打造无缝动画效果:使用MotionLayout
发布时间: 2023-12-31 08:19:50 阅读量: 11 订阅数: 13 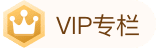
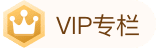
# 章节一:介绍MotionLayout
## 1.1 什么是MotionLayout
MotionLayout是Android平台上一个强大的布局容器,可以帮助开发者轻松实现复杂的动画效果。它基于ConstraintLayout,能够在不编写大量代码的情况下,实现各种复杂的动画和过渡效果。
## 1.2 MotionLayout的优势和作用
MotionLayout的优势主要体现在其便捷性和灵活性。开发者可以通过简单定义布局的起始和结束状态,就能够轻松实现平滑的动画过渡效果,而无需编写大量繁琐的动画代码。
## 1.3 MotionLayout在移动应用开发中的应用场景
MotionLayout广泛应用于移动应用开发中,例如在用户界面过渡、导航栏动画、卡片式布局等方面,可以帮助开发者实现更加流畅、美观的用户体验。
## 章节二:MotionLayout基础知识
MotionLayout的基础知识是理解和使用该库的关键,本章将介绍MotionLayout的工作原理、基本属性和语法,以及在项目中引入MotionLayout的方法。让我们一起来深入了解MotionLayout的基础知识。
## 章节三:创建基本动画效果
### 3.1 使用ConstraintSet定义起始和结束状态
动画效果的实现通常需要定义起始状态和结束状态,而在MotionLayout中,可以使用ConstraintSet来定义这些状态。ConstraintSet是一个用于定义布局约束的类,它包含了所有视图的约束关系。
下面以一个简单的示例来说明如何使用ConstraintSet来创建动画效果。假设我们有一个布局文件,其中包含一个按钮和一个文本视图。我们希望在点击按钮时,文本视图向下移动。
首先,在res目录下创建一个新的XML文件,命名为`motion_scene.xml`,用于定义动画场景:
```xml
<MotionScene xmlns:android="http://schemas.android.com/apk/res-auto">
<ConstraintSet android:id="@+id/start">
<Constraint
android:id="@+id/tv_text"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="16dp"
android:layout_centerHorizontal="true"
android:layout_marginBottom="@id/btn_button"
android:text="Hello MotionLayout" />
<Constraint
android:id="@+id/btn_button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_centerHorizontal="true"
android:layout_marginBottom="16dp"
android:text="Click Me" />
</ConstraintSet>
<ConstraintSet android:id="@+id/end">
<Constraint
android:id="@+id/tv_text"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="100dp"
android:layout_centerHorizontal="true"
android:layout_marginBottom="@id/btn_button"
android:text="Hello MotionLayout" />
<Constraint
android:id="@+id/btn_button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_centerHorizontal="true"
android:layout_marginBottom="100dp"
android:text="Click Me" />
</ConstraintSet>
<Transition
android:constraintSetStart="@id/start"
android:constraintSetEnd="@id/end"
android:duration="500">
<OnClick
android:target="@id/btn_button"
android:onClick="onClick" />
</Transition>
</MotionScene>
```
在上述代码中,我们定义了两个ConstraintSet,分别为起始状态(start)和结束状态(end)。在起始状态中,文本视图和按钮的位置以及其他属性都在`<Constraint>`标签中进行了定义。在结束状态中,我们将文本视图向下移动了100dp。同时,在`<Transition>`标签中指定了从起始状态到结束状态的过渡时间为500毫秒,并且指定了按钮的点击事件为`onClick`方法。
接下来,在对应的Activity或Fragment中,通过如下方式加载动画场景和设置MotionLayout:
```java
MotionLayout motionLayout = findViewById(R.id.motion_layout);
MotionScene motionScene = MotionScene.createFromXml(this, R.xml.motion_scene);
motionLayout.setTransition(motionScene.getTransition(R.id.transition));
```
在上述代码中,我们首先通过`MotionScene.createFromXml()`方法加载动画场景,然后通过`MotionLayout.setTransition()`方法将加载的动画场景应用到MotionLayout上。
最后,在对应的Activity或Fragment中实现按钮的点击事件:
```java
public void onClick(View view) {
motionLayout.transitionToEnd();
}
```
在上述代码中,我们通过调用`motionLayout.transitionToEnd()`方法来触发动画的播放,使文本视图向下移动到结束状态。
运行程序,点击按钮,可以看到文本视图向下移动的动画效果。
### 3.2 MotionLayout中的关键帧动画
除了使用起始状态和结束状态来创建动画效果之外,MotionLayout还支持关键帧动画的创建。关键帧动画是指将动画效果分解为多个关键帧,在每个关键帧上定义视图的属性和位置,从而实现更为自由和精细的动画效果。
下面以一个渐变效果的示例来说明如何在MotionLayout中创建关键帧动画。假设我们有一个布局文件,其中包含一个按钮和一个文本视图。我们希望在点击按钮时,文本视图的背景色从红色渐变到蓝色。
首先,在res目录下创建一个新的XML文件,命名为`motion_scene.xml`,用于定义动画场景:
```xml
<MotionScene xmlns:android="http://schemas.android.com/apk/res-auto">
<ConstraintSet android:id="@+id/start">
<Constraint
android:id="@+id/tv_text"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="16dp"
android:layout_centerHorizontal="true"
android:background="@color/red"
android:text="Hello MotionLayout" />
<Constraint
android:id="@+id/btn_button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_centerHorizontal="true"
android:layout_marginTop="100dp"
android:text="Click Me" />
</ConstraintSet>
<ConstraintSet android:id="@+id/end">
<Constraint
android:id="@+id/tv_text"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="16dp"
android:layout_centerHorizontal="true"
android:background="@color/blue"
android:text="Hello MotionLayout" />
<Constraint
android:id="@+id/btn_button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_centerHorizontal="true"
android:layout_marginTop="100dp"
android:text="Click Me" />
</ConstraintSet>
<ConstraintSet android:id="@+id/frame1">
<Constraint
android:id="@+id/tv_text"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="16dp"
android:layout_centerHorizontal="true"
android:background="@color/red"
android:text="Hello MotionLayout" />
<Constraint
android:id="
```
0
0
相关推荐
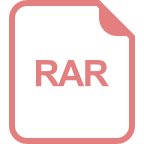





