()
.and()
.formLogin().permitAll()
.and()
.logout().permitAll();
}
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
// 配置用户存储源和认证流程
auth.inMemoryAuthentication()
.withUser("admin").password("{noop}admin123").roles("ADMIN")
.and()
.withUser("user").password("{noop}user123").roles("USER");
}
}
```
在上述代码中,我们通过重写`configure(HttpSecurity http)`方法来配置认证和授权规则,通过`configure(AuthenticationManagerBuilder auth)`方法来配置用户存储源和认证流程。这里使用了内存中的用户存储源,并添加了两个用户。
#### 2.2 配置Spring Security的基本认证和授权规则
在上一节的代码中,我们配置了Spring Security的认证和授权规则。以下是一些常见的配置示例:
- `.antMatchers("/admin/**").hasRole("ADMIN")`:要求访问"/admin/**"路径的用户需要拥有"ADMIN"角色。
- `.antMatchers("/user/**").hasAnyRole("ADMIN", "USER")`:要求访问"/user/**"路径的用户需要拥有"ADMIN"或"USER"角色。
- `.anyRequest().authenticated()`:要求所有请求都必须经过认证才能访问。
- `.formLogin().permitAll()`:允许使用表单登录进行认证。
- `.logout().permitAll()`:允许注销登录。
#### 2.3 使用Spring Security提供的默认登录页面和认证逻辑
通过配置`.formLogin().permitAll()`,Spring Security会自动提供一个简单的登录页面和认证逻辑。在登录页面中输入正确的用户名和密码,即可进行登录。
需要注意的是,在上述的配置类中,我们使用了明文密码,这是为了演示简单起见。在实际项目中,我们应该使用加密的密码进行存储和认证。
以上就是Spring Security的基本配置。在下一章中,我们将介绍如何自定义认证和授权规则。
# 3. 自定义认证和授权规则
在实际项目中,我们往往需要根据业务需求对认证和授权规则进行定制化。Spring Security提供了丰富的扩展机制,可以让我们轻松地自定义认证和授权规则。
### 3.1 使用自定义的用户存储源
#### 代码场景:
首先,我们需要创建一个自定义的用户存储源(UserDetailsService),用于获取用户信息和权限。假设我们的项目中使用了数据库进行用户信息的存储,我们可以参考以下代码示例:
```java
@Component
public class CustomUserDetailsService implements UserDetailsService {
@Autowired
private UserRepository userRepository;
@Override
public UserDetails loadUserByUsername(String username) throws UsernameNotFoundException {
User user = userRepository.findByUsername(username);
if (user == null) {
throw new UsernameNotFoundException("User not found");
}
return new CustomUserDetails(user);
}
}
```
上述代码中,我们通过自定义的`UserDetailsService`接口实现类`CustomUserDetailsService`,从数据库中根据用户名查找用户信息,并将其封装为`CustomUserDetails`对象。
#### 代码解释:
- `UserRepository`:自定义的用户数据访问类,用于与数据库交互。
- `loadUserByUsername`方法:根据用户名查询数据库中的用户信息,并将其封装为Spring Security所需的`UserDetails`接口的实现类`CustomUserDetails`对象。
### 3.2 自定义认证流程和逻辑
#### 代码场景:
接下来,我们将自定义认证流程和逻辑,假设我们的认证逻辑需要额外校验用户的某些字段,请参考以下示例代码:
```java
@Component
public class CustomAuthenticationProvider implements AuthenticationProvider {
@Autowired
private CustomUserDetailsService userDetailsService;
@Override
public Authentication authenticate(Authentication authentication) throws AuthenticationException {
String username = authentication.getName();
String password = authentication.getCredentials().toString();
UserDetails userDetails = userDetailsService.loadUserByUsername(username);
// 自定义校验逻辑
if (!customCheck(username, password)) {
throw new BadCredentialsException("Invalid username or password");
}
return new UsernamePasswordAuthenticationToken(userDetails, password, userDetails.getAuthorities());
}
@Override
public boolean supports(Class<?> authentication) {
return authentication.equals(UsernamePasswordAuthenticationToken.class);
}
private boolean customCheck(String username, String password) {
// 自定义校验逻辑,例如校验用户字段等
return true;
}
}
```
上述代码中,我们通过自定义的`AuthenticationProvider`接口实现类`CustomAuthenticationProvider`,可以在认证流程中加入我们自己的逻辑,比如校验用户其他字段等。
#### 代码解释:
- `CustomUserDetailsService`:自定义的用户存储源,同样需要在认证流程中调用该服务获取用户信息。
- `authenticate`方法:重写认证逻辑,同时调用并扩展了`CustomUserDetailsService`的`loadUserByUsername`方法,和自定义的校验逻辑`customCheck`方法。
### 3.3 自定义授权规则和权限管理
#### 代码场景:
在Spring Security中,我们可以通过自定义AccessDecisionManager和AccessDecisionVoter来实现对权限的控制。以下是一个示例代码:
```java
@Component
public class CustomDecisionManager implements AccessDecisionManager {
@Override
public void decide(Authentication authentication, Object object, Collection<ConfigAttribute> attributes)
throws AccessDeniedException, InsufficientAuthenticationException {
// 自定义授权规则的实现逻辑,例如根据用户角色判断是否具有权限
// 可以使用ConfigAttribute中的角色信息和authentication中的用户信息进行判断
// 如果没有权限,可以抛出AccessDeniedException异常
}
@Override
public boolean supports(ConfigAttribute attribute) {
// 在这里可以指定支持的ConfigAttribute类型
return true;
}
@Override
public boolean supports(Class<?> clazz) {
// 在这里可以指定支持的Class类型
return true;
}
}
```
上述代码中,我们通过自定义的`AccessDecisionManager`接口实现类`CustomDecisionManager`,可以根据具体需求编写我们自己的授权规则和权限管理逻辑。
#### 代码解释:
- `decide`方法:自定义授权规则的实现逻辑,例如根据用户角色判断是否具有权限。
- `supports`方法:指定支持的ConfigAttribute类型和Class类型。
通过自定义认证和授权规则,我们可以根据实际项目需求对Spring Security进行个性化定制,以满足项目的安全性要求。
注:以上代码只是为了演示自定义认证和授权规则的基本思路和流程,实际项目中应根据具体业务逻辑进行进一步开发和扩展。
到目前为止,我们已经了解了Spring Security中自定义认证和授权规则的方法,接下来,我们将介绍如何使用Spring Security加强Web应用的安全性。
# 4. 使用Spring Security加强Web应用的安全性
在本章中,我们将探讨如何使用Spring Security来加强Web应用的安全性。我们将介绍一些常见的Web攻击方式,并使用Spring Security来防止它们。我们还将学习如何保护敏感资源和API,并了解如何管理会话和登录状态。
### 4.1 防止常见的Web攻击
在Web开发中,安全是一个至关重要的方面。为了保护我们的应用和用户数据,我们需要防止一些常见的Web攻击,例如跨站请求伪造(CSRF)和跨站脚本攻击(XSS)。
#### 4.1.1 跨站请求伪造(CSRF)防护
跨站请求伪造(CSRF)是一种攻击方式,攻击者通过在受害者浏览器中伪造请求,以受害者身份进行恶意操作。为了防止CSRF攻击,我们可以使用Spring Security提供的CSRF防护功能。
首先,我们需要在我们的Web应用中启用CSRF防护,可以通过在Spring Security配置中添加以下代码来实现:
```java
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.csrf().csrfTokenRepository(CookieCsrfTokenRepository.withHttpOnlyFalse());
}
```
上述配置将启用Spring Security的CSRF防护,并使用Cookie作为CSRF令牌的存储方式。
#### 4.1.2 跨站脚本攻击(XSS)防护
跨站脚本攻击(XSS)是一种攻击方式,攻击者通过在受害者浏览器中注入恶意脚本来执行攻击。为了防止XSS攻击,我们可以使用Spring Security提供的XSS防护功能。
在Spring Security的配置中,我们可以通过添加以下代码来启用XSS防护:
```java
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.headers()
.xssProtection().block(true);
}
```
通过上述配置,Spring Security将在HTTP响应中启用X-XSS-Protection头,以阻止浏览器执行恶意脚本。
### 4.2 使用Spring Security保护敏感资源和API
在Web应用中,可能存在一些敏感的资源和API,例如用户个人信息、支付接口等。为了保护这些敏感资源和API,我们可以使用Spring Security进行访问控制。
我们可以通过以下方式来配置Spring Security的访问控制规则:
```java
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.antMatchers("/admin/**").hasRole("ADMIN")
.antMatchers("/user/**").hasRole("USER")
.anyRequest().authenticated()
.and()
.formLogin()
.loginPage("/login")
.permitAll()
.and()
.logout()
.permitAll();
}
```
上述配置将指定"/admin/**"路径需要具有"ADMIN"角色的用户才能访问,而"/user/**"路径需要具有"USER"角色的用户才能访问。其他路径需要进行身份验证后才能访问。
### 4.3 使用Spring Security管理会话和登录状态
在Web应用中,会话和登录状态的管理非常重要。为了提高安全性,我们可以使用Spring Security来管理会话和登录状态。
首先,我们可以配置Spring Security使用基于Cookie的会话管理:
```java
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.sessionManagement()
.sessionCreationPolicy(SessionCreationPolicy.ALWAYS)
.sessionFixation().none()
.invalidSessionUrl("/login")
.maximumSessions(1)
.expiredUrl("/login");
}
```
上述配置将指定在用户进行身份验证后,Spring Security将始终创建新的会话,禁止使用URL重写防止固定会话攻击,并设置无效会话的重定向URL和最大会话数。
另外,我们还可以通过以下方式来配置Spring Security管理登录状态:
```java
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.rememberMe()
.tokenValiditySeconds(60 * 60 * 24 * 7)
.key("uniqueAndSecretKey")
.rememberMeParameter("rememberMe")
.rememberMeCookieName("rememberMeCookie");
}
```
上述配置将指定记住我功能的有效期为7天,并设置唯一的密钥、参数名和Cookie名。
通过使用Spring Security的会话和登录状态管理功能,我们可以提高应用的安全性和用户体验。
总结:
在本章中,我们学习了如何使用Spring Security来加强Web应用的安全性。我们了解了防止跨站请求伪造(CSRF)和跨站脚本攻击(XSS)的方法,以及如何使用Spring Security保护敏感资源和API。我们还学习了如何使用Spring Security管理会话和登录状态。通过合理配置Spring Security,我们可以提高应用的安全性并保护用户数据的安全。
# 5. 高级安全特性和扩展
### 5.1 使用二次认证提高安全性
二次认证(Two-Factor Authentication,简称2FA)是一种提高账户安全性的方法,它要求用户在登录时除了使用密码外,还需要提供另外一种身份验证方式,例如短信验证、指纹识别、动态口令等。Spring Security提供了对二次认证的支持,可以很方便地集成二次认证功能到应用中。
#### 5.1.1 使用短信验证实现二次认证
```java
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
// 其他配置代码省略
@Override
protected void configure(HttpSecurity http) throws Exception {
http
// 其他配置代码省略
.and()
.apply(smsCodeAuthenticationSecurityConfig);
}
}
```
```java
@Component
public class SmsCodeAuthenticationSecurityConfig extends
SecurityConfigurerAdapter<DefaultSecurityFilterChain, HttpSecurity> {
@Autowired
private SmsCodeAuthenticationProvider smsCodeAuthenticationProvider;
@Override
public void configure(HttpSecurity http) throws Exception {
SmsCodeAuthenticationFilter smsCodeAuthenticationFilter = new SmsCodeAuthenticationFilter();
smsCodeAuthenticationFilter.setAuthenticationManager(http.getSharedObject(AuthenticationManager.class));
smsCodeAuthenticationFilter.setAuthenticationSuccessHandler(new SmsCodeAuthenticationSuccessHandler());
smsCodeAuthenticationFilter.setAuthenticationFailureHandler(new SmsCodeAuthenticationFailureHandler());
SmsCodeAuthenticationProvider smsCodeAuthenticationProvider = new SmsCodeAuthenticationProvider();
smsCodeAuthenticationProvider.setUserDetailsService(userDetailsService);
smsCodeAuthenticationProvider.setPasswordEncoder(passwordEncoder());
http.authenticationProvider(smsCodeAuthenticationProvider)
.addFilterAfter(smsCodeAuthenticationFilter, UsernamePasswordAuthenticationFilter.class);
}
}
```
```java
@Component
public class SmsCodeAuthenticationProvider implements AuthenticationProvider {
@Override
public Authentication authenticate(Authentication authentication) throws AuthenticationException {
SmsCodeAuthenticationToken authenticationToken = (SmsCodeAuthenticationToken) authentication;
// 根据手机号和短信验证码进行认证逻辑
String mobile = (String) authenticationToken.getPrincipal();
String code = (String) authenticationToken.getCredentials();
UserDetails user = userDetailsService.loadUserByMobile(mobile);
if (user == null) {
throw new BadCredentialsException("手机号未注册");
}
if (!smsCodeService.verifyCode(mobile, code)) {
throw new BadCredentialsException("短信验证码错误");
}
SmsCodeAuthenticationToken authenticationResult = new SmsCodeAuthenticationToken(user, user.getAuthorities());
authenticationResult.setDetails(authenticationToken.getDetails());
return authenticationResult;
}
@Override
public boolean supports(Class<?> authentication) {
return SmsCodeAuthenticationToken.class.isAssignableFrom(authentication);
}
}
```
#### 5.1.2 集成动态口令实现二次认证
```java
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
// 其他配置代码省略
@Override
protected void configure(HttpSecurity http) throws Exception {
http
// 其他配置代码省略
.and()
.apply(totpAuthenticationSecurityConfig);
}
}
```
```java
@Component
public class TotpAuthenticationSecurityConfig extends
SecurityConfigurerAdapter<DefaultSecurityFilterChain, HttpSecurity> {
@Override
public void configure(HttpSecurity http) throws Exception {
TotpAuthenticationFilter totpAuthenticationFilter = new TotpAuthenticationFilter();
totpAuthenticationFilter.setAuthenticationManager(http.getSharedObject(AuthenticationManager.class));
totpAuthenticationFilter.setAuthenticationSuccessHandler(new TotpAuthenticationSuccessHandler());
totpAuthenticationFilter.setAuthenticationFailureHandler(new TotpAuthenticationFailureHandler());
TotpAuthenticationProvider totpAuthenticationProvider = new TotpAuthenticationProvider();
totpAuthenticationProvider.setUserDetailsService(userDetailsService);
totpAuthenticationProvider.setPasswordEncoder(passwordEncoder());
http.authenticationProvider(totpAuthenticationProvider)
.addFilterAfter(totpAuthenticationFilter, UsernamePasswordAuthenticationFilter.class);
}
}
```
```java
@Component
public class TotpAuthenticationProvider implements AuthenticationProvider {
@Override
public Authentication authenticate(Authentication authentication) throws AuthenticationException {
TotpAuthenticationToken authenticationToken = (TotpAuthenticationToken) authentication;
// 根据用户名和动态口令进行认证逻辑
String username = (String) authenticationToken.getPrincipal();
String totpCode = (String) authenticationToken.getCredentials();
UserDetails user = userDetailsService.loadUserByUsername(username);
if (user == null) {
throw new BadCredentialsException("用户不存在");
}
VerificationResult result = totpService.verifyCode(user.getTotpSecret(), totpCode);
if (!result.isSuccess()) {
throw new BadCredentialsException("动态口令错误");
}
TotpAuthenticationToken authenticationResult = new TotpAuthenticationToken(user, user.getAuthorities());
authenticationResult.setDetails(authenticationToken.getDetails());
return authenticationResult;
}
@Override
public boolean supports(Class<?> authentication) {
return TotpAuthenticationToken.class.isAssignableFrom(authentication);
}
}
```
### 5.2 集成多因素认证(MFA)
多因素认证(Multi-Factor Authentication,简称MFA)是一种更加安全的身份验证方式,它要求用户在登录时提供多个独立的验证因素,例如密码、指纹、手机动态口令等多种因素的组合。Spring Security的扩展库Spring Security MFA提供了对MFA的支持,可以很方便地集成MFA功能到应用中。
#### 5.2.1 配置Spring Security MFA
首先,在Spring项目中引入spring-security-mfa依赖:
```xml
<dependency>
<groupId>org.springframework.security.extensions</groupId>
<artifactId>spring-security-mfa</artifactId>
<version>1.0.0</version>
</dependency>
```
然后,在Spring Security的配置类中使用`MfaConfigurer`配置MFA:
```java
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
private final MfaConfigurer mfaConfigurer;
public SecurityConfig(MfaConfigurer mfaConfigurer) {
this.mfaConfigurer = mfaConfigurer;
}
@Override
protected void configure(HttpSecurity http) throws Exception {
http
// 其他配置代码省略
.and()
.apply(mfaConfigurer)
.customAuthenticationDetailsSource(mfaAuthenticationDetailsSource);
}
}
```
#### 5.2.2 集成手机动态口令实现MFA
首先,配置MFA的身份验证提供者:
```java
@Component
public class MfaAuthenticationProvider implements AuthenticationProvider {
@Override
public Authentication authenticate(Authentication authentication) throws AuthenticationException {
MfaAuthenticationToken authenticationToken = (MfaAuthenticationToken) authentication;
// 根据用户名和动态口令进行认证逻辑
String username = (String) authenticationToken.getPrincipal();
String mfaCode = (String) authenticationToken.getCredentials();
UserDetails user = userDetailsService.loadUserByUsername(username);
if (user == null) {
throw new BadCredentialsException("用户不存在");
}
VerificationResult result = mfaService.verifyCode(user.getMfaSecret(), mfaCode);
if (!result.isSuccess()) {
throw new BadCredentialsException("动态口令错误");
}
MfaAuthenticationToken authenticationResult = new MfaAuthenticationToken(user, mfaCode, user.getAuthorities());
authenticationResult.setDetails(authenticationToken.getDetails());
return authenticationResult;
}
@Override
public boolean supports(Class<?> authentication) {
return MfaAuthenticationToken.class.isAssignableFrom(authentication);
}
}
```
然后,配置MFA的过滤器:
```java
@Component
public class MfaAuthenticationFilter extends AbstractAuthenticationProcessingFilter {
public MfaAuthenticationFilter() {
super(new AntPathRequestMatcher("/mfa", "POST"));
}
@Override
public Authentication attemptAuthentication(HttpServletRequest request, HttpServletResponse response) throws AuthenticationException, IOException, ServletException {
String username = request.getParameter("username");
String mfaCode = request.getParameter("mfaCode");
MfaAuthenticationToken authenticationToken = new MfaAuthenticationToken(username, mfaCode);
return this.getAuthenticationManager().authenticate(authenticationToken);
}
}
```
接下来,配置MFA的认证流程:
```java
@Configuration
public class MfaAuthenticationFlow extends AbstractMfaAuthenticationFlowConfigurer {
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
auth.authenticationProvider(mfaAuthenticationProvider);
}
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.addFilterAfter(mfaAuthenticationFilter, UsernamePasswordAuthenticationFilter.class)
.authorizeRequests().antMatchers("/mfa").authenticated()
.and()
.mfa().requireMfaAuthenticated().mfaAuthenticationFilterId("mfaAuthenticationFilter");
}
}
```
### 5.3 集成其他安全技术(如OAuth、OpenID Connect等)
除了二次认证和多因素认证,Spring Security还提供了对其他安全协议和标准的支持,例如OAuth、OpenID Connect等。通过集成这些安全技术,我们可以实现更加灵活和安全的身份验证和授权机制。
#### 5.3.1 集成OAuth实现第三方登录
```java
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http
// 其他配置代码省略
.and()
.apply(oauthSecurityConfig);
}
}
```
```java
@Component
public class OAuthSecurityConfig extends SecurityConfigurerAdapter<DefaultSecurityFilterChain, HttpSecurity> {
@Override
public void configure(HttpSecurity http) throws Exception {
http
// 配置OAuth2登录认证流程
.oauth2Login()
.loginPage("/login")
.authorizationEndpoint()
.baseUri("/oauth2/authorize")
.authorizationRequestRepository(cookieAuthorizationRequestRepository())
.and()
.userInfoEndpoint()
.userService(oauth2UserService)
.and()
.successHandler(oauth2AuthenticationSuccessHandler)
.failureHandler(oauth2AuthenticationFailureHandler);
}
}
```
#### 5.3.2 集成OpenID Connect实现单点登录
```java
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http
// 其他配置代码省略
.and()
.apply(openidConnectSecurityConfig);
}
}
```
```java
@Component
public class OpenidConnectSecurityConfig extends SecurityConfigurerAdapter<DefaultSecurityFilterChain, HttpSecurity> {
@Override
public void configure(HttpSecurity http) throws Exception {
http
// 配置OpenID Connect登录认证流程
.openidLogin()
.loginPage("/login")
.loginProcessingUrl("/openid_connect/auth")
.userInfoEndpoint()
.customUserType(OpenidConnectUser.class, "openid_connect")
.and()
.and()
.successHandler(openidConnectAuthenticationSuccessHandler)
.failureHandler(openidConnectAuthenticationFailureHandler);
}
}
```
本章介绍了使用Spring Security实现二次认证、多因素认证以及集成其他安全技术的方法。通过合理配置和使用这些高级安全特性,我们可以提高应用的安全性和用户体验。在实际项目中,根据具体需求选择适合的认证和授权方式,并结合业务场景进行定制化开发,以达到最佳的安全性和用户体验。
*代码示例为Java语言,具体的实现细节和配置方式可能因版本和框架不同而有所差异,可根据具体情况进行调整。*
# 6. Spring Security的最佳实践和未来发展
在本章中,我们将探讨Spring Security在实际项目中的最佳实践以及未来的发展趋势。我们将介绍一些常见的最佳实践,并讨论如何将Spring Security应用于具体的项目和实际场景中。同时,我们还将展望Spring Security未来版本的新功能和计划。
## 6.1 Spring Security的最佳实践和常见问题解决方案
在实际项目中,使用Spring Security时可能会遇到一些常见问题,本节将介绍一些解决这些问题的最佳实践和方法。以下是一些常见问题和解决方案:
### 6.1.1 如何处理用户密码的安全存储
在用户认证过程中,密码的安全存储是非常重要的。我们将介绍如何使用安全的密码存储方案,例如加盐哈希算法和密码加密等。
```java
// 密码加盐和哈希示例代码
PasswordEncoder passwordEncoder = new BCryptPasswordEncoder();
String rawPassword = "password";
String encodedPassword = passwordEncoder.encode(rawPassword);
// 密码验证示例代码
if (passwordEncoder.matches(rawPassword, encodedPassword)) {
// 密码验证成功
} else {
// 密码验证失败
}
```
### 6.1.2 如何处理用户身份验证和授权
在使用Spring Security时,如何正确处理用户身份验证和授权是一个关键问题。我们将介绍如何使用适当的认证和授权策略来确保系统的安全性。
```java
// 配置用户身份认证和授权示例代码
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
auth.inMemoryAuthentication()
.withUser("user").password(passwordEncoder().encode("password")).roles("USER")
.and()
.withUser("admin").password(passwordEncoder().encode("password")).roles("ADMIN");
}
@Override
protected void configure(HttpSecurity http) throws Exception {
http.authorizeRequests()
.antMatchers("/admin/**").hasRole("ADMIN")
.anyRequest().authenticated()
.and()
.formLogin()
.and()
.logout().logoutUrl("/logout").logoutSuccessUrl("/login");
}
```
### 6.1.3 如何使用安全的会话管理
在Web应用中,会话管理是非常重要的。我们将介绍如何使用Spring Security来管理会话,如设置会话超时时间、禁止并发会话等。
```java
// 配置会话管理示例代码
@Override
protected void configure(HttpSecurity http) throws Exception {
http.sessionManagement()
.invalidSessionUrl("/login")
.maximumSessions(1)
.maxSessionsPreventsLogin(true)
.expiredUrl("/login?expired=true");
}
```
## 6.2 Spring Security的发展趋势和未来版本的新功能
Spring Security作为一个开源项目,不断发展着。Spring Security的未来版本将引入一些新功能来进一步提升系统的安全性和易用性。
以下是一些未来版本的新功能和计划:
- 引入更多的认证和授权策略,以满足不同的安全需求。
- 支持新的认证方式,例如基于硬件的认证和生物特征认证等。
- 提供更灵活的会话管理和登录状态管理策略。
- 引入更多的安全性检测工具和报告,帮助开发人员发现和解决安全漏洞。
- 支持更多的Web应用框架和云平台。
## 6.3 怎样将Spring Security应用于具体的项目和实际场景中
最后,我们将讨论如何将Spring Security应用于具体的项目和实际场景中。我们将介绍一些实际案例,并给出一些建议和技巧,帮助读者更好地使用Spring Security。
以下是一些将Spring Security应用于具体项目的建议:
- 根据项目需求选择合适的认证和授权策略,并进行适当的定制和配置。
- 尽可能使用安全的密码存储方案,避免使用明文存储密码。
- 根据实际情况设置合理的会话管理策略,保证会话的安全性和有效性。
- 定期更新Spring Security版本,以获得最新的功能和安全性修复。
- 在开发过程中进行安全性测试,并及时修复发现的安全漏洞。
希望本章内容能够帮助读者更好地理解和应用Spring Security,并在实际项目中提升系统的安全性。
以上就是本章的内容,我们介绍了Spring Security的最佳实践和常见问题解决方案,展望了Spring Security的未来发展趋势和新功能,并给出了将Spring Security应用于具体项目的建议。希望这些内容对读者有所帮助!
0
0
相关推荐
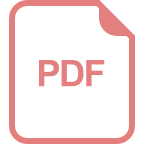
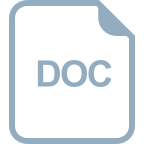
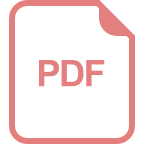
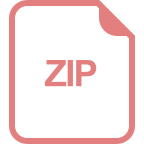
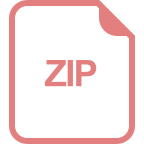
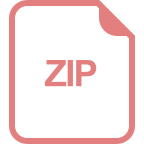
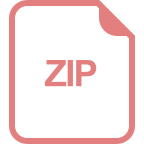
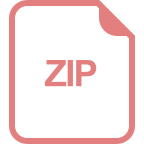